Improving Web Scraping Efficiency and Accuracy
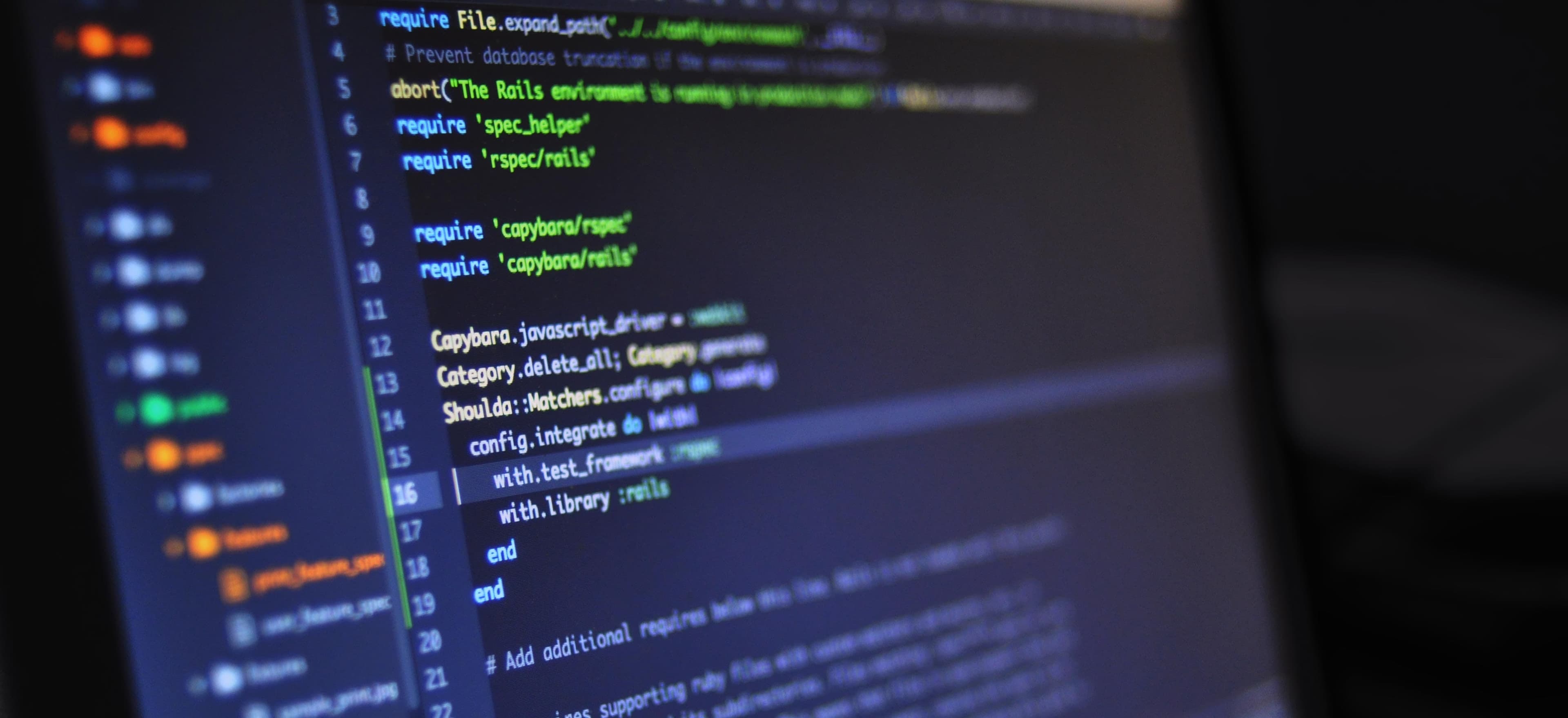
- Published on
Improving Web Scraping Efficiency and Accuracy
Web scraping is a powerful tool for extracting data from websites, but it can be a challenging task due to the dynamic nature of web pages. In this blog post, we will explore some techniques to improve the efficiency and accuracy of web scraping using Java.
Understanding the Basics
Before diving into optimization techniques, it's essential to have a solid understanding of the basics of web scraping. Java provides several libraries, such as Jsoup and Selenium, that can aid in web scraping. Jsoup is a convenient library for extracting and manipulating data, while Selenium is great for interacting with dynamic web pages.
Optimizing Web Scraping Efficiency
1. Use Selectors Wisely
Selectors are patterns used to select the elements you want to scrape from a web page. Utilizing specific and concise selectors can significantly improve the efficiency of your web scraping code. For example, using CSS selectors or XPath expressions to target elements precisely can reduce the time taken to extract the required data.
// Example using Jsoup CSS selector
Elements links = document.select("a[href]");
2. Implement Throttling
Web scraping can put a strain on servers, so it's crucial to implement throttling to control the rate of requests. Adding pauses between requests can prevent overloading the server and getting blocked. This can be achieved using simple techniques like adding a delay with Thread.sleep()
or more advanced approaches with libraries like Apache HttpClient for request rate limiting.
// Example using Thread.sleep() for throttling
try {
Thread.sleep(1000); // Adding a 1-second delay
} catch (InterruptedException e) {
// Handle the exception
}
3. Utilize Parallel Processing
To speed up the scraping process, consider utilizing parallel processing. Java provides various ways to implement parallelism, such as using ExecutorService to execute multiple tasks concurrently. This can significantly reduce the overall scraping time, especially when dealing with a large number of web pages.
// Example using ExecutorService for parallel processing
ExecutorService executor = Executors.newFixedThreadPool(10);
for (String url : urls) {
executor.execute(() -> {
// Scrape the web page
});
}
Improving Web Scraping Accuracy
1. Handle Dynamic Content
Web pages often contain dynamic content loaded through JavaScript, which may not be immediately available in the initial HTML response. Using Selenium in combination with headless browsers like ChromeDriver can help in effectively scraping dynamically generated content by simulating user interactions and waiting for the page to fully load.
// Example using Selenium to handle dynamic content
WebDriver driver = new ChromeDriver();
driver.get("https://example.com");
// Wait for the dynamic content to load
new WebDriverWait(driver, 10).until(ExpectedConditions.visibilityOfElementLocated(By.id("dynamicElement")));
2. Implement Error Handling
Web scraping is prone to errors due to various reasons such as network issues, changes in website structure, or unexpected data formats. Implementing robust error handling mechanisms, such as try-catch blocks and logging, can help in identifying and handling these errors gracefully, ensuring the scraping process continues with minimal disruptions.
// Example using try-catch block for error handling
try {
// Web scraping code
} catch (IOException e) {
// Handle the IO exception
} catch (Exception e) {
// Handle other exceptions
}
3. Regularly Update Selectors
Websites often undergo changes in their structure, which can break existing web scraping code. Regularly updating the selectors used to target elements on the web page is crucial for maintaining the accuracy of the scraping process. Additionally, utilizing techniques like fuzzy matching or fallback selectors can make the code more resilient to changes.
Final Thoughts
Web scraping in Java can be optimized for both efficiency and accuracy by employing various techniques such as utilizing precise selectors, implementing throttling, leveraging parallel processing, handling dynamic content, implementing error handling, and regularly updating selectors. By understanding the nuances of web scraping and applying these optimization strategies, developers can build robust and reliable web scraping solutions.
Remember, while web scraping can provide valuable data, it's important to respect website terms of service and robots.txt guidelines, and to scrape responsibly and ethically.
References: