Uncovering the Importance of RESTful APIs
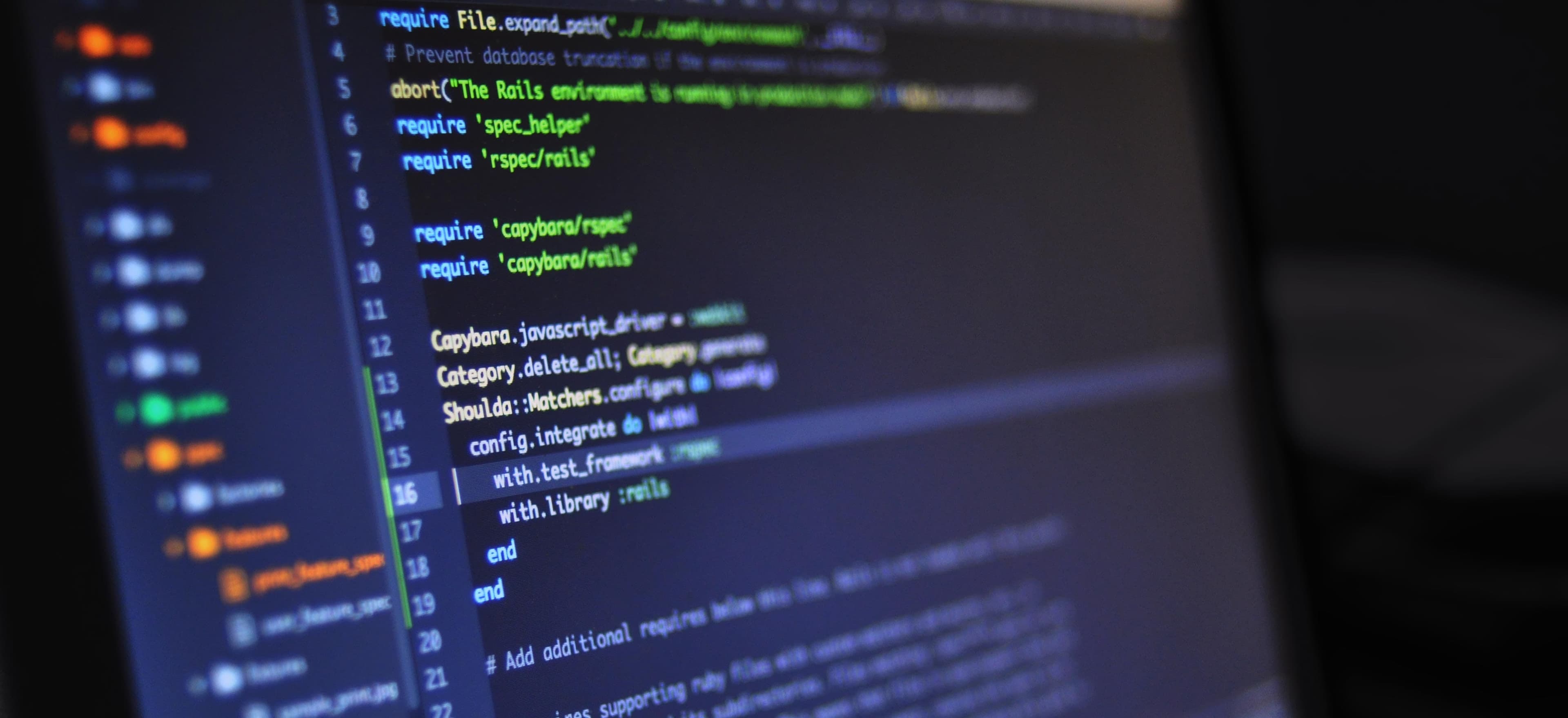
- Published on
Uncovering the Importance of RESTful APIs
In the world of web development, building robust and scalable applications is of utmost importance. Representational State Transfer (REST) has emerged as the primary architectural style for developing networked applications, and its implementation through Application Programming Interfaces (APIs) has become the cornerstone of modern web development.
What is a RESTful API?
RESTful, or Representational State Transfer, refers to an architectural style for designing networked applications. It relies on a stateless, client-server communication protocol, typically the HTTP protocol. RESTful APIs are designed to allow clients to access and manipulate web resources using a predefined set of operations.
RESTful API principles include using standard HTTP methods, such as GET, POST, PUT, DELETE, and PATCH to perform CRUD (Create, Read, Update, Delete) operations on resources. These APIs are known for their simplicity, scalability, and flexibility. They can be consumed by a wide range of clients, from web browsers to mobile devices.
Why are RESTful APIs Important?
Simplicity
One of the key reasons for the widespread adoption of RESTful APIs is their simplicity. By leveraging standard HTTP methods, developers can easily understand and work with these APIs. This simplicity also extends to client-side development, as consuming RESTful APIs in web or mobile applications is straightforward due to the ubiquitous support for HTTP in modern programming languages and frameworks.
Scalability
RESTful APIs are highly scalable, allowing for the modularization of services and the ability to distribute server load. This scalability is vital for applications experiencing growth, as it enables the system to handle increased traffic and data volume without compromising performance.
Flexibility
The flexibility of RESTful APIs is another critical factor in their importance. They allow for multiple data formats such as JSON and XML, making them suitable for a wide range of clients. Additionally, RESTful APIs can support various transport protocols, not limited to HTTP, which provides flexibility in integrating with different systems and technologies.
Compatibility
The design of RESTful APIs aligns with the principles of the web, making them compatible with the existing web infrastructure. This compatibility ensures that RESTful APIs can leverage existing web technologies and infrastructures, enabling seamless integration within the web ecosystem.
Stateless Communication
Statelessness is a fundamental aspect of RESTful APIs. Each request from the client to the server must contain all the information necessary for the server to fulfill that request. This stateless communication simplifies the server implementation and enhances the reliability and visibility of the interactions between clients and servers.
Implementing a Simple RESTful API in Java
Now, let's dive into the implementation of a simple RESTful API using Java.
Prerequisites
Before getting started, make sure you have the following tools installed:
- Java Development Kit (JDK)
- Apache Maven
Setting Up the Project
First, create a new Maven project or use an existing one. Then, add the following dependencies to the pom.xml
file to include the necessary libraries for building the RESTful API:
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
</dependencies>
Creating the REST Controller
Next, create a new Java class for the REST controller. Annotate the class with @RestController
to indicate that it will handle incoming HTTP requests.
import org.springframework.web.bind.annotation.*;
@RestController
@RequestMapping("/api")
public class HelloController {
@GetMapping("/hello")
public String sayHello() {
return "Hello, World!";
}
}
In this example, we've defined a simple GET endpoint that returns a "Hello, World!" message.
Running the Application
To run the application, use the following command:
mvn spring-boot:run
Once the application is running, you can access the RESTful endpoint by making a GET request to http://localhost:8080/api/hello
.
Why Use Java for RESTful APIs?
Java is widely used for building RESTful APIs due to several reasons:
-
Platform Independence: Java's "write once, run anywhere" capability makes it suitable for deploying RESTful APIs across different operating systems and platforms.
-
Robust Ecosystem: Java boasts a vast ecosystem of libraries, frameworks, and tools that facilitate the development, testing, and deployment of RESTful APIs.
-
Scalability: Java's support for multithreading and its robustness in handling concurrent requests make it well-suited for building scalable RESTful APIs.
-
Enterprise Adoption: Many enterprises have existing Java codebases and expertise, making Java a natural choice for developing RESTful APIs within enterprise environments.
-
Security: Java provides strong support for security features, which is crucial for building secure RESTful APIs, especially in enterprise and fintech domains.
Closing the Chapter
RESTful APIs play a crucial role in modern web development, offering simplicity, scalability, flexibility, and compatibility. When implementing RESTful APIs in Java, developers benefit from Java's platform independence, robust ecosystem, scalability, enterprise adoption, and security features. By understanding the importance of RESTful APIs and leveraging Java's capabilities, developers can build resilient and high-performing web applications that cater to the demands of today's interconnected digital landscape.