Preventing Security Vulnerabilities in Java JWT Authentication
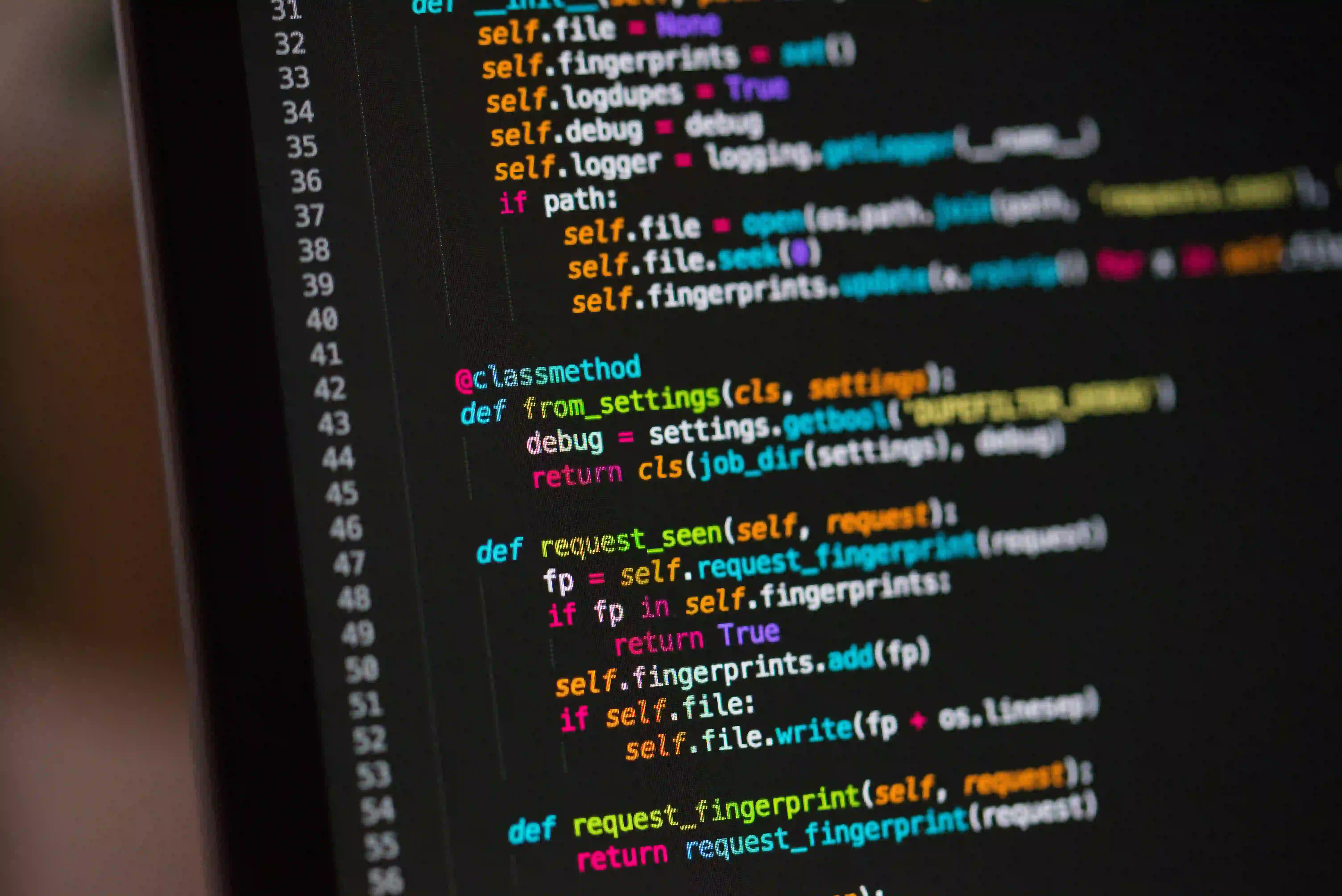
Preventing Security Vulnerabilities in Java JWT Authentication
In today's digital landscape, security is of utmost importance, especially when it comes to handling user authentication. JSON Web Tokens (JWT) have become a popular method for securely transmitting information between parties. However, improper implementation of JWT authentication in Java can lead to security vulnerabilities.
In this article, we will explore best practices for preventing security vulnerabilities in Java JWT authentication. We will discuss common pitfalls and how to mitigate them, as well as provide code examples using Java libraries to illustrate secure implementation.
Understanding the Basics of JWT
Before diving into the security aspects, let’s briefly review what JWT is and how it works. JSON Web Tokens are compact, URL-safe means of representing claims to be transferred between two parties. These tokens are digitally signed, ensuring that the claims contained within them can be trusted.
A JWT typically consists of three parts: the header, the payload, and the signature. The header specifies the type of the token and the signing algorithm used, while the payload contains the claims. The signature is generated by base64url encoding the header and payload, and then applying the specified signature algorithm using a secret key.
Common Security Vulnerabilities in JWT Authentication
1. Insecure Key Storage
One of the most common vulnerabilities in JWT authentication is insecure key storage. If the signing key used to generate JWTs is compromised, attackers can create their own tokens and impersonate legitimate users. Storing the key in a location accessible to unauthorized users, using weak encryption, or hardcoding the key in the source code are all examples of insecure key storage.
2. Lack of Token Expiration
Failure to set an expiration time for JWTs can lead to security risks. Without an expiration, a JWT remains valid indefinitely once it's issued. An attacker who gains access to an active JWT can use it to impersonate the user indefinitely.
3. Insufficient Signature Validation
Improperly validating the JWT signature can lead to security vulnerabilities. If the signature validation process is flawed or can be bypassed, attackers can craft their own JWTs with tampered claims and pass the validation process, leading to unauthorized access.
Mitigating Security Vulnerabilities
1. Secure Key Storage
To mitigate the risk of insecure key storage, always store the signing key in a secure location, such as an environment variable, a secure configuration file, or a key management service. Use strong encryption for the key at rest and in transit. Avoid hardcoding the key in source code or storing it in public repositories.
String jwtSecret = System.getenv("JWT_SECRET");
In this example, we retrieve the JWT secret from an environment variable, ensuring that it remains secure and separate from the application code.
2. Setting Token Expiration
Set a reasonable expiration time for JWTs to limit their lifespan and reduce the window of opportunity for attackers. This can be achieved by including an expiration claim in the JWT payload.
Date expirationDate = new Date(System.currentTimeMillis() + 3600000); // 1 hour from now
By setting the expiration date, JWTs become invalid after a specified period, reducing the risk posed by long-lived tokens.
3. Proper Signature Validation
When validating the JWT signature, it’s crucial to use a strong cryptographic algorithm and verify the integrity of the token. Utilize well-established libraries like JJWT which provides a simple and clear API for creating and validating JWTs.
Jwts.parser().setSigningKey(jwtSecret).parseClaimsJws(token);
In this code snippet, we use the JJWT library to parse and validate the JWT signature using the securely stored jwtSecret
.
The Last Word
In conclusion, implementing secure JWT authentication in Java requires attention to detail and adherence to best practices. By securely storing the signing key, setting token expiration, and properly validating the signature, developers can significantly reduce the risk of security vulnerabilities in JWT authentication.
As cyber threats continue to evolve, staying informed about secure coding practices and leveraging robust libraries is essential in maintaining the integrity and security of authentication systems.
By following these best practices and leveraging reputable libraries, developers can build a more secure and reliable authentication system with Java JWT.