Troubleshooting Java Puzzlers for OCA: Part 4
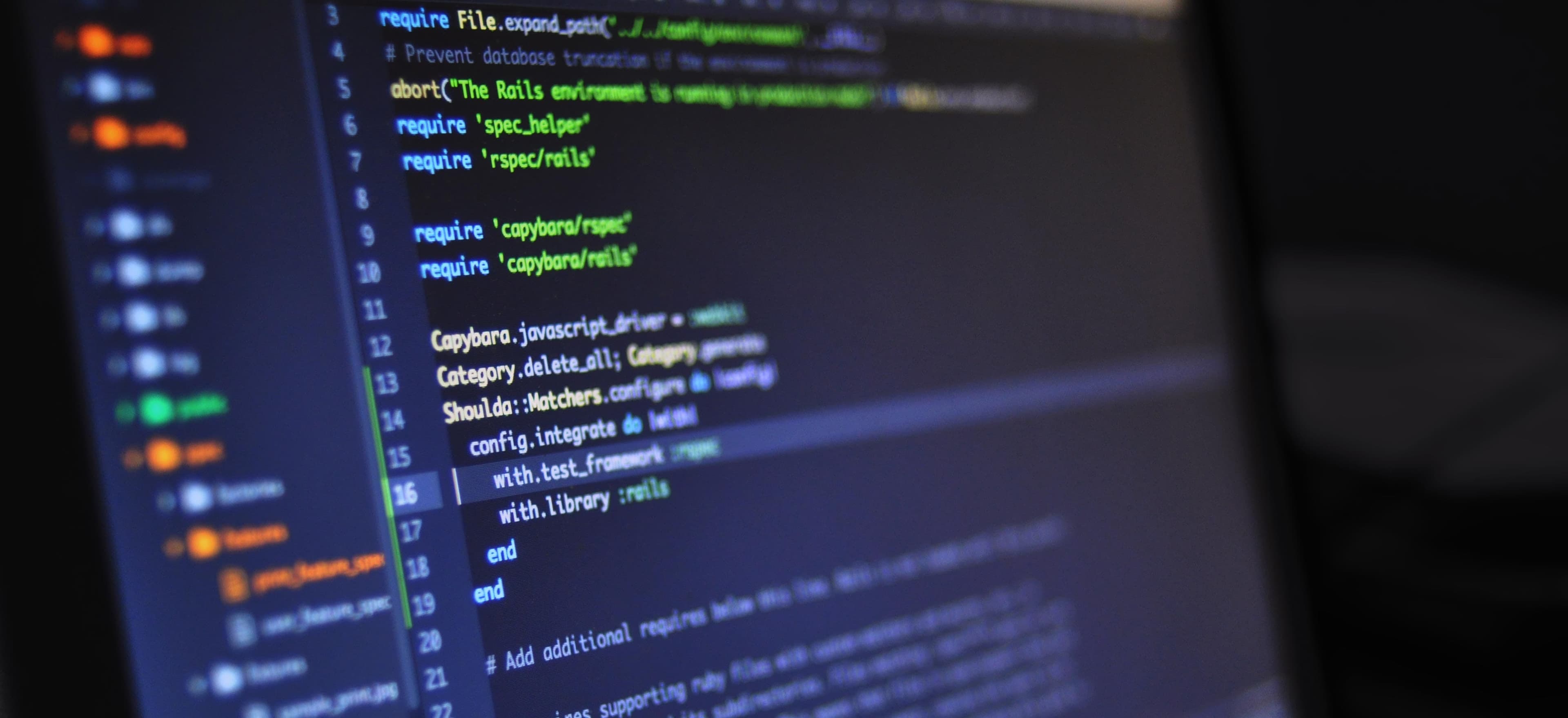
- Published on
Troubleshooting Java Puzzlers for OCA: Part 4
Welcome to the fourth installment of our OCA (Oracle Certified Associate) Java certification puzzlers series! In this post, we'll explore some common Java puzzlers that can help you sharpen your Java programming skills and prepare for the OCA certification exam. This series aims to tackle tricky Java concepts with real-world examples to help you understand Java in a deeper and more practical way.
Let's dive into the world of Java puzzlers and unravel some perplexing code snippets to better understand the intricacies of the Java programming language.
Puzzler 1: The NullPointerException Trap
Consider the following Java code snippet:
public class NullPointerExceptionPuzzler {
public static void main(String[] args) {
String text = getText();
System.out.println(text.length());
}
private static String getText() {
return null;
}
}
What do you think will happen when you run this code? Will it compile successfully? Will it throw a NullPointerException
at runtime? Take a moment to think about it.
Surprisingly, this code snippet will compile successfully. However, when you run the program, it will throw a NullPointerException
at the statement System.out.println(text.length())
.
The reason behind this behavior is that the getText()
method returns null
, and invoking the length()
method on a null
String reference leads to a NullPointerException
. To fix this, you can add a null check before invoking the length()
method, like so:
public class NullPointerExceptionPuzzler {
public static void main(String[] args) {
String text = getText();
if (text != null) {
System.out.println(text.length());
} else {
System.out.println("Text is null");
}
}
private static String getText() {
return null;
}
}
Always remember to perform a null check when invoking methods on potentially null references to avoid unexpected NullPointerException
errors.
Puzzler 2: The Infamous Floating-Point Trap
Let's explore a puzzler related to floating-point arithmetic in Java:
public class FloatingPointPuzzler {
public static void main(String[] args) {
double price1 = 1.03;
double price2 = 0.42;
double sum = price1 - price2;
System.out.println("Sum: " + sum);
}
}
What do you think will be the output of this program? Will it display the expected result of 0.61
?
Surprisingly, the output of this program will be 0.6100000000000001
instead of the expected 0.61
. This unexpected behavior is due to the inherent nature of floating-point arithmetic in Java, which can lead to precision errors.
To accurately handle such scenarios, it's recommended to use BigDecimal
for precise decimal arithmetic operations. Here's how you can refactor the code using BigDecimal
:
import java.math.BigDecimal;
public class FloatingPointPuzzler {
public static void main(String[] args) {
BigDecimal price1 = new BigDecimal("1.03");
BigDecimal price2 = new BigDecimal("0.42");
BigDecimal sum = price1.subtract(price2);
System.out.println("Sum: " + sum);
}
}
By using BigDecimal
, you can ensure precise and accurate arithmetic operations, especially when dealing with monetary calculations or other scenarios that require exact decimal representations.
The Closing Argument
In this part of our Java puzzlers series, we've examined puzzlers related to NullPointerException
and floating-point arithmetic in Java. It's crucial to understand these nuances to write robust and error-free Java code.
Stay tuned for the next installment, where we'll unravel more Java puzzlers to enhance your Java programming skills and prepare you for the OCA certification exam.
Remember, practice makes perfect, so keep coding and exploring the fascinating world of Java!
Make sure to check out our OCA certification guide to further your understanding of Java and prepare for the OCA exam.
Happy coding!