Optimizing Database Insertion Through JSON Parsing
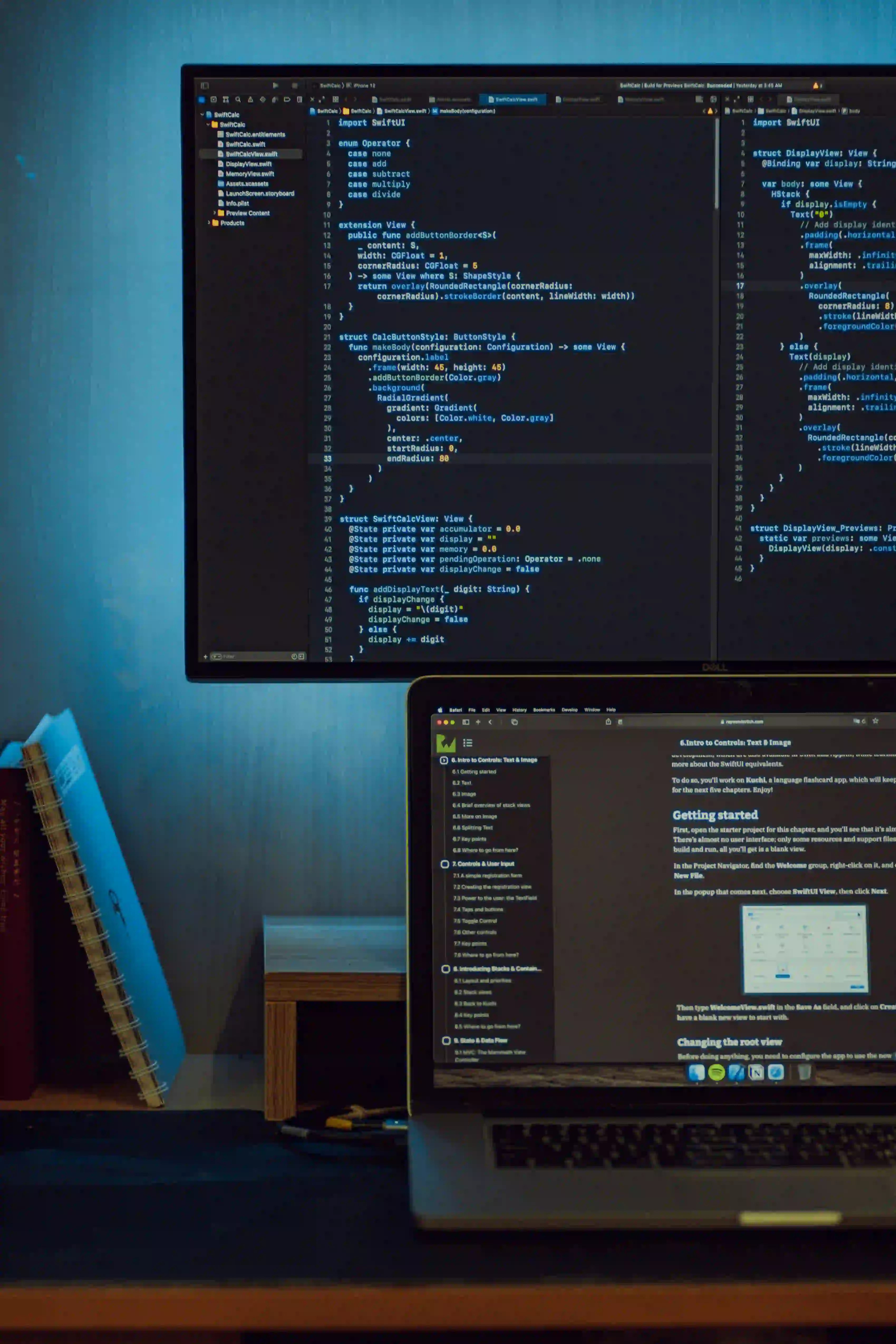
In today's blog post, we will discuss how to optimize database insertion processes in Java by leveraging JSON parsing. As data storage and retrieval play crucial roles in modern software development, it's important to ensure that these operations are as efficient as possible. We'll explore how JSON parsing can streamline the insertion of data into a database, leading to improved performance and a more seamless user experience.
Understanding the Problem
When dealing with database insertion in Java, one common scenario involves the need to transform JSON data into database records. This process often entails parsing JSON objects and mapping their properties to database columns, which can be both time-consuming and error-prone. Inefficient parsing and mapping can lead to performance bottlenecks, especially when dealing with large datasets.
Leveraging JSON Parsing for Database Insertion
To address these challenges, we can utilize JSON parsing libraries in Java to streamline the data insertion process. Libraries such as Gson and Jackson provide convenient tools for parsing JSON data and mapping it to Java objects, allowing for easy integration with database operations.
Using Gson for JSON Parsing
Gson, a popular JSON parsing library developed by Google, offers a straightforward way to parse JSON data into Java objects. Let's consider an example where we have a JSON object representing a user, and we want to insert this data into a database.
// Sample JSON data representing a user
String json = "{\"id\": 1, \"name\": \"John Doe\", \"email\": \"john@example.com\"}";
// Parse JSON data into a Java object using Gson
Gson gson = new Gson();
User user = gson.fromJson(json, User.class);
In this example, we parse the JSON data into a User
object using Gson's fromJson
method. This allows us to easily work with the parsed data as a Java object, facilitating the subsequent database insertion process.
Mapping Parsed Data to Database Columns
Once we have the JSON data parsed into Java objects, we can map their properties to database columns for insertion. Let's take a look at how we can map the User
object to a database insertion query using JDBC.
// Assuming a database connection is established
PreparedStatement statement = connection.prepareStatement("INSERT INTO users (id, name, email) VALUES (?, ?, ?)");
statement.setInt(1, user.getId());
statement.setString(2, user.getName());
statement.setString(3, user.getEmail());
statement.executeUpdate();
In this JDBC example, we map the properties of the User
object to the corresponding columns in the users
table for insertion. By leveraging the parsed Java object, we simplify the process of populating the database with the JSON data.
Why JSON Parsing Optimizes Database Insertion
Simplified Data Transformation
JSON parsing libraries eliminate the need for manual parsing and transformation of JSON data into Java objects. This streamlines the process and reduces the likelihood of errors, resulting in more efficient database insertion.
Improved Data Integrity
By using parsed Java objects, we leverage the type safety and validation provided by the Java language, ensuring that the data being inserted into the database adheres to the expected data types and constraints.
Enhanced Readability and Maintainability
The use of JSON parsing libraries improves the readability and maintainability of the codebase by providing a clear and concise way to handle JSON data. This makes the insertion logic more accessible to developers and easier to maintain over time.
Wrapping Up
In this blog post, we've explored how JSON parsing can optimize the database insertion process in Java. By leveraging libraries such as Gson and Jackson, we can streamline the parsing of JSON data and its subsequent insertion into a database. This approach simplifies data transformation, enhances data integrity, and improves the readability and maintainability of the codebase.
Optimizing database insertion through JSON parsing ultimately leads to better performance and a more robust data storage solution. As software developers, it's important to continually seek out ways to enhance the efficiency and reliability of data operations, and leveraging JSON parsing is a valuable strategy in this pursuit.
We hope this post has provided valuable insights into optimizing database insertion in Java and encourages you to explore the benefits of JSON parsing in your own projects.
To further your understanding of JSON parsing and database operations in Java, consider exploring the official documentation for Gson and Jackson. These resources offer comprehensive insights into the capabilities and usage of these libraries.
Thank you for reading, and happy coding!