Optimizing Performance with Functional Java Collections
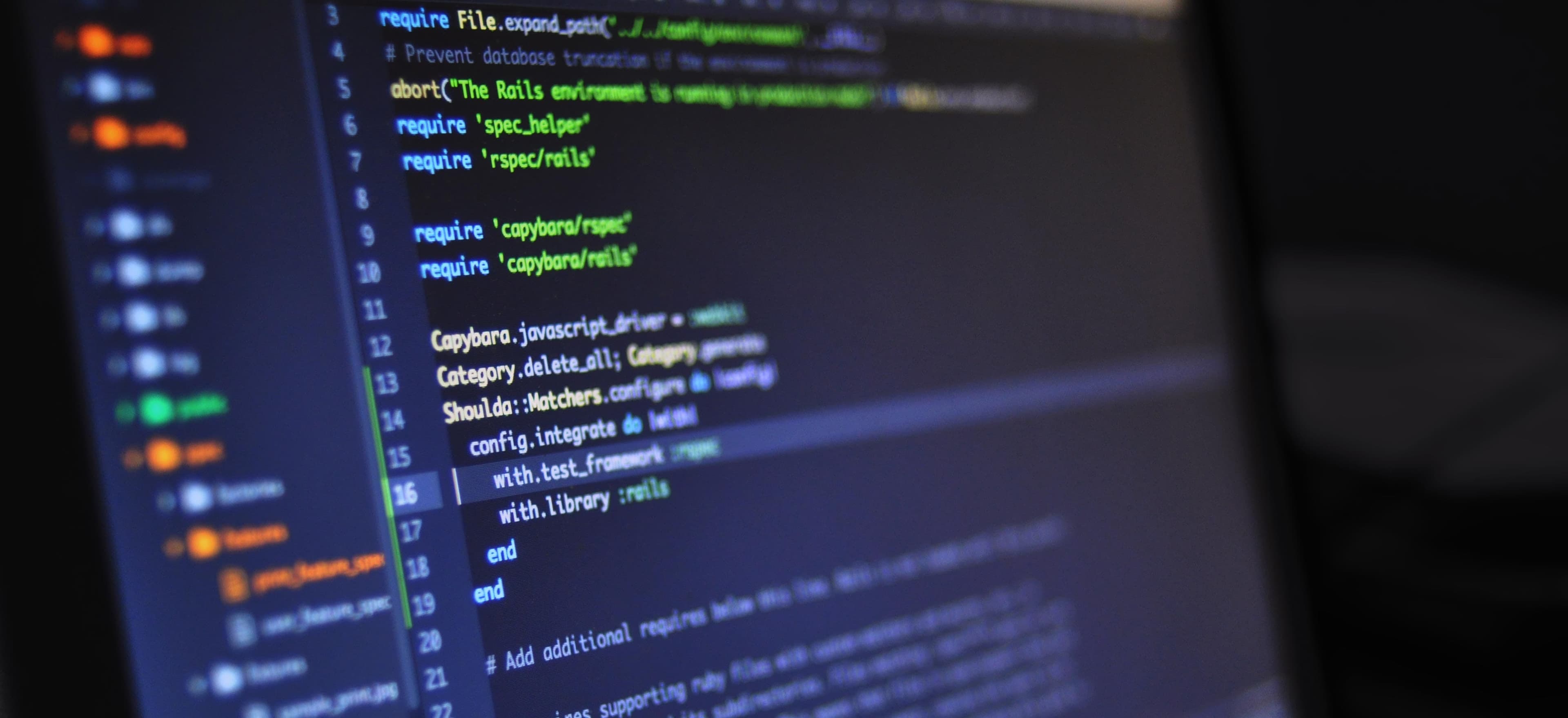
- Published on
Optimizing Performance with Functional Java Collections
When it comes to working with collections in Java, performance is always a key consideration. In recent years, functional programming has gained popularity for its expressive and concise syntax. Java has also embraced this paradigm, offering functional features that can help optimize the performance of working with collections.
In this article, we'll explore how functional programming can be used to optimize performance when working with Java collections. We'll cover key concepts such as immutability, lazy evaluation, and parallel processing, and demonstrate how these concepts can be leveraged to achieve better performance.
Immutability and Functional Collections
Immutability is a cornerstone of functional programming and it brings significant performance benefits when working with collections. In Java, the java.util.Collections
class provides static factory methods for creating immutable collections. Immutability ensures that a collection cannot be changed after it has been created, which allows for more predictable behavior and enables certain optimizations.
Let's consider an example of creating an immutable list using Collections.unmodifiableList
:
List<String> mutableList = new ArrayList<>();
mutableList.add("apple");
mutableList.add("banana");
mutableList.add("cherry");
List<String> immutableList = Collections.unmodifiableList(mutableList);
In this example, the immutableList
is created from the mutableList
. Any attempt to modify immutableList
will result in an UnsupportedOperationException
. This immutability ensures that the collection remains unchanged, which can lead to performance benefits, especially in multithreaded scenarios.
Lazy Evaluation and Streams
Java 8 introduced the Stream
API, which provides a powerful way to work with collections in a functional style. One key advantage of streams is their support for lazy evaluation, which means that operations on the elements of a stream are deferred until a terminal operation is invoked.
Consider the following example of using a stream to filter and collect elements:
List<String> fruits = Arrays.asList("apple", "banana", "cherry", "date");
List<String> filteredFruits = fruits.stream()
.filter(fruit -> fruit.startsWith("a"))
.collect(Collectors.toList());
In this example, the filter
operation is applied lazily to each element of the stream, and the collect
operation triggers the processing and collection of the filtered elements. Lazy evaluation can lead to improved performance, especially when dealing with large collections, as it avoids unnecessary intermediate operations.
Parallel Processing with Streams
Another performance optimization that comes with using streams is the ability to perform operations in parallel. The Stream
API provides the parallel
method, which allows operations on a stream to be executed concurrently.
Let's see how parallel processing can be used to improve performance when processing a large collection of numbers:
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 10);
int sum = numbers.parallelStream()
.filter(number -> number % 2 == 0)
.mapToInt(number -> number * 2)
.sum();
In this example, the parallelStream
method enables parallel processing of the elements in the stream. This can result in performance improvements, especially when working with computationally intensive operations or when processing large datasets.
Using Immutable Collections and Streams in Practice
To demonstrate the performance benefits of using immutable collections and streams in Java, let's consider a scenario where we need to process a large collection of data. We'll compare the performance of mutable collections and traditional iteration with immutable collections and streams.
Scenario
We have a list of employee objects, and we need to calculate the average age of employees whose salary is above a certain threshold.
Approach 1: Using Mutable Collections and Traditional Iteration
List<Employee> employees = // populate the list of employees
double totalAge = 0;
int count = 0;
for(Employee employee : employees) {
if(employee.getSalary() > threshold) {
totalAge += employee.getAge();
count++;
}
}
double averageAge = totalAge / count;
Approach 2: Using Immutable Collections and Streams
List<Employee> employees = // populate the list of employees
double averageAge = employees.stream()
.filter(employee -> employee.getSalary() > threshold)
.mapToInt(Employee::getAge)
.average()
.orElse(0.0);
Performance Comparison
When we compare the performance of these two approaches, especially with a large dataset, we can often see significant performance improvements when using immutable collections and streams. The lazy evaluation and potential for parallel processing offered by streams can lead to better performance, especially for computationally intensive operations.
The Closing Argument
In conclusion, functional programming features in Java, such as immutability, lazy evaluation, and parallel processing provided by streams, offer powerful ways to optimize the performance of working with collections. By leveraging these features, developers can write more expressive and concise code while achieving better performance.
When working with collections in Java, it's important to consider the performance implications of different approaches and to leverage the functional features provided by the language to optimize performance.
By embracing immutability, lazy evaluation, and parallel processing, developers can maximize the performance of their applications when working with collections, ultimately leading to more efficient and scalable solutions.
For further reading on the topic, I recommend checking out Oracle's official documentation on Java Collections and Baeldung's guide to Java Streams for a deeper understanding of these concepts.
I hope this article has provided valuable insights into how functional programming can be used to optimize performance when working with Java collections. Happy coding!
Checkout our other articles