Managing Dependency Hell: Google Libraries for Java
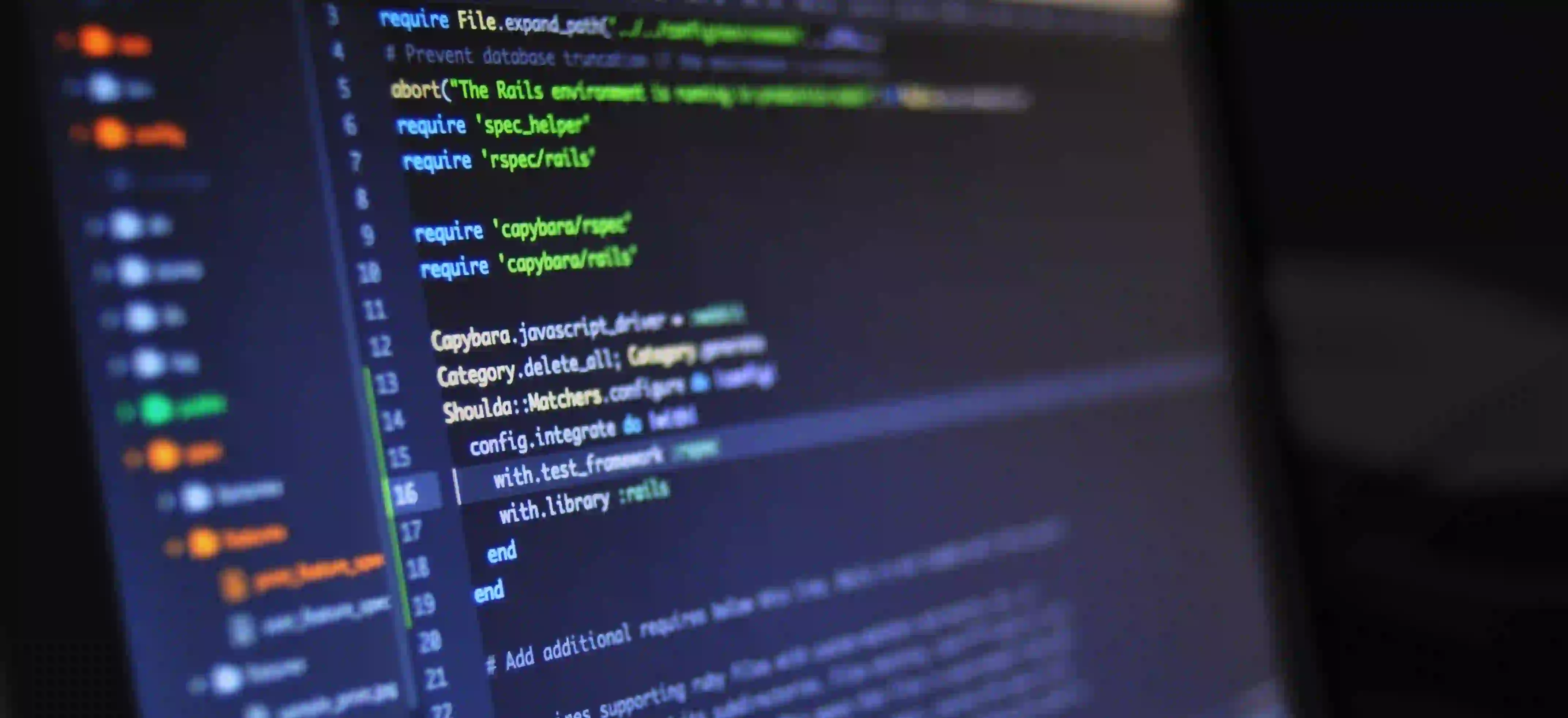
Managing Dependency Hell: Google Libraries for Java
As a Java developer, you're no stranger to the complexities of managing dependencies. Dependency management, when not handled properly, can lead to what developers colloquially refer to as "dependency hell" - a state where the project's dependencies become tangled, conflicting, or outdated. The result? A nightmare of debugging, troubleshooting, and general frustration. However, Google has provided a suite of libraries and tools that can help you not only better manage dependencies but also enhance your Java development experience.
In this blog post, we'll take a closer look at some of the essential and popular Google libraries that you can leverage to streamline your Java projects, boost productivity, and avoid dependency hell.
1. Guava: Google Core Libraries for Java
Dependency management becomes a lot easier when you have a well-maintained set of core libraries at your disposal. This is where Guava comes into play. Guava provides a rich set of core libraries that extend and augment the Java programming language, making common tasks simpler and more reliable, resulting in a boost to your productivity.
Code Example - Using Guava's Preconditions
import com.google.common.base.Preconditions;
public class User {
private String name;
public void setName(String name) {
this.name = Preconditions.checkNotNull(name, "Name must not be null");
}
}
In this example, we're using Guava's Preconditions
to ensure that the name
parameter is not null. This not only helps in writing robust code but also makes the intent of the code clear.
2. Gson: Google's JSON Library for Java
Working with JSON data is a common task for Java developers, and Gson simplifies this process by allowing you to convert Java objects to JSON and vice versa. Gson is not only easy to use, but it also provides customization options for complex JSON-to-Java object mappings.
Code Example - Using Gson to Serialize/Deserialize JSON
import com.google.gson.Gson;
public class Main {
public static void main(String[] args) {
Gson gson = new Gson();
// Serialize Java object to JSON
String json = gson.toJson(new User("John Doe", 30));
// Deserialize JSON to Java object
User user = gson.fromJson(json, User.class);
}
}
Gson's simplicity and ease of use make it an indispensable tool for handling JSON in your Java projects.
3. Guice: Lightweight Dependency Injection Framework
Managing dependencies manually can be error-prone and tedious. Guice addresses this issue by providing a lightweight dependency injection framework for Java. It allows you to externalize object creation and assembly logic, resulting in cleaner, more modular code.
Code Example - Using Guice for Dependency Injection
import com.google.inject.Guice;
import com.google.inject.Injector;
public class Main {
public static void main(String[] args) {
Injector injector = Guice.createInjector(new BillingModule());
BillingService billingService = injector.getInstance(BillingService.class);
billingService.charge();
}
}
In this example, Guice is used to create an injector and perform dependency injection of the BillingService
class. This results in a decoupled and easily testable codebase.
4. Truth: Assertion Framework for Testing
Writing comprehensive and readable tests is crucial for maintaining a robust codebase. Truth is an assertion framework that makes your test assertions more expressive and easier to read, leading to better test coverage and more robust code.
Code Example - Using Truth for Test Assertions
import static com.google.common.truth.Truth.assertThat;
public class UserTest {
@Test
public void testUserAge() {
User user = new User("John Doe", 30);
assertThat(user.getAge()).isEqualTo(30);
}
}
In this test, we're using Truth's assertThat
to clearly express our assertion, making the test intent explicit and readable.
Wrapping Up
Google libraries for Java offer a plethora of tools and utilities that can significantly improve your Java development process. By leveraging libraries such as Guava, Gson, Guice, and Truth, you can mitigate the complexities of dependency management, streamline your codebase, and enhance the testability of your Java applications.
Remember, effective dependency management is essential for the long-term maintainability and scalability of your projects. Incorporating these Google libraries into your Java development toolkit can alleviate the pains of dependency hell and pave the way for more seamless, efficient, and robust software development.
So, next time you find yourself embroiled in the quagmire of dependency management, remember that Google has your back with these powerful Java libraries.
Happy coding!