Handling Nested Routes in RESTful Express.js API
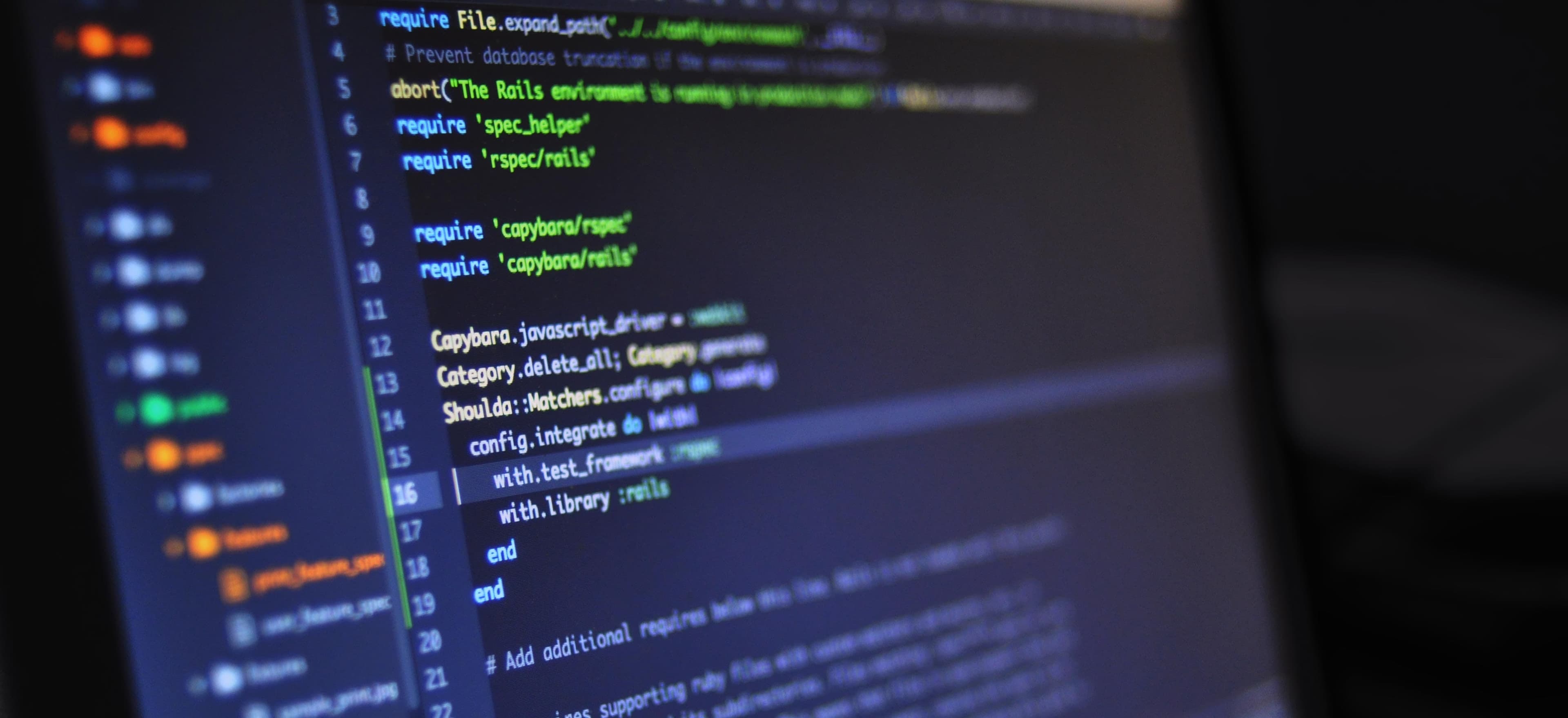
- Published on
Handling Nested Routes in RESTful Express.js API
When building a RESTful API with Express.js, you may encounter scenarios where resources are related in a hierarchical manner. In such cases, handling nested routes becomes essential to maintain a logical and organized API structure.
In this article, we'll explore the concept of nested routes in the context of a Java Express.js API. We'll discuss how to design and implement nested routes to manage related resources effectively.
Understanding Nested Routes
Nested routes involve incorporating resource relationships into the URL structure of an API. This allows for more intuitive and expressive endpoints when dealing with related resources.
For example, consider a scenario where you have a blog application with the following resources:
- Users
- Posts
- Comments
As per the RESTful design, you might want to establish a nested relationship where each post is associated with a specific user, and each comment is associated with a specific post.
To achieve this, you can create nested routes that reflect this relationship in the API endpoint structure.
Designing Nested Routes
In our example, the following URL structure reflects the relationships between users, posts, and comments:
/users
- Represents the collection of users/users/:userId/posts
- Represents the posts created by a specific user/users/:userId/posts/:postId/comments
- Represents the comments on a specific post by a specific user
Implementing Nested Routes in Express.js
Let's dive into the implementation of nested routes in Express.js. We'll use the express
framework to demonstrate the handling of nested routes.
First, we need to define the routes for users, posts, and comments:
const express = require('express');
const app = express();
// Users Route
app.get('/users', (req, res) => {
// Get all users
});
// Posts Route
app.get('/users/:userId/posts', (req, res) => {
// Get posts by userId
});
// Comments Route
app.get('/users/:userId/posts/:postId/comments', (req, res) => {
// Get comments by userId and postId
});
app.listen(3000, () => {
console.log('Server running on port 3000');
});
In this example, we have defined routes for users, posts, and comments with their corresponding URL structure to represent the nested relationship.
Why Nested Routes in Express.js?
- Organized Code Structure: Nested routes help in organizing the codebase by grouping related endpoints together.
- Improved Readability: By reflecting resource relationships in the URL structure, nested routes enhance the readability and understanding of the API.
- Expressiveness: Nested routes allow for a more expressive and intuitive API design, making it easier for developers to work with the API.
Managing Nested Route Parameters
When working with nested routes, it's crucial to extract and handle the nested parameters effectively. In Express.js, route parameters can be accessed using the req.params
object.
Let's consider the /users/:userId/posts/:postId/comments
route. We need to extract the userId
and postId
from the URL to perform further operations.
// Comments Route
app.get('/users/:userId/posts/:postId/comments', (req, res) => {
const { userId, postId } = req.params;
// Use userId and postId to retrieve comments
});
Why Managing Nested Route Parameters is Important
- Data Segregation: Extracting nested parameters enables the segregation of data based on the relationships between resources.
- Targeted Operations: Access to nested parameters allows for targeted operations on specific resources, enhancing precision in API functionality.
Handling Nested Route Actions
Nested routes also involve performing actions specific to the nested relationship. For instance, creating a new post for a user or deleting comments on a post by a specific user.
Let's take the example of adding a new post for a user:
// Add Post Route
app.post('/users/:userId/posts', (req, res) => {
const { userId } = req.params;
// Create a new post for the user with userId
});
In this case, by utilizing the nested route, we can clearly specify that the action is related to a particular user.
Why Handling Nested Route Actions Matters
- Contextual Operations: Nested routes provide context-specific actions, ensuring that operations are performed within the relevant resource relationships.
- Simplified Endpoint Naming: Actions specific to nested relationships can be represented more clearly in endpoint naming, improving the overall API readability and maintainability.
Error Handling in Nested Routes
When dealing with nested routes, it's imperative to ensure proper error handling. This includes validating route parameters, handling resource not found errors, and managing other potential errors specific to nested relationships.
// Error Handling
app.use((err, req, res, next) => {
if (err instanceof CustomNotFoundError) {
res.status(404).json({ error: 'Resource not found' });
} else {
res.status(500).json({ error: 'Internal Server Error' });
}
});
By implementing custom error handling middleware, you can cater to errors related to nested routes and provide appropriate responses.
Why Error Handling in Nested Routes is Vital
- Enhanced Reliability: Proper error handling ensures the reliability of the API, especially when dealing with interdependent resource relationships.
- User-Friendly Responses: Custom error handling allows for tailored error messages, improving the overall user experience when consuming the API.
Final Thoughts
In this guide, we have explored the concept of nested routes in a RESTful Express.js API. By understanding the design and implementation of nested routes, you can create a well-organized, expressive, and straightforward API structure. Additionally, efficient management of nested route parameters, actions, and error handling contributes to the robustness and usability of the API.
By incorporating nested routes, you can build RESTful APIs that elegantly capture the relationships between resources, providing a seamless experience for API consumers.
Start implementing nested routes in your Express.js API to streamline resource management and enhance the overall effectiveness of your API design.