Enhancing REST API Performance With Best Practices
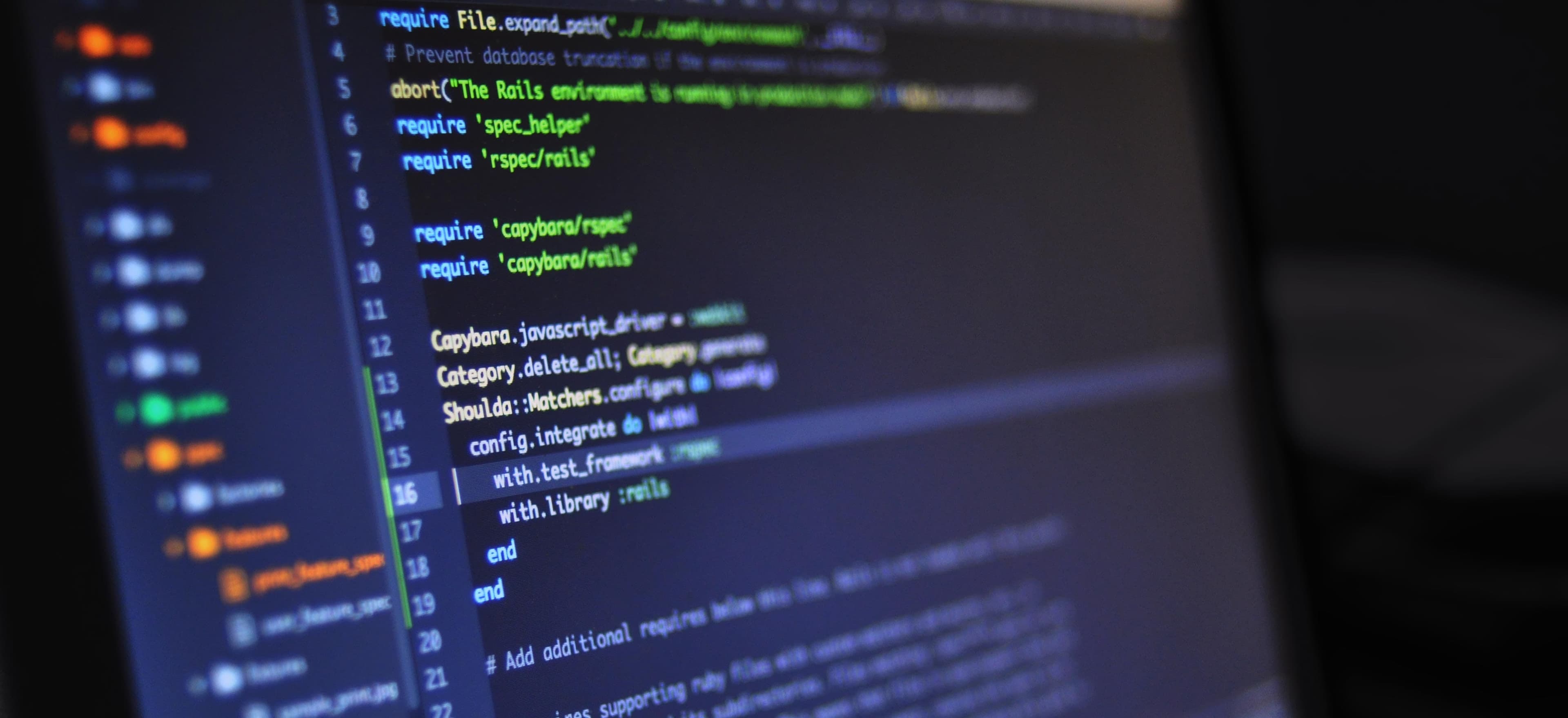
- Published on
Enhancing REST API Performance With Best Practices
In the world of web applications, performance is key. Users expect quick response times and efficient interactions with their favorite apps. When it comes to building REST APIs in Java, there are several best practices and techniques to enhance performance.
In this article, we'll explore some of the most effective strategies for optimizing REST API performance in Java, including asynchronous programming, caching, and payload optimization.
Asynchronous Programming
One of the most effective ways to improve REST API performance is by leveraging asynchronous programming. Traditionally, web applications have been built using synchronous request-response cycles, where each incoming request ties up a thread until a response is returned. This approach can lead to thread exhaustion and poor utilization of resources.
In Java, the introduction of CompletableFuture in Java 8 and enhancements in Java 9 have made asynchronous programming more accessible. By using CompletableFuture and the CompletableFuture API, developers can execute tasks concurrently and compose asynchronous operations more effectively.
Let's take a look at an example of asynchronous processing using CompletableFuture in Java:
import java.util.concurrent.CompletableFuture;
public class AsyncExample {
public static void main(String[] args) {
CompletableFuture.runAsync(() -> {
// Asynchronous task
System.out.println("Running task asynchronously");
});
}
}
In this example, the CompletableFuture.runAsync
method is used to run a task asynchronously. This allows the application to continue processing other tasks while the asynchronous operation is in progress, leading to improved overall performance and resource utilization.
By incorporating asynchronous programming techniques into the design of REST APIs, developers can handle a larger number of concurrent requests with fewer resources, thus enhancing performance significantly.
Caching
Caching is another powerful technique for improving REST API performance. By caching the responses to commonly requested endpoints, subsequent requests for the same data can be served directly from the cache, reducing database or computation overhead.
In Java, the implementation of caching can be achieved through libraries such as Caffeine or caching solutions like Redis. When implementing caching, it's important to consider factors such as cache eviction policies, data expiration, and cache coherency.
Let's consider an example of how caching can be implemented using the Caffeine library in Java:
import com.github.benmanes.caffeine.cache.Caffeine;
import com.github.benmanes.caffeine.cache.Cache;
public class CachingExample {
public static void main(String[] args) {
Cache<String, String> cache = Caffeine.newBuilder().build();
// Populate the cache
cache.put("key1", "value1");
// Retrieve data from the cache
String data = cache.getIfPresent("key1");
}
}
In this example, the Caffeine library is used to create an in-memory cache, and data is stored and retrieved using the put
and getIfPresent
methods, respectively. By incorporating caching into REST API endpoints that fetch static or infrequently changing data, developers can significantly boost performance and reduce response times.
Payload Optimization
Optimizing the payload size of responses is crucial for improving REST API performance, especially in scenarios where the APIs are consumed by mobile devices or low-bandwidth environments. Minimizing the data transferred over the network not only enhances performance but also reduces the load on the client devices.
In Java, the use of libraries such as Jackson for JSON serialization and deserialization provides options for optimizing payload size by including only necessary data in the API responses. Additionally, compressing the response payload using techniques like GZIP can further reduce the size of data transmitted over the network.
Let's consider a simple example of how payload optimization can be achieved using Jackson in Java:
import com.fasterxml.jackson.databind.ObjectMapper;
public class PayloadOptimizationExample {
public static void main(String[] args) throws Exception {
ObjectMapper objectMapper = new ObjectMapper();
// Convert object to JSON string
String jsonString = objectMapper.writeValueAsString(someObject);
// Convert JSON string to object
SomeObject newObj = objectMapper.readValue(jsonString, SomeObject.class);
}
}
In this example, the ObjectMapper class from the Jackson library is used to serialize and deserialize Java objects into JSON. By carefully selecting the fields to include in the JSON response and compressing the payload, developers can significantly enhance the performance of their REST APIs.
Key Takeaways
In conclusion, optimizing REST API performance in Java is essential for delivering high-performance, scalable, and responsive web applications. By incorporating asynchronous programming, caching, and payload optimization techniques, developers can ensure that their REST APIs perform optimally, even under heavy load.
Adopting best practices such as asynchronous programming, caching, and payload optimization can lead to significant improvements in REST API performance, providing a seamless and efficient user experience.
Incorporating these best practices into the design and implementation of REST APIs in Java will not only enhance performance but also contribute to the overall success of web applications in today's competitive digital landscape.
When it comes to optimizing REST API performance, these best practices are just the beginning. To delve deeper into this topic, check out Java's CompletableFuture documentation and Caffeine caching library for further insights.
Remember, the pursuit of excellence in REST API performance is a continuous journey, and embracing these best practices will undoubtedly set you on the path to success.