Developing a Devfile Registry for Seamless Environment Management
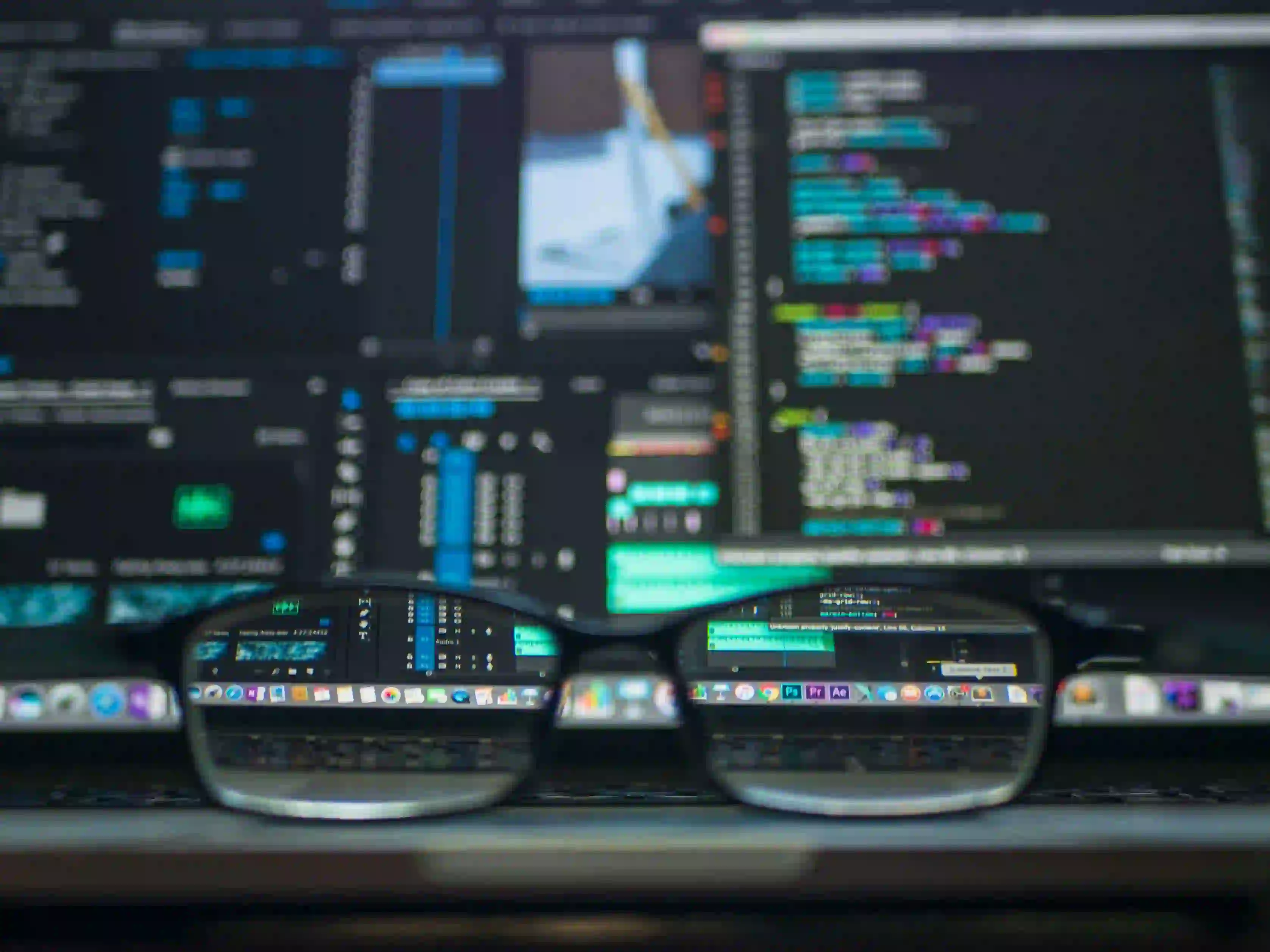
Introduction
Devfiles have revolutionized the way developers manage and share their development environments. They encapsulate the tools, runtimes, and configuration settings required for a project, ensuring consistency across different development machines. However, as the number of devfile-based projects grows, the need for a centralized repository to store and share these devfiles becomes increasingly apparent. In this article, we will explore the concept of a Devfile Registry and discuss the steps to develop one using Java.
Understanding Devfile
Before delving into the concept of a Devfile Registry, let's briefly understand what a devfile is. A devfile is a YAML file that describes the components of a developer environment. It contains information about the runtime, tools, and commands required for a project, thus enabling a consistent and reproducible development environment.
The Need for a Devfile Registry
As the adoption of devfiles rises, developers often find themselves reinventing the wheel by creating devfiles for tools and runtimes that have already been configured by others. A Devfile Registry addresses this inefficiency by providing a centralized platform where developers can discover, share, and reuse devfiles.
Developing a Devfile Registry
Developing a Devfile Registry involves building a platform that allows users to upload, search, and download devfiles. In this section, we will discuss the key components and functionality of a Devfile Registry using Java.
1. Database Management
The backbone of any registry is a robust database that stores the devfiles and associated metadata. In a Java-based Devfile Registry, you can use popular databases like MySQL or PostgreSQL to store this information. The database schema should include fields for devfile name, description, author, tags, and the devfile itself.
// Example schema for devfiles table
CREATE TABLE devfiles (
id INT AUTO_INCREMENT PRIMARY KEY,
name VARCHAR(255) NOT NULL,
description TEXT,
author VARCHAR(100),
tags VARCHAR(255),
devfile_content BLOB
);
2. RESTful API
Building a RESTful API allows users to interact with the Devfile Registry platform. You can use Java frameworks like Spring Boot to create robust and scalable APIs for functionalities such as uploading a devfile, searching for devfiles, and downloading devfiles.
// Example controller to handle devfile operations
@RestController
@RequestMapping("/devfiles")
public class DevfileController {
@Autowired
private DevfileService devfileService;
@PostMapping
public ResponseEntity<String> uploadDevfile(@RequestBody Devfile devfile) {
devfileService.saveDevfile(devfile);
return ResponseEntity.status(HttpStatus.CREATED).body("Devfile uploaded successfully");
}
@GetMapping
public List<Devfile> searchDevfiles(@RequestParam String keyword) {
return devfileService.searchDevfiles(keyword);
}
@GetMapping("/{id}")
public ResponseEntity<byte[]> downloadDevfile(@PathVariable Long id) {
Devfile devfile = devfileService.getDevfileById(id);
HttpHeaders headers = new HttpHeaders();
headers.setContentDispositionFormData("attachment", devfile.getName() + ".yaml");
return new ResponseEntity<>(devfile.getDevfileContent(), headers, HttpStatus.OK);
}
}
3. User Authentication and Authorization
To ensure secure access to the Devfile Registry, implementing user authentication and authorization is crucial. You can use Java security frameworks like Spring Security to handle user authentication, role-based access control, and secure endpoints.
// Example security configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
private UserDetailsService userDetailsService;
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.userDetailsService(userDetailsService).passwordEncoder(passwordEncoder());
}
@Bean
public PasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder();
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http.csrf().disable()
.authorizeRequests()
.antMatchers("/devfiles/**").authenticated()
.and().httpBasic();
}
}
4. Frontend Interface
Creating an intuitive and user-friendly frontend interface is essential for users to interact with the Devfile Registry. Java-based frontend frameworks like Vaadin or Thymeleaf can be used to build the web interface for functionalities such as uploading, searching, and downloading devfiles.
Conclusion
As the demand for streamlined and consistent development environments continues to grow, the significance of a Devfile Registry becomes increasingly evident. By developing a Devfile Registry using Java, developers can benefit from a centralized platform for sharing and reusing devfiles, thereby enhancing productivity and collaboration within the development community.