Handling Message Loss in Apache Kafka for Microservices
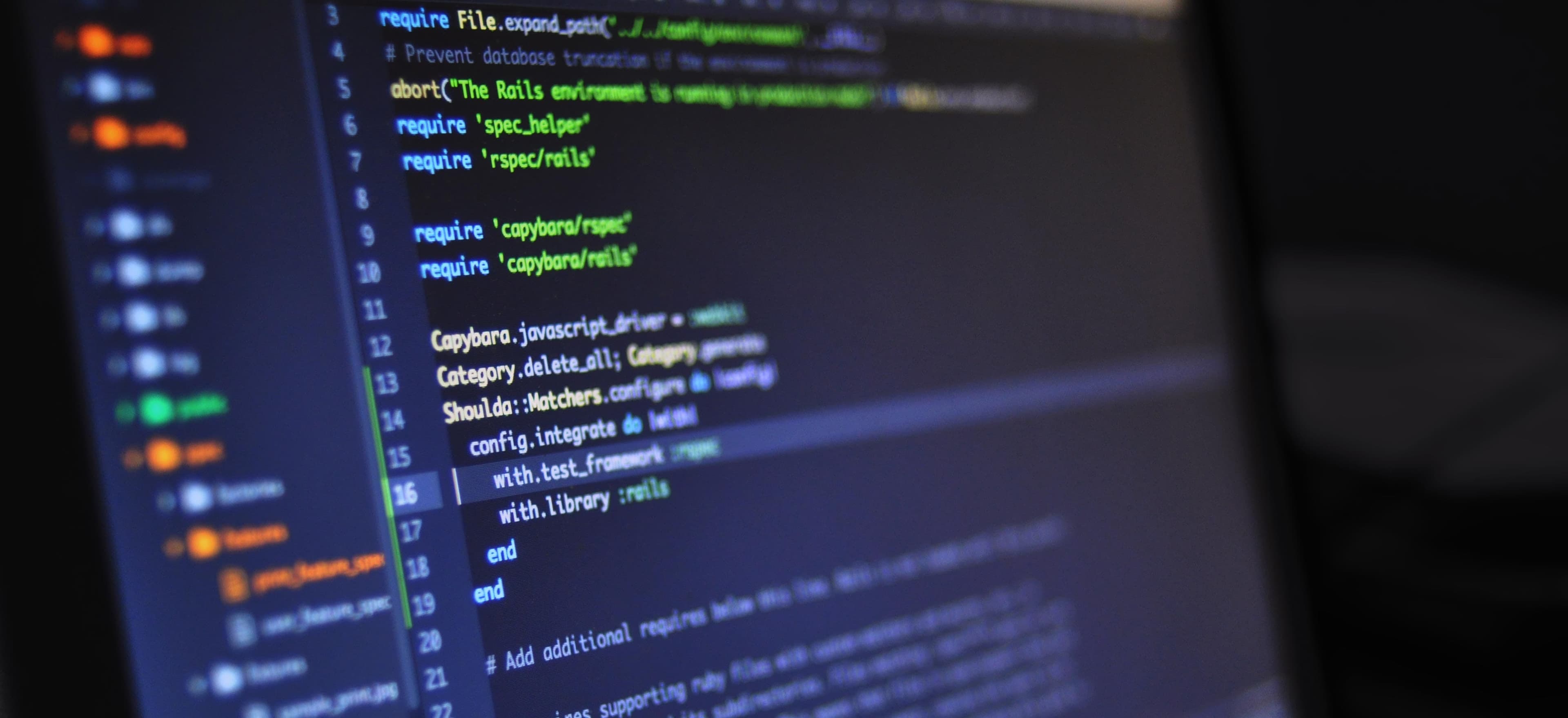
- Published on
Handling Message Loss in Apache Kafka for Microservices
In a microservices architecture, Apache Kafka plays a crucial role in enabling communication between services through messaging. However, ensuring that messages are not lost is vital for maintaining data integrity. In this article, we will explore some strategies for handling message loss in Apache Kafka for microservices.
Understanding Message Loss
Message loss can occur in Apache Kafka due to various reasons such as network issues, service outages, or incorrect configurations. When messages are lost, it can lead to inconsistencies in data processing and affect the overall reliability of the system.
Acknowledgement-based Messaging
One of the fundamental principles for handling message loss in Apache Kafka is to utilize acknowledgment-based messaging. This means that a message consumer acknowledges the successful processing of a message back to the broker. If the acknowledgment is not received, the broker can then reprocess the message or take appropriate action.
Configuring Message Retention
Apache Kafka allows configuring the retention period for messages in topics. By setting an appropriate message retention period, the risk of message loss due to messages aging out can be mitigated. Additionally, configuring log compaction can ensure that the latest value for each key is retained, reducing the possibility of data loss.
Properties props = new Properties();
props.put("message.max.retention.ms", "604800000"); // 7 days
props.put("log.cleaner.enable", "true");
In this code snippet, we set the message retention period to 7 days and enable log compaction to prevent data loss.
Implementing Message Replays
Another approach to handle message loss is by implementing message replay mechanisms. This involves storing consumed message offsets and reprocessing them in case of failure. By using consumer group offsets and tracking the processed messages, it's possible to reprocess any lost messages.
Map<TopicPartition, OffsetAndMetadata> offsets = consumer.committed(partitions);
// Store the offsets externally
// Upon failure, seek to the stored offsets and replay the messages
By implementing message replays, the system can recover from message loss scenarios and maintain data consistency.
Using Idempotent Consumers
Idempotent consumers can help mitigate the impact of message loss by ensuring that processing the same message multiple times has the same result as processing it once. This can be achieved by assigning unique identifiers to messages and handling duplicates appropriately.
consumer.subscribe(Collections.singletonList("topic"));
while (true) {
ConsumerRecords<String, String> records = consumer.poll(Duration.ofMillis(100));
for (ConsumerRecord<String, String> record : records) {
if (!processedMessages.contains(record.key())) {
// Process the message
processedMessages.add(record.key());
}
}
}
In this example, we maintain a set of processed message keys to identify and discard duplicate messages, thereby achieving idempotent message processing.
Monitoring and Alerting
Monitoring and alerting play a crucial role in identifying and addressing message loss issues. By monitoring Kafka consumer lag, message throughput, and broker health, potential message loss scenarios can be detected early.
Integrating monitoring tools like Prometheus and Grafana can provide real-time visibility into Kafka metrics, enabling proactive measures to prevent message loss.
Closing Remarks
In a microservices architecture, ensuring message reliability in Apache Kafka is essential for maintaining data integrity and system stability. By employing acknowledgment-based messaging, configuring message retention, implementing message replays, using idempotent consumers, and setting up robust monitoring and alerting, message loss can be effectively handled in Apache Kafka for microservices.
By implementing these strategies, organizations can build resilient and reliable microservices architectures powered by Apache Kafka.
For further reading on Apache Kafka and microservices, check out the following resources:
- Apache Kafka Documentation
- Microservices Patterns
- Kafka Monitoring with Prometheus and Grafana