Handling Common Exceptions in Python
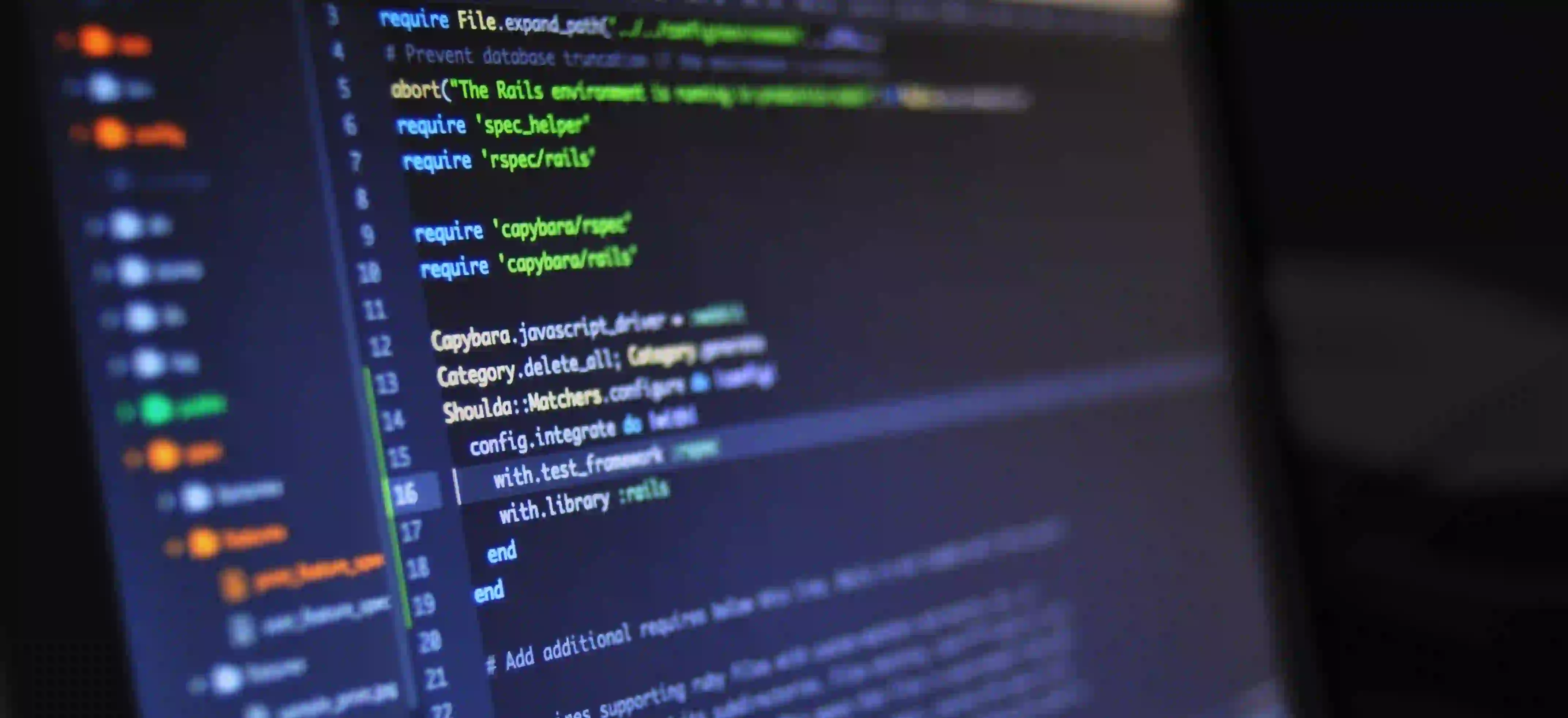
The Importance of Exception Handling in Java
When writing Java code, it's crucial to anticipate and handle exceptions. An exception is an event that occurs during the execution of a program that disrupts the normal flow of instructions. Failing to address exceptions can lead to unexpected program termination and potentially harmful consequences. In this blog post, we'll explore the significance of exception handling in Java and discuss common exceptions and their resolutions.
Understanding Exceptions in Java
In Java, exceptions are objects that represent an abnormal condition or error. When a method encounters an exceptional situation, it throws an exception. If the exception is not handled, it propagates up the call stack to the calling method, continuing until it is either caught or the program terminates.
Types of Exceptions
Java exceptions are categorized into two main types: checked exceptions and unchecked exceptions. Checked exceptions must be either caught or declared in the method's signature using the throws
keyword. Unchecked exceptions, on the other hand, do not require handling.
Checked Exceptions
Checked exceptions are typically external to the Java program and are not caused by programming errors. Examples include IOException
, SQLException
, and ClassNotFoundException
. These exceptions are checked at compile time, ensuring that they are either caught or declared to be thrown.
Unchecked Exceptions
Unchecked exceptions, also known as runtime exceptions, are usually caused by programming errors such as logical and runtime errors. Examples include NullPointerException
, ArrayIndexOutOfBoundsException
, and ArithmeticException
. Unlike checked exceptions, unchecked exceptions do not need to be explicitly caught or declared.
Handling Exceptions
Java provides a mechanism for handling exceptions using the try-catch-finally
block. The try
block encloses the code that may throw an exception, while the catch
block handles the exception if it occurs. The finally
block contains code that will always execute, regardless of whether an exception is thrown.
try {
// Code that may throw an exception
} catch (ExceptionType1 e1) {
// Handle ExceptionType1
} catch (ExceptionType2 e2) {
// Handle ExceptionType2
} finally {
// Code to be executed regardless of whether an exception is thrown
}
Example:
Let's consider an example where we attempt to read from a file using Java's FileInputStream
and handle a potential FileNotFoundException
.
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
public class FileReadExample {
public static void main(String[] args) {
FileInputStream fis = null;
try {
fis = new FileInputStream("file.txt");
// Perform file reading operations
} catch (FileNotFoundException e) {
System.out.println("File not found: " + e.getMessage());
} finally {
try {
if (fis != null) {
fis.close();
}
} catch (IOException e) {
System.out.println("Error while closing the file: " + e.getMessage());
}
}
}
}
In the above example, the try
block attempts to create a FileInputStream
for a file named "file.txt". If the file is not found, a FileNotFoundException
is thrown and caught in the catch
block, displaying an error message. The finally
block ensures that the file stream is closed, handling any potential IOException
.
Best Practices for Exception Handling
While handling exceptions in Java, it's essential to follow best practices to ensure robust and maintainable code:
-
Catch Specific Exceptions: Catch specific exceptions rather than using a generic
catch
block forException
. This allows for more targeted handling based on the type of exception. -
Use
finally
for Cleanup: Use thefinally
block to perform cleanup operations such as closing streams or releasing resources, ensuring that critical tasks are executed regardless of whether an exception occurs. -
Throw Custom Exceptions: Define and throw custom exceptions when a specific error condition is encountered, providing meaningful information about the exceptional situation.
-
Logging Exceptions: Log exceptions using a logging framework like Log4j or java.util.logging to record the details of exceptions for debugging and monitoring purposes.
Bringing It All Together
Exception handling is an integral aspect of Java programming, enabling developers to anticipate and manage unexpected situations effectively. By understanding the types of exceptions, implementing proper exception handling mechanisms, and adhering to best practices, Java developers can write resilient and reliable code.
In this post, we've explored the significance of exception handling in Java, discussed common types of exceptions, provided examples of handling exceptions, and highlighted best practices for robust exception management. Incorporating these principles into your Java development endeavors will contribute to the creation of more stable and maintainable applications.
For additional insights into Java exception handling, consider exploring Oracle's official documentation on exceptions and Baeldung's comprehensive guide to exception handling in Java.