Integrating Solr with Maven for Effective Search Solution
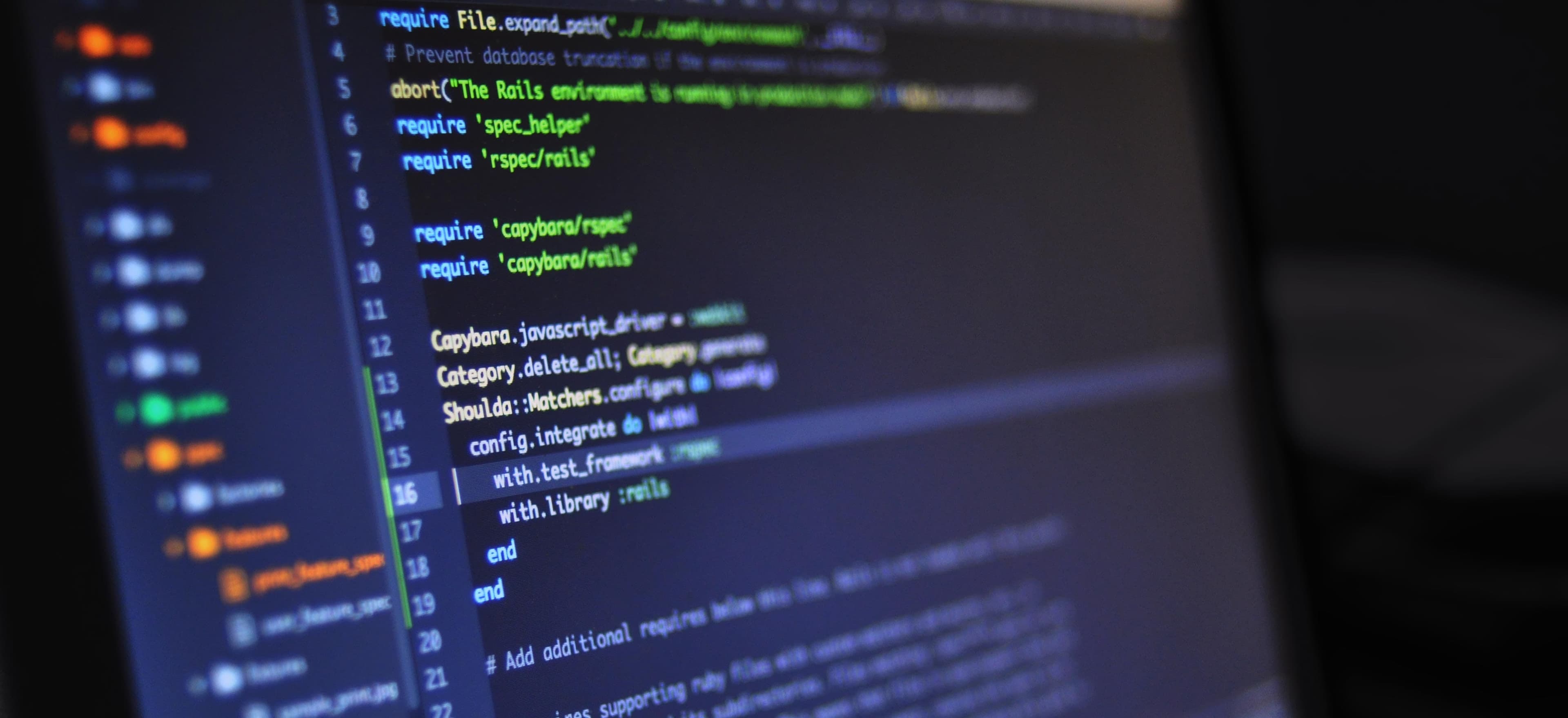
- Published on
Integrating Solr with Maven for Effective Search Solution
In today's fast-paced world, having an effective search solution in your Java application is crucial for delivering a seamless user experience. Apache Solr, a popular open-source search platform built on Apache Lucene, provides powerful full-text search, hit highlighting, faceted search, dynamic clustering, and much more. Integrating Solr with Maven, a widely-used build automation tool primarily used for Java projects, allows for streamlined project management and dependency resolution. In this article, we'll walk through the process of integrating Solr with Maven to create a robust search solution for your Java application.
Step 1: Setting Up the Solr Server
Before we dive into Maven integration, we need to have a Solr server up and running. You can download the latest version of Solr from the official Apache Solr website or use tools like Apache Solr Docker to set up a local instance for development.
Once the Solr server is running, make sure to create a new core for your project using the Solr Admin UI or the Solr Core API. The core represents a single index, along with its configuration and schema.
Step 2: Maven Project Setup
Assuming you already have a Maven-based Java project, you can start integrating Solr by adding the SolrJ dependency to your project's pom.xml
file. SolrJ is the official Java client for interacting with Solr.
<dependency>
<groupId>org.apache.solr</groupId>
<artifactId>solr-solrj</artifactId>
<version>8.11.1</version> <!-- Replace with the latest version -->
</dependency>
Step 3: Creating a Solr Service
Next, create a Solr service class to encapsulate the interactions with the Solr server. This class will handle indexing documents, querying, and other Solr operations. Let's start by creating a basic skeleton for the Solr service.
import org.apache.solr.client.solrj.SolrClient;
import org.apache.solr.client.solrj.impl.HttpSolrClient;
public class SolrService {
private SolrClient solrClient;
public SolrService(String solrUrl) {
this.solrClient = new HttpSolrClient.Builder(solrUrl).build();
}
// Add methods for indexing, querying, and other operations
}
In the above code, we're using the HttpSolrClient
to connect to the Solr server. The solrUrl
parameter will be the URL of your Solr server, such as http://localhost:8983/solr/core1
. You should replace core1
with the name of the core you created earlier.
Step 4: Indexing Documents
Indexing documents is a fundamental aspect of utilizing Solr for search. Let's create a method in the SolrService
class to index a document.
import org.apache.solr.common.SolrInputDocument;
import org.apache.solr.client.solrj.SolrServerException;
import java.io.IOException;
public void indexDocument(String id, String title, String content) {
SolrInputDocument document = new SolrInputDocument();
document.addField("id", id);
document.addField("title", title);
document.addField("content", content);
try {
solrClient.add(document);
solrClient.commit();
} catch (SolrServerException | IOException e) {
// Handle exceptions
}
}
In the indexDocument
method, we create a SolrInputDocument
and add fields such as id
, title
, and content
. After adding the document, we commit the changes to make them visible for searching.
Step 5: Querying Solr
Now, let's add a method to perform a basic query to retrieve documents from Solr.
import org.apache.solr.client.solrj.SolrQuery;
import org.apache.solr.client.solrj.response.QueryResponse;
public QueryResponse search(String query) {
SolrQuery solrQuery = new SolrQuery();
solrQuery.setQuery(query);
try {
return solrClient.query(solrQuery);
} catch (SolrServerException | IOException e) {
// Handle exceptions
return null;
}
}
The search
method takes a query
as input, creates a SolrQuery
object, sets the query, and executes it against the Solr server. The returned QueryResponse
contains the search results.
Step 6: Using the Solr Service in Your Application
With the Solr service in place, you can now use it in your Java application to index documents and perform searches.
public class Main {
public static void main(String[] args) {
SolrService solrService = new SolrService("http://localhost:8983/solr/core1");
// Index document
solrService.indexDocument("1", "Sample Document", "This is a sample document for Solr indexing");
// Perform a search
QueryResponse response = solrService.search("sample");
// Process the search results
// ...
}
}
In Conclusion, Here is What Matters
Integrating Solr with Maven allows for a seamless search solution as part of your Java application. By following the steps outlined in this article, you can effectively leverage Solr's capabilities for full-text search, indexing, and more while benefiting from Maven's project management features. With this integration, you are well-equipped to deliver a powerful and efficient search experience to your users.
In conclusion, integrating Solr with Maven offers a robust and scalable search solution for Java applications. By leveraging the strength of Solr's search capabilities and Maven's project management, you can enhance the search experience for your users in a streamlined and efficient manner. Start integrating Solr with Maven today and take your search functionality to the next level!