Troubleshooting Java Performance Issues
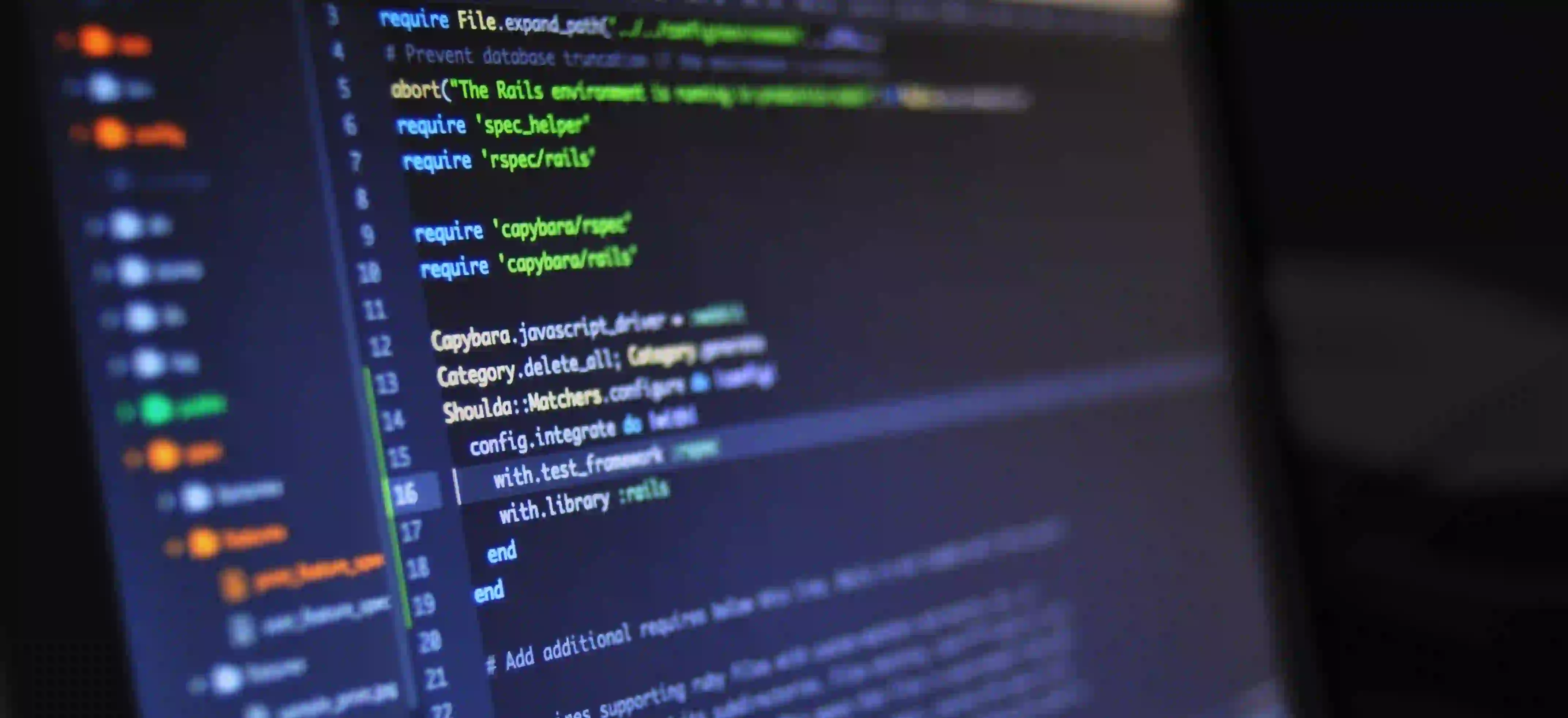
Troubleshooting Java Performance Issues
Java is a versatile and powerful programming language, but like any other language, it can suffer from performance issues. As a Java developer, it's essential to have the skills to troubleshoot and optimize the performance of Java applications. In this guide, we will explore some common performance issues in Java applications and discuss techniques to identify and resolve them.
Performance Profiling
Performance profiling is the first step in troubleshooting Java performance issues. Profiling helps in identifying the performance bottlenecks in the application. There are several tools available for profiling Java applications, such as YourKit, VisualVM, and JProfiler. These tools provide insights into CPU usage, memory usage, garbage collection behavior, and thread behavior.
Using VisualVM for Performance Profiling
VisualVM is a powerful tool that is included with the Java Development Kit (JDK). It provides a visual interface to monitor and profile Java applications. Let's look at an example of how to use VisualVM to profile a simple Java application.
public class PerformanceExample {
public static void main(String[] args) {
for (int i = 0; i < 1000000; i++) {
String s = "Hello" + i;
System.out.println(s);
}
}
}
In this example, we have a simple Java application that concatenates a string in a loop. We can use VisualVM to profile this application and identify any performance issues.
After running the application, we can connect VisualVM to the running Java process and analyze the CPU and memory usage. We may observe high CPU usage or excessive memory consumption, indicating potential performance issues that need to be addressed.
Common Performance Issues
Memory Leaks
Memory leaks occur when an application fails to release memory that is no longer needed. In Java, memory leaks often occur due to improper handling of object references, leading to excessive memory consumption and eventual OutOfMemoryError. Memory profiling tools, such as YourKit and JProfiler, can help identify memory leaks by analyzing object retention and garbage collection behavior.
Inefficient Algorithms
Inefficient algorithms can significantly impact the performance of Java applications. For example, using nested loops with a high computational complexity can lead to poor performance, especially for large input sizes. It's crucial to analyze the algorithmic complexity of critical code segments and refactor them for improved performance.
Excessive Garbage Collection
Frequent garbage collection cycles can introduce significant overhead and degrade the performance of Java applications. This often occurs when objects are created and discarded at a high rate, causing the garbage collector to work overtime. Utilizing tools like VisualVM or Java Mission Control to monitor garbage collection behavior can help in identifying and optimizing excessive garbage collection.
Optimizing Java Performance
Efficient Data Structures and Collections
Choosing the right data structures and collections can greatly impact the performance of Java applications. For example, using HashMap instead of ArrayList for fast lookups or ConcurrentHashMap for thread-safe operations can improve performance. It's essential to understand the characteristics and performance implications of different data structures and utilize them appropriately.
Multithreading and Concurrency
Utilizing multithreading and concurrency can significantly enhance the performance of Java applications, especially for computationally intensive or I/O-bound tasks. However, improper synchronization and contention among threads can lead to performance bottlenecks and thread-related issues. Utilize synchronized blocks, locks, and concurrent data structures effectively to ensure safe and efficient multithreading.
JVM Tuning
The Java Virtual Machine (JVM) provides a plethora of tuning options to optimize the performance of Java applications. Tweaking parameters such as heap size, garbage collection algorithms, and thread stack size can have a substantial impact on application performance. It is essential to understand the implications of JVM tuning and carefully analyze the performance impact of each tuning parameter.
To Wrap Things Up
Troubleshooting and optimizing the performance of Java applications is a crucial aspect of Java development. By leveraging performance profiling tools, identifying common performance issues, and applying optimization techniques, Java developers can significantly enhance the performance and scalability of their applications. Continuous monitoring and performance tuning are essential for maintaining optimal performance as the application evolves.
In conclusion, mastering the art of troubleshooting and optimizing Java performance empowers developers to build high-performing and efficient Java applications that meet the demands of modern software development.
Remember, a performant Java application is not just a feature, but a reflection of skill and dedication to the craft of software development. Good luck on your journey to Java performance optimization excellence!
The beauty of Java lies in its versatility, but ensuring its performance is equally crucial. Understanding the intricacies of performance issues and proficiently handling them can elevate your Java development prowess. Let's craft exceptional Java applications that not only meet expectations but exceed them through optimal performance!