The Pitfalls of Test Automation: Part 3
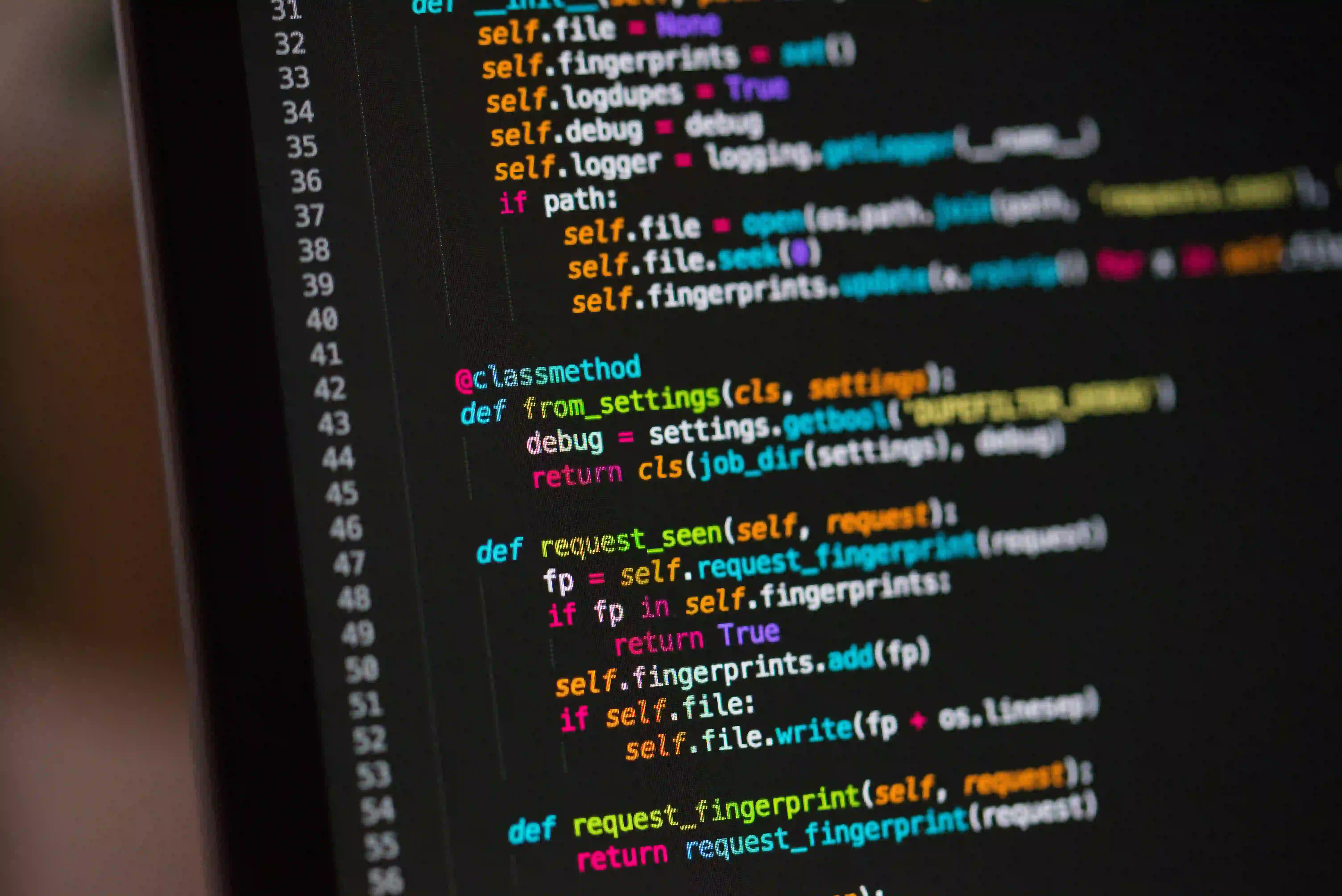
The Pitfalls of Test Automation: Part 3
Welcome back to the third part of our series on the pitfalls of test automation. In this installment, we will delve into the common challenges encountered when using Java for test automation and explore best practices to overcome them. Let's jump right in!
The Java Dilemma
Java, being a versatile and robust programming language, is a popular choice for test automation due to its strong ecosystem and extensive libraries. However, with great power comes great responsibility, and Java test automation is not without its challenges.
Pitfall 1: Over-Engineering Test Cases
One common pitfall in Java test automation is over-engineering test cases. It's easy to fall into the trap of creating complex, overly intricate test cases that are challenging to maintain and debug. This can result in decreased productivity and increased maintenance effort.
To mitigate this, it's essential to follow the KISS (Keep It Simple, Stupid) principle. Focus on writing simple, modular test cases that are easy to understand and maintain. This approach enhances readability and reduces the likelihood of errors. Let's take a look at an example of a simple test case in Java using Selenium WebDriver:
@Test
public void loginTest() {
LoginPage loginPage = new LoginPage(driver);
loginPage.navigate();
loginPage.enterUsername("username");
loginPage.enterPassword("password");
loginPage.clickLoginButton();
assertTrue(homePage.isUserLoggedIn());
}
In the above example, we have a straightforward test case for a login functionality. It follows a linear flow and is easy to comprehend, making maintenance and troubleshooting more straightforward.
Pitfall 2: Not Leveraging Page Object Model
Another common pitfall is not leveraging the Page Object Model (POM) when writing automated tests in Java. Without implementing a POM, test code becomes tightly coupled with the UI, leading to code duplication and increased fragility.
By utilizing POM, we can encapsulate the functionality of the web pages in a separate class, abstracting the interaction with the UI elements. This promotes reusability and maintainability, resulting in more robust and stable test suites.
Let's see how POM can be employed in Java test automation using Selenium WebDriver:
public class LoginPage {
private WebDriver driver;
public LoginPage(WebDriver driver) {
this.driver = driver;
}
public void navigate() {
driver.get("https://www.example.com/login");
}
public void enterUsername(String username) {
driver.findElement(By.id("username")).sendKeys(username);
}
public void enterPassword(String password) {
driver.findElement(By.id("password")).sendKeys(password);
}
public void clickLoginButton() {
driver.findElement(By.id("loginButton")).click();
}
}
In the example above, we have encapsulated the login page functionality in the LoginPage
class, keeping the test code clean and maintainable.
Pitfall 3: Inadequate Exception Handling
In Java test automation, inadequate exception handling can lead to flaky and unreliable tests. Failing to handle exceptions properly can result in unexpected test failures and difficulty in diagnosing the root cause of failures.
By incorporating proper exception handling, such as using try-catch blocks and utilizing assertion frameworks effectively, we can enhance the stability and reliability of our automated tests.
@Test
public void elementPresenceTest() {
try {
assertTrue(driver.findElement(By.id("someElement")).isDisplayed());
} catch (NoSuchElementException e) {
fail("Element not found!");
}
}
In this example, we use a try-catch block to handle the NoSuchElementException
gracefully, providing a custom failure message for better diagnostic information.
The Bottom Line
In conclusion, Java test automation, while powerful, comes with its own set of challenges. By avoiding over-engineering test cases, leveraging the Page Object Model, and implementing adequate exception handling, we can mitigate these pitfalls and build more robust and maintainable test suites.
Stay tuned for the next installment of our series, where we'll explore advanced strategies for overcoming test automation pitfalls. Happy testing!
Remember to check out Selenium and TestNG for additional resources and best practices.