Effective Exception Handling in Message-Driven Beans
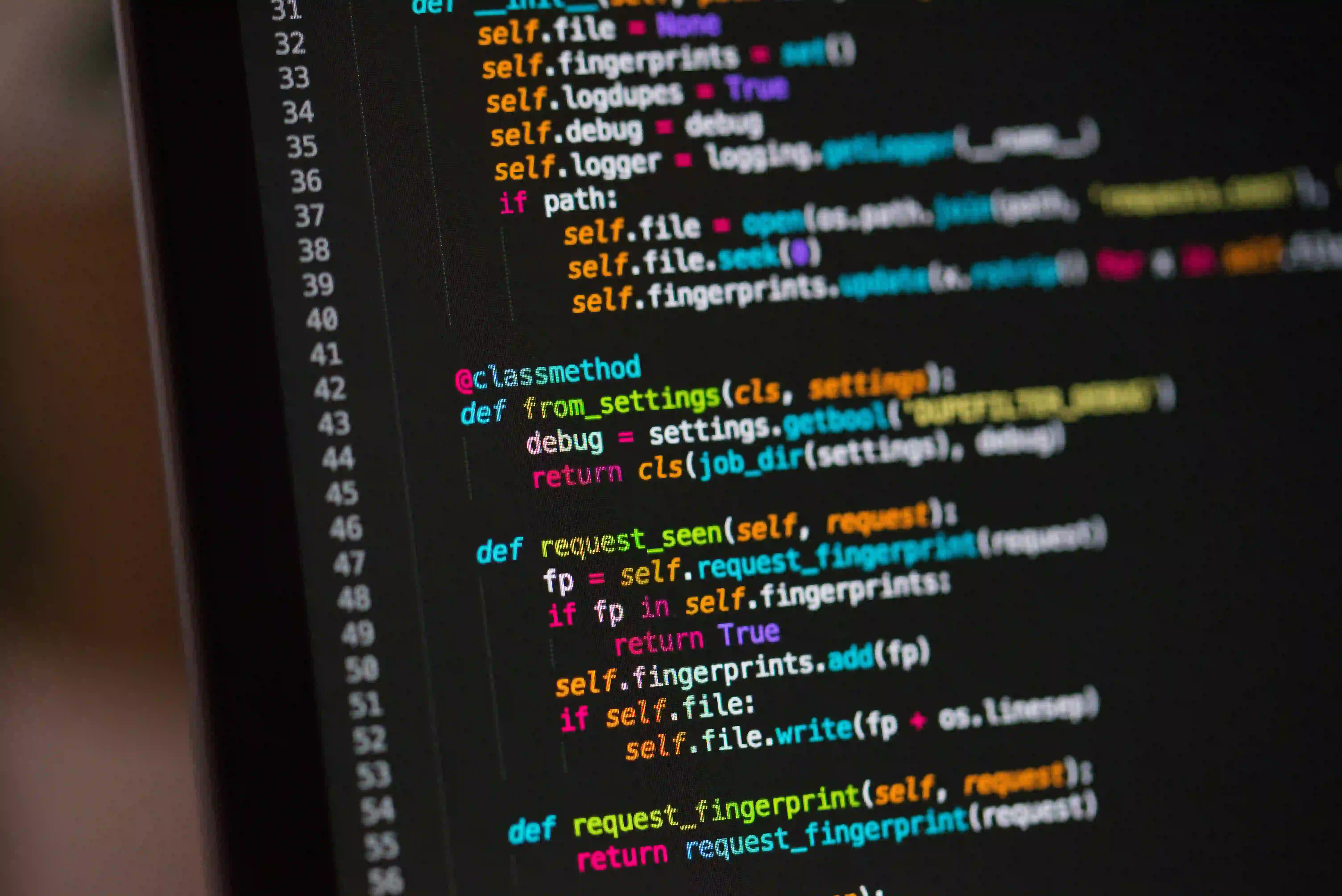
Effective Exception Handling in Message-Driven Beans
In the world of Java EE, Message-Driven Beans (MDBs) are widely used to process messages asynchronously. When dealing with any kind of enterprise application, robust exception handling is crucial to ensure the application's stability, reliability, and maintainability. In this post, we will explore the best practices for handling exceptions in Message-Driven Beans, leveraging Java EE and the Jakarta EE platform to build resilient and fault-tolerant applications.
Understanding Message-Driven Beans
Message-Driven Beans are part of the Java EE and Jakarta EE specifications and are designed specifically to process asynchronous JMS (Java Message Service) messages. They are commonly used in scenarios where decoupling the message sender from the message receiver is necessary, allowing for scalability and reliability in enterprise applications.
Exception Handling Strategies
Exception handling in Message-Driven Beans requires careful consideration due to the asynchronous nature of message processing. Let's delve into some effective strategies for handling exceptions in MDBs.
Logging and Error Reporting
Logging exceptions is a fundamental aspect of exception handling. It provides essential insight into the root cause of an issue and aids in debugging and troubleshooting. Utilize a robust logging framework such as Log4j or Logback to log exceptions and relevant contextual information.
try {
// Message processing code
} catch (Exception e) {
logger.error("Exception occurred while processing message: " + e.getMessage(), e);
}
Dead Letter Queue
A Dead Letter Queue (DLQ) is a designated queue for storing messages that cannot be processed successfully. In the context of Message-Driven Beans, redirecting problematic messages to a DLQ helps prevent message loss and allows for subsequent analysis and reprocessing. Most JMS providers, such as ActiveMQ and IBM MQ, support DLQ configurations.
@MessageDriven(activationConfig = {
@ActivationConfigProperty(propertyName = "destinationType", propertyValue = "javax.jms.Queue"),
@ActivationConfigProperty(propertyName = "destination", propertyValue = "yourQueue"),
@ActivationConfigProperty(propertyName = "DLQMaxResent", propertyValue = "5") // Example configuration for DLQ
})
public class YourMessageDrivenBean implements MessageListener {
// Message processing logic
}
JMS Message Redelivery
JMS providers offer mechanisms for redelivering messages that have failed to be processed. By configuring the redelivery limit and delay, you can control the number of delivery attempts and the time intervals between attempts, providing a way to handle transient failures gracefully.
@MessageDriven(activationConfig = {
@ActivationConfigProperty(propertyName = "destinationType", propertyValue = "javax.jms.Queue"),
@ActivationConfigProperty(propertyName = "destination", propertyValue = "yourQueue"),
@ActivationConfigProperty(propertyName = "maxRedelivery", propertyValue = "5"), // Example configuration for maximum redelivery attempts
@ActivationConfigProperty(propertyName = "redeliveryDelay", propertyValue = "3000") // Example configuration for redelivery delay (in milliseconds)
})
public class YourMessageDrivenBean implements MessageListener {
// Message processing logic
}
Transaction Handling
Ensuring proper transaction handling is critical in Message-Driven Beans to maintain data integrity and consistency. By utilizing container-managed transactions or explicitly managing transactions within the MDB, you can handle exceptions in a transactional context, allowing for rollback and recovery in case of failures.
@TransactionManagement(TransactionManagementType.CONTAINER) // Example declaration for container-managed transactions
@MessageDriven(activationConfig = {
// Activation configuration
})
public class YourMessageDrivenBean implements MessageListener {
// Message processing logic within the transactional context
}
The Bottom Line
Exception handling in Message-Driven Beans is a crucial aspect of building reliable and resilient enterprise applications. By implementing effective exception handling strategies such as logging, dead letter queues, JMS message redelivery, and transaction handling, you can ensure that your Message-Driven Beans can gracefully handle exceptional scenarios, contribute to improved system reliability, and maintain data consistency.
Remember, mastering exception handling in Message-Driven Beans not only elevates the robustness of your application but also enhances your skills as a Java EE developer.
Incorporating effective exception handling in Message-Driven Beans is critical to ensure the stability and reliability of enterprise applications. By following best practices and leveraging features of the Java EE and Jakarta EE platforms, developers can build fault-tolerant and resilient systems.