Resolving ClassNotFoundException in Java
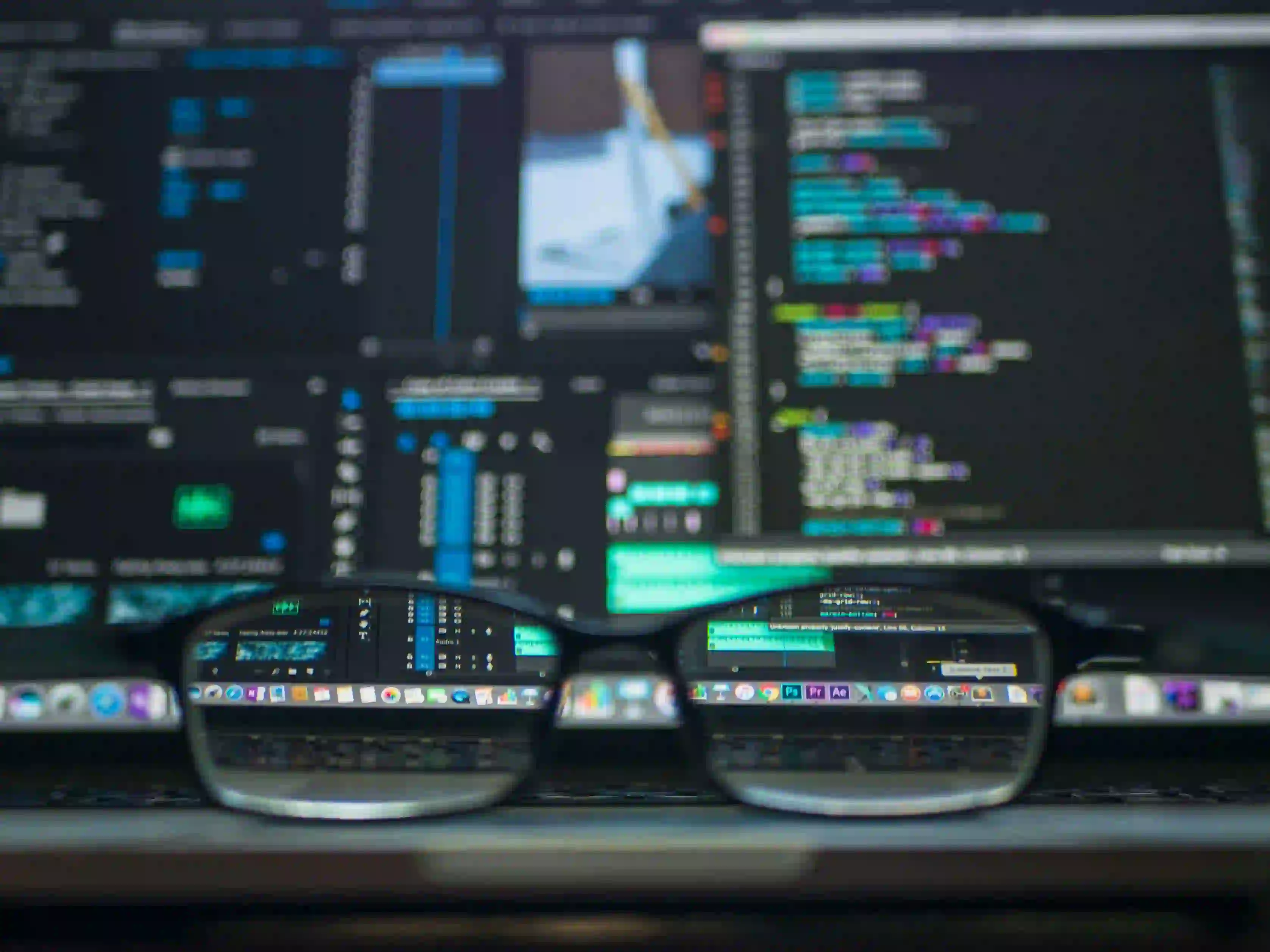
Resolving ClassNotFoundException in Java
When working with Java, it's not uncommon to encounter the dreaded ClassNotFoundException
. This exception occurs when the Java Virtual Machine (JVM) tries to load a particular class but fails to find it in the classpath. In this blog post, we will delve into the reasons behind this error and explore various strategies to resolve it.
Understanding ClassNotFoundException
The ClassNotFoundException
is a checked exception that occurs at runtime when a program attempts to load a class using the Class.forName()
method or by accessing a class through the classloader, but the class with the specified name cannot be found.
try {
Class<?> myClass = Class.forName("com.example.MyClass");
} catch (ClassNotFoundException e) {
// Handle the exception
}
The reasons for this exception can vary, but they usually boil down to the following:
-
Incorrect Classpath Configuration: The class in question is not available in the classpath specified for the application.
-
Missing Dependency: The required JAR file or dependency containing the class is not included in the classpath.
-
Dynamic Class Loading: Dynamically loading classes without the proper handling of ClassNotFoundException.
Resolving ClassNotFoundException
Now, let's explore some effective strategies to troubleshoot and resolve ClassNotFoundException
errors.
1. Verify the Classpath Configuration
The first step is to ensure that the classpath is correctly configured. Verify that the class or JAR file containing the class is included in the classpath. When executing a Java program, the classpath can be specified using the -cp
or -classpath
option.
java -cp /path/to/your/class com.example.MainClass
2. Check for Missing Dependencies
If the class is part of an external library or dependency, ensure that the corresponding JAR file is included in the classpath. Using a build tool such as Maven or Gradle can help manage dependencies and ensure they are included in the classpath during compilation and execution.
3. Dynamic Class Loading and Exception Handling
When using dynamic class loading mechanisms, it's crucial to handle ClassNotFoundException
appropriately. Always wrap dynamic class loading code within a try-catch block to handle the exception gracefully.
try {
Class<?> myClass = Class.forName("com.example.MyClass");
} catch (ClassNotFoundException e) {
// Log the error or take necessary action
e.printStackTrace();
}
4. Classloaders and Classpath Exploration
Understanding how classloaders work and how they search for classes can provide valuable insights into resolving ClassNotFoundException
. Sometimes, the issue may stem from conflicting classloaders or an incorrect class loading strategy.
5. Package Structure and Naming Conventions
Ensure that the package structure and naming conventions align with the actual filesystem structure. The package names specified in the Java source files should match the directory structure.
6. IDE-Specific Configurations
If the ClassNotFoundException
occurs during development within an IDE such as Eclipse or IntelliJ IDEA, check the project settings and build configurations to ensure that all necessary libraries and dependencies are included.
Final Thoughts
In conclusion, the ClassNotFoundException
in Java can be a frustrating error to encounter. However, armed with a solid understanding of the classpath, dynamic class loading, and effective troubleshooting strategies, you can confidently diagnose and resolve this issue. Always remember to verify the classpath, manage dependencies diligently, handle exceptions properly, and familiarize yourself with classloading mechanisms to effectively tackle ClassNotFoundException
.
By following the strategies outlined in this post, you can ensure that your Java applications load classes seamlessly without encountering the dreaded ClassNotFoundException
error.
Remember, adeptly navigating the intricacies of class loading and classpath configuration is a hallmark of a proficient Java developer. Happy coding!
Do you have any experiences dealing with ClassNotFoundException
in Java? Share your experiences and insights in the comments below.
For further reading, check out the Java Documentation on Class.forName and Understanding Java Classloaders.
Happy coding!