Maximizing Performance with Kotlin Tail Recursion
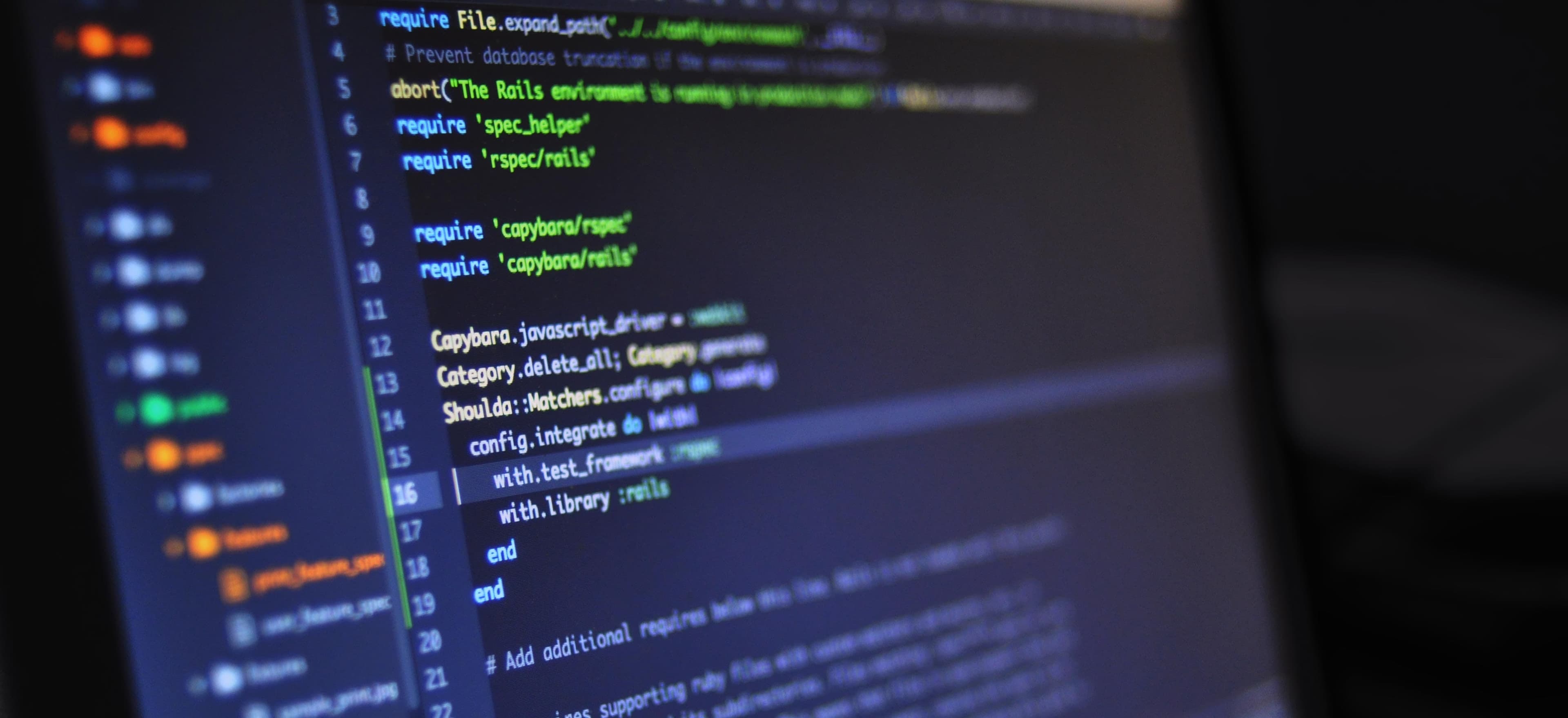
- Published on
Maximizing Performance with Kotlin Tail Recursion
When it comes to optimizing the performance of your Kotlin applications, leveraging tail recursion can significantly improve efficiency and reduce the risk of stack overflow errors. In this blog post, we will delve into the concept of tail recursion, explore its benefits, and demonstrate its implementation with Kotlin.
Understanding Tail Recursion
In traditional recursion, a function calls itself to solve subproblems. However, if the recursive call is not the last operation in the function, the call stack continues to grow with each recursive call, potentially leading to a stack overflow when dealing with large inputs.
On the other hand, tail recursion occurs when the recursive call is the last operation in the function. This crucial distinction allows compilers to optimize tail-recursive functions by reusing the current stack frame for the next iteration, effectively eliminating the risk of stack overflow.
The Benefits of Tail Recursion
Tail recursion optimization offers several advantages:
-
Improved Performance: By reusing the stack frame, tail-recursive functions avoid unnecessary stack growth, leading to more efficient memory utilization and faster execution.
-
Prevention of Stack Overflow: Since tail recursion eliminates stack growth, it mitigates the risk of encountering stack overflow errors, enabling the processing of larger inputs without failure.
-
Elegance and Readability: Tail-recursive functions often exhibit a clear, iterative structure, enhancing code readability and maintainability.
Having grasped the concept and advantages of tail recursion, let’s now explore its practical implementation in Kotlin.
Implementing Tail Recursion in Kotlin
Consider the classic example of computing the factorial of a non-negative integer using traditional recursion. The following Kotlin function illustrates this scenario:
fun factorial(n: Int): Int {
return if (n == 0) 1 else n * factorial(n - 1)
}
While elegant, this straightforward implementation is not tail-recursive, as the recursive call is not the last operation due to the multiplication operation.
To transform this function into a tail-recursive version, we can leverage an accumulator parameter to store intermediate results. This approach allows us to eliminate the multiplication operation after the recursion, facilitating tail call optimization:
tailrec fun factorialTR(n: Int, accumulator: Int = 1): Int {
return if (n == 0) accumulator else factorialTR(n - 1, n * accumulator)
}
In this tail-recursive version, the accumulator
parameter accumulates the intermediate results as the function iterates through the recursive calls, making it eligible for tail call optimization.
Leveraging Tail Recursion for Performance
To appreciate the impact of tail recursion on performance, let's compare the traditional recursive factorial function with its tail-recursive counterpart. We can measure the execution time for both approaches using the following benchmarking code:
fun main() {
val n = 20
val start = System.currentTimeMillis()
println(factorial(n))
val end = System.currentTimeMillis()
println("Factorial elapsed time: ${end - start} milliseconds")
val startTR = System.currentTimeMillis()
println(factorialTR(n))
val endTR = System.currentTimeMillis()
println("FactorialTR elapsed time: ${endTR - startTR} milliseconds")
}
By benchmarking the two factorial functions, we can observe the performance improvements achieved through tail recursion. This not only demonstrates the efficiency gains but also underscores the importance of tail recursion in optimizing recursive algorithms.
Considerations and Limitations
While tail recursion offers compelling performance benefits, it's essential to recognize its limitations.
-
Compiler Support: Tail call optimization is subject to the capabilities of the compiler. Although Kotlin provides support for tail recursion optimization, not all recursive functions may be eligible for optimization, depending on the specific compiler and its configuration.
-
Accumulator Complexity: Adapting a function for tail recursion optimization may introduce the need for additional accumulator parameters, potentially increasing the complexity of the function signature.
-
Algorithm Suitability: Not all recursive algorithms can be easily adapted for tail recursion. Complex algorithms with multiple recursive calls or non-linear control flow may not lend themselves to straightforward tail call optimization.
Considering these factors, it's crucial to weigh the benefits and implications of tail recursion within the context of your specific use case.
Final Considerations
In conclusion, leveraging tail recursion in Kotlin empowers developers to enhance the performance and robustness of recursive algorithms. By harnessing this optimization technique, developers can mitigate the risks of stack overflow errors, improve memory efficiency, and refine the readability of their code.
As you continue to explore Kotlin and refine your programming skills, incorporating tail recursion into your algorithmic toolkit can undoubtedly elevate the efficiency and elegance of your code.
With its powerful combination of simplicity and performance, tail recursion stands as a valuable tool in the arsenal of any Kotlin developer aiming to optimize their applications.
Discover more about Kotlin and tail recursion:
- Kotlin Official Documentation
- Tail Recursion in Kotlin
- Understanding Tail Recursion in Depth
- Exploring Tail Recursion Optimization
Happy coding with Kotlin and tail recursion!