Handling Fault Tolerance with Hystrix
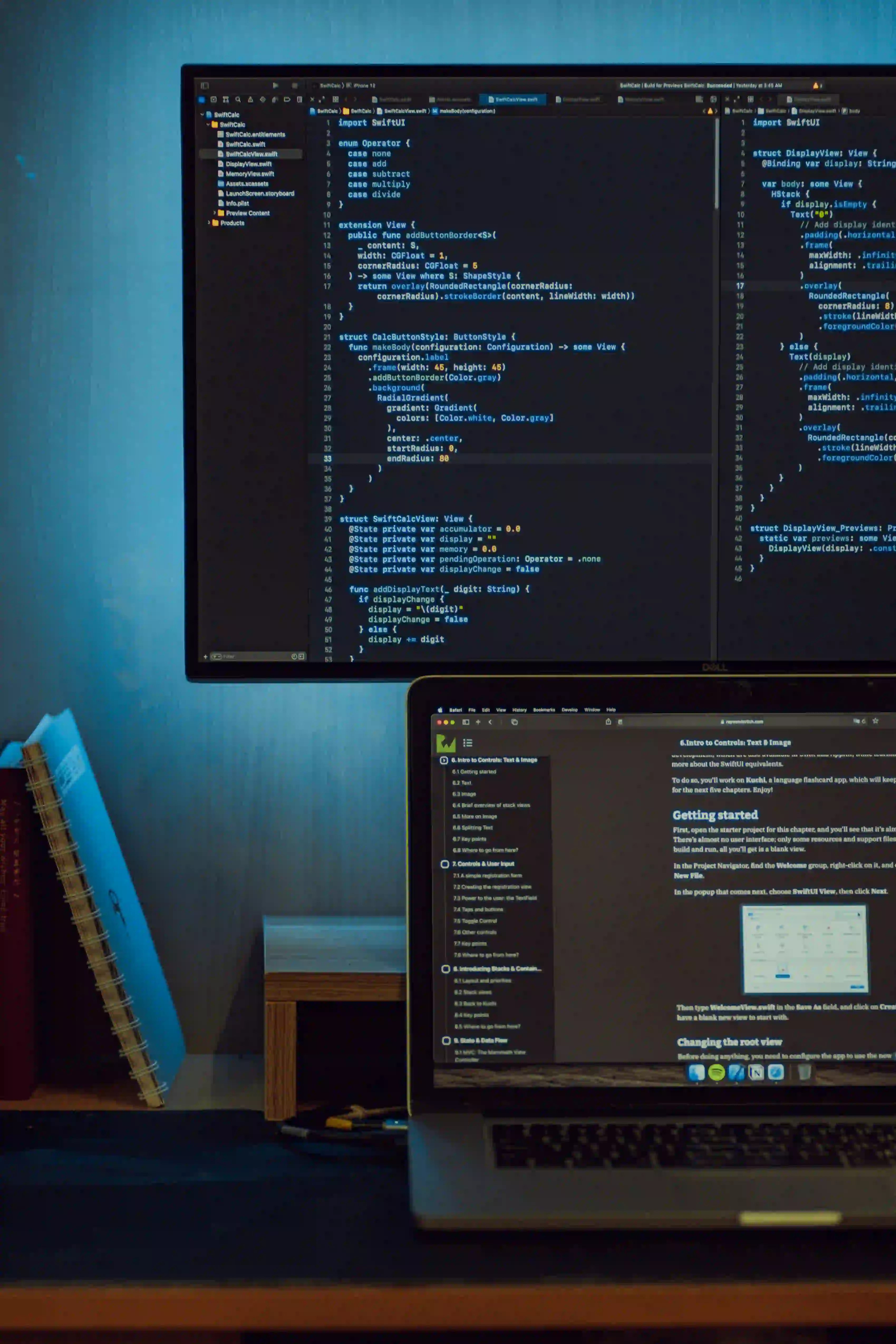
Handling Fault Tolerance with Hystrix
In a distributed system, failures are inevitable. Hence, building fault-tolerant systems is crucial. Netflix's Hystrix, a latency and fault tolerance library, provides an effective solution to handle faults in distributed, microservice-based architectures.
Understanding Hystrix
Hystrix, designed to control the interactions between distributed services, helps in preventing cascading failures and providing fallback options, thereby improving the overall system resilience. It achieves this by isolating points of access between the services, stopping any issues from affecting the entire system.
Setting Up Hystrix
To integrate Hystrix into a Java application, you first need to add the Hystrix dependency to your project's Maven or Gradle configuration.
Maven Dependency
<dependency>
<groupId>com.netflix.hystrix</groupId>
<artifactId>hystrix-core</artifactId>
<version>latest_version</version>
</dependency>
Gradle Dependency
implementation 'com.netflix.hystrix:hystrix-core:latest_version'
With Hystrix added to your project, you can start utilizing its features to enhance fault tolerance in your application.
Implementing Resilient Commands with Hystrix
Hystrix introduces the concept of a "command," which represents the primary mechanism for protecting your application from failures.
Let's create a simple example to demonstrate the implementation of a resilient command using Hystrix.
Example: Implementing a Resilient Command
import com.netflix.hystrix.HystrixCommand;
import com.netflix.hystrix.HystrixCommandGroupKey;
public class HelloCommand extends HystrixCommand<String> {
public HelloCommand() {
super(HystrixCommandGroupKey.Factory.asKey("ExampleGroup"));
}
@Override
protected String run() {
// Code that might fail under normal circumstances
return "Hello, World!";
}
@Override
protected String getFallback() {
// Fallback logic to be executed when the main code fails
return "Fallback: Hello from the fallback method!";
}
}
In this example, we create a HelloCommand
class that extends HystrixCommand
. We override the run
method, which contains the primary logic that might fail. Additionally, we provide a getFallback
method, which defines the fallback logic to be executed in case the main logic fails.
The HystrixCommandGroupKey
provides a way to group commands together for monitoring, alerting, and configuration. Grouping commands is beneficial for understanding the overall health of dependencies and how they might be affecting the system.
Executing Resilient Commands
Once the resilient command is implemented, we need to execute it using a Hystrix command execution.
Executing the Resilient Command
public class Application {
public static void main(String[] args) {
String result = new HelloCommand().execute();
System.out.println(result);
}
}
When running the HelloCommand
, Hystrix handles the execution and applies the necessary fault tolerance strategies such as timeouts, circuit breakers, and fallbacks. This ensures that the application remains responsive and resilient in the face of failures.
Configuring Hystrix Behavior
Hystrix provides a wide range of configuration options to customize its behavior and adapt it to the specific needs of your application. One such configuration is the command timeout, which defines the maximum time a command can take before considering it a failure and triggering the fallback method.
Configuring Command Timeout
@HystrixCommand(commandProperties = {
@HystrixProperty(name = "execution.isolation.thread.timeoutInMilliseconds", value = "3000")
})
public String someMethod() {
// Method logic
}
In this example, the @HystrixCommand
annotation is used to specify the timeout for the someMethod
to 3000 milliseconds. This ensures that if the execution exceeds the defined time, the fallback method is triggered, preventing the command from causing delays in the system.
Monitoring with Hystrix Dashboard
To gain insights into the behavior and performance of the Hystrix commands, you can utilize the Hystrix dashboard, which provides real-time monitoring of the circuit breakers, metrics, and configurations.
By simply adding the Hystrix metrics stream to your application, you can start monitoring the health of your Hystrix commands.
Adding Hystrix Metrics Stream
@SpringBootApplication
@EnableHystrixDashboard
public class HystrixDashboardApplication {
public static void main(String[] args) {
SpringApplication.run(HystrixDashboardApplication.class, args);
}
}
Once the Hystrix dashboard is set up, you can access it through a web browser and gain valuable insights into the behavior of your Hystrix commands, thus enabling you to make informed decisions about the fault tolerance strategies within your application.
Key Takeaways
In a distributed system, fault tolerance is a crucial aspect of ensuring system resilience and stability. With Hystrix, developers can effectively handle faults and latency issues, thereby building robust and reliable microservice architectures. By implementing resilient commands, configuring fault tolerance strategies, and monitoring the system's behavior, Hystrix empowers developers to create systems that gracefully handle failures, providing a better experience for both application users and developers.
By integrating Hystrix into your Java applications and understanding its capabilities, you can elevate your fault tolerance mechanisms and enhance the reliability of your distributed systems.
Start leveraging Hystrix today and elevate your system's fault tolerance capabilities!
For more in-depth information about Hystrix and fault tolerance strategies, check out Netflix's official Hystrix documentation.
Also, keep an eye on the best practices for fault tolerance in microservices architecture to further improve your system's resilience.