Common Challenges Faced When Learning Scala
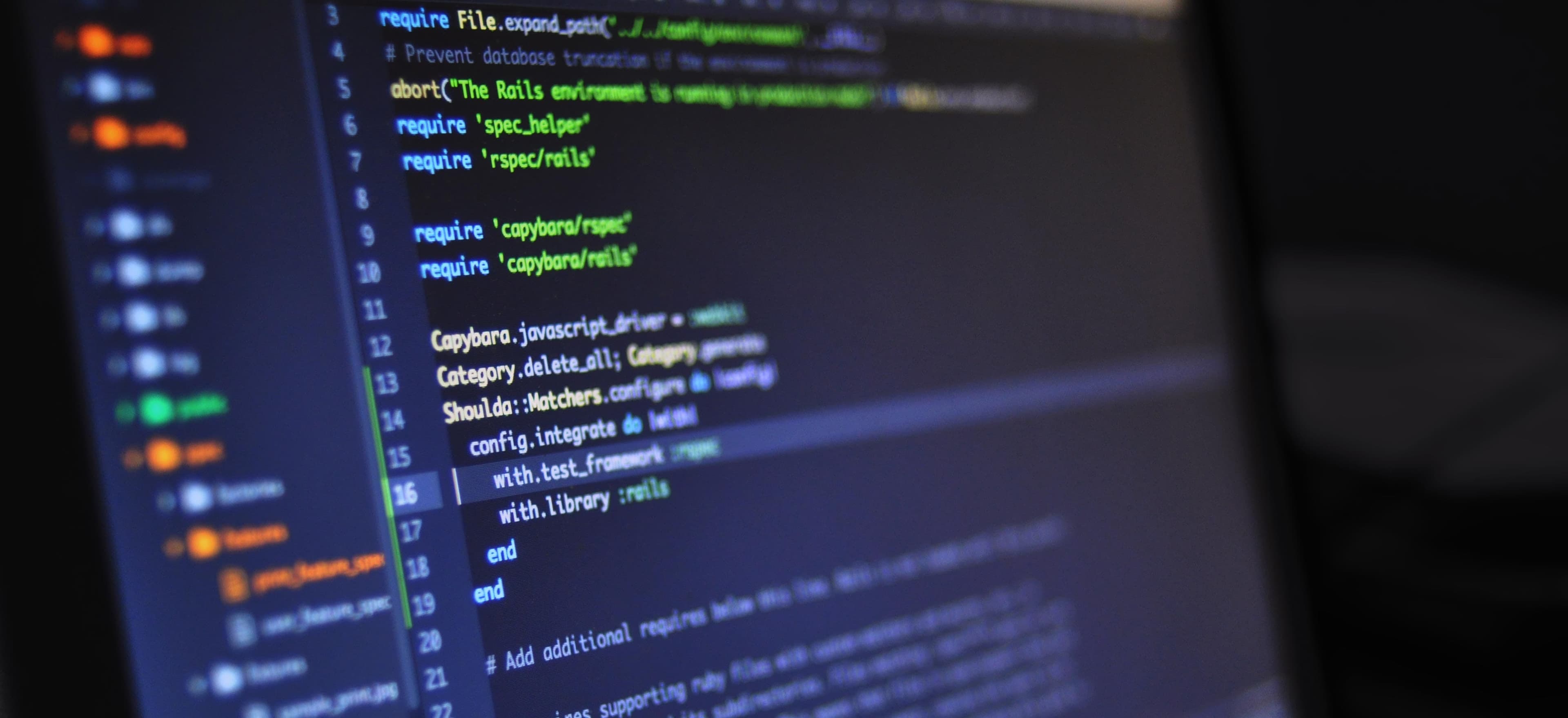
- Published on
Common Challenges Faced When Learning Scala
Scala is a powerful language that blends functional and object-oriented programming paradigms. As a Java developer, learning Scala can be an exciting yet challenging experience. In this post, we'll explore some of the common hurdles that developers face when diving into Scala and provide insights into how to overcome them.
Understanding Functional Programming Concepts
One of the primary challenges for Java developers transitioning to Scala is understanding and effectively applying functional programming concepts. Scala embraces immutability, higher-order functions, and function composition, which may be new concepts for developers accustomed to imperative programming in Java.
// Example of higher-order function in Scala
def operateOnList(list: List[Int], f: Int => Int): List[Int] = {
list.map(f)
}
Understanding the "what" and "why" behind these concepts is crucial for harnessing the full power of Scala. Resources like the Functional Programming Principles in Scala course on Coursera can provide a solid foundation in functional programming with Scala.
Embracing Immutability
In Scala, immutability is encouraged to write safe and concurrent code. Embracing immutability can be a significant shift for Java developers accustomed to mutable data structures and variables. Understanding how to work with immutable collections and variables is key to writing idiomatic Scala code.
// Immutable list in Scala
val numbers = List(1, 2, 3, 4, 5)
By grasping the benefits of immutability, such as avoiding unintended side effects and facilitating concurrent programming, developers can leverage the full potential of Scala.
Grasping the Power of Pattern Matching
Pattern matching is a powerful feature in Scala that allows developers to match complex data structures and extract data succinctly. Java developers may initially find this concept foreign, as it differs significantly from traditional switch statements or if-else chains.
// Example of pattern matching in Scala
def checkNumberType(num: Int): String = num match {
case 0 => "Zero"
case x if x > 0 => "Positive"
case _ => "Negative"
}
Mastering pattern matching can lead to more expressive and concise code. The book "Programming in Scala" by Martin Odersky, the creator of Scala, delves into pattern matching and its applications in depth.
Dealing with the Learning Curve of Type Inference
Scala's strong type inference system can initially pose a learning curve for Java developers. While Java developers are accustomed to explicitly declaring types, Scala's type inference often handles type deduction behind the scenes, leading to more concise yet sometimes perplexing code.
// Type inference in Scala
val message: String = "Hello, Scala!"
val anotherMessage = "Hello, Type Inference!"
Understanding the nuances of Scala's type inference, such as its interplay with inheritance and implicit conversions, is crucial for writing clean and expressive code.
Adapting to Scala's Unique Syntax
Scala's syntax, influenced by both functional and object-oriented paradigms, can appear daunting at first. Features like implicits, traits, and for-comprehensions may seem unfamiliar to developers coming from Java.
// Example of an implicit conversion in Scala
implicit def intToString(x: Int): String = x.toString
val string: String = 42 // Implicit conversion in action
The official Scala documentation serves as an invaluable resource for familiarizing oneself with Scala's syntax and language constructs.
Embracing the Actor Model for Concurrency
Scala's Akka toolkit introduces the actor model for building concurrent, distributed, and resilient applications. For Java developers transitioning to Scala, understanding and harnessing the actor model can be a significant learning curve.
// Example of creating an actor in Akka
class MyActor extends Actor {
def receive = {
case message => println(s"Received message: $message")
}
}
The official Akka documentation and the book "Akka in Action" provide comprehensive insights into leveraging the actor model for building scalable and fault-tolerant systems.
Overcoming the Challenges
The journey of learning Scala as a Java developer is undoubtedly rife with challenges, but each obstacle presents an opportunity for growth and mastery. By delving into the core principles of functional programming, immutability, pattern matching, type inference, syntax, and the actor model, developers can overcome these hurdles and unlock the full potential of Scala.
In conclusion, Scala's expressive syntax, powerful abstractions, and seamless Java interoperability make it a compelling language for Java developers to explore. Embracing the challenges and nuances of Scala ultimately leads to broadening one's programming horizons and becoming a more versatile and skilled developer.
Keep coding, keep learning, and embrace the elegance of Scala!