Understanding Thread Dump Analysis: Solving Concurrency Issues
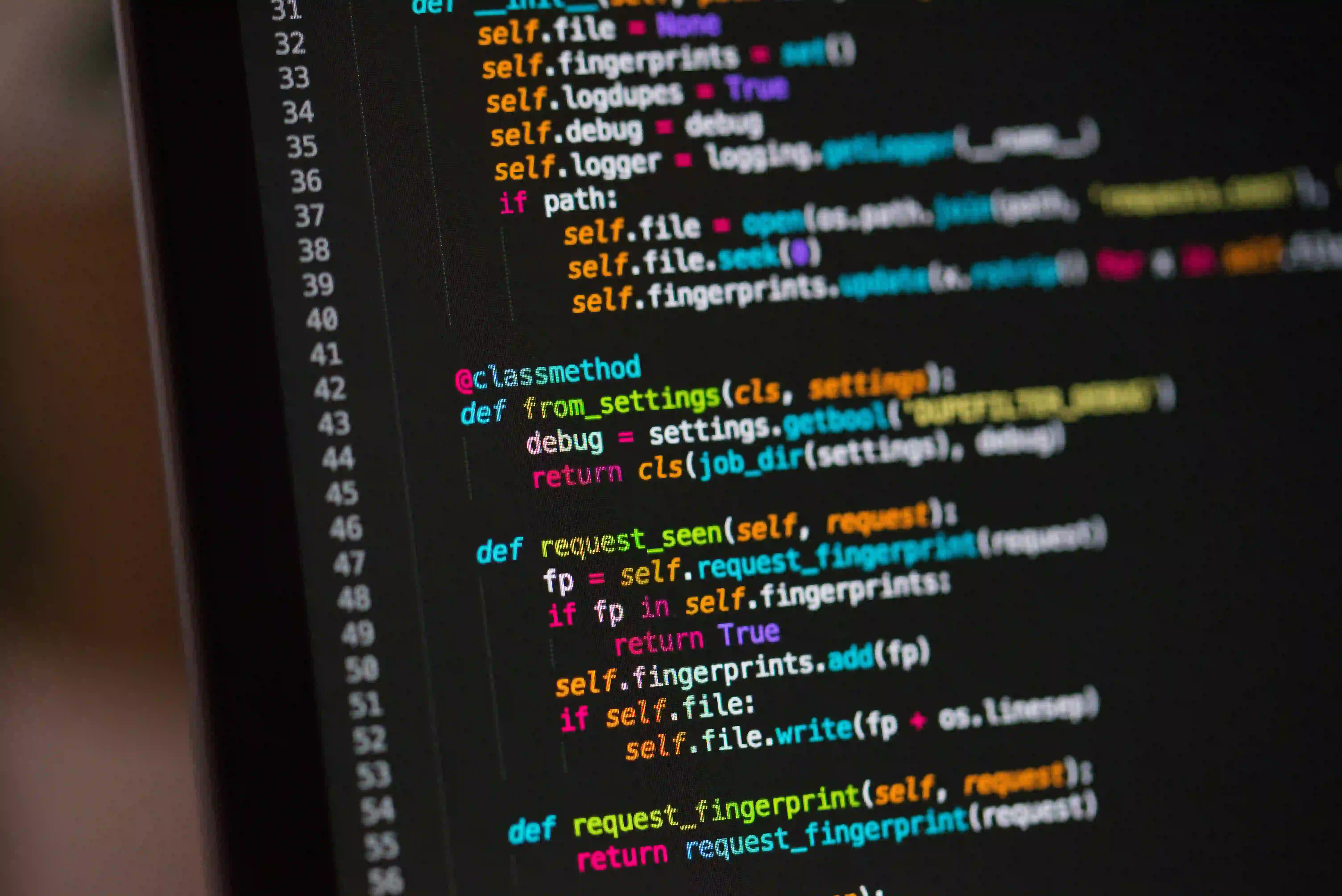
Understanding Thread Dump Analysis: Solving Concurrency Issues
In Java programming, dealing with concurrency issues is a common challenge. When multiple threads access shared resources, it can lead to problems such as race conditions, deadlocks, and thread contention. Thread dumps are a powerful tool for diagnosing such issues, providing valuable insights into the state of the Java Virtual Machine (JVM) and the running threads.
What is a Thread Dump?
A thread dump is a snapshot of the state of all the threads running within a Java process at a particular point in time. It provides information about the threads' state, such as what they are currently doing, whether they are waiting or blocked, and the stack traces of each thread. Analyzing a thread dump can help identify problematic areas in the code where threads are contending for resources or are deadlocked.
Generating a Thread Dump
In a production environment, you can generate a thread dump using tools like jstack, VisualVM, or by sending a signal to the JVM process. For example, to generate a thread dump using jstack, you can run the following command:
jstack <pid>
Replace <pid>
with the process ID of the Java process for which you want to generate the thread dump.
Analyzing a Thread Dump
Once you have a thread dump, the next step is to analyze it to pinpoint concurrency issues and bottlenecks. The following are key areas to focus on during thread dump analysis:
1. Identifying Deadlocked Threads
Look for threads that are in the "BLOCKED" state, as this may indicate a deadlock situation. In the thread dump, you will see the thread's status and the stack trace, which can help you identify the resource that is causing the deadlock.
2. Analyzing Thread States
Examine the states of the threads to understand how they are interacting with each other and with shared resources. Threads in a waiting or blocked state may indicate contention for resources, while threads in a timed waiting state may be waiting for a specific condition to be met.
3. Examining Stack Traces
Inspect the stack traces of the threads to identify the sequence of method calls and understand the code path that led to the current state of each thread. This can help in isolating the code that is causing contention or synchronization issues.
4. Checking for CPU Intensive Threads
Identify threads that are consuming excessive CPU time, as this may indicate inefficient resource usage or poorly designed concurrent algorithms.
Resolving Concurrency Issues
Once you have identified the concurrency issues using the thread dump analysis, you can take several approaches to address them:
Synchronization and Locking
Consider using synchronized blocks, ReentrantLock, or other synchronization mechanisms to control access to shared resources and prevent concurrent modification issues.
Concurrent Data Structures
Utilize concurrent data structures such as ConcurrentHashMap, CopyOnWriteArrayList, and ConcurrentHashMap to minimize contention and improve thread safety when working with shared collections.
Thread Pool Management
Review the usage of thread pools and ensure that the pool size, task queue capacity, and thread timeouts are appropriately configured to avoid thread contention and excessive resource consumption.
Asynchronous and Non-blocking Design
Explore asynchronous and non-blocking programming paradigms using CompletableFuture, Reactive Streams, or asynchronous I/O to design systems that can handle concurrency more efficiently.
Wrapping Up
In conclusion, thread dump analysis is a valuable technique for diagnosing and resolving concurrency issues in Java. By understanding the state of the running threads and identifying contention, deadlocks, and resource bottlenecks, developers can effectively optimize their concurrent applications for better performance and reliability.
Analyzing thread dumps requires a solid understanding of Java concurrency, thread management, and synchronization techniques. It's a crucial skill for Java developers who want to build scalable and efficient concurrent applications.
In the next blog post, we will delve deeper into advanced techniques for thread dump analysis and explore real-world examples of solving complex concurrency problems in Java applications.
For more in-depth information about Java concurrency and thread management, check out the Java Concurrency in Practice book by Brian Goetz.
Stay tuned for the next installment!