Overcoming Roadblocks in Agile Project Design
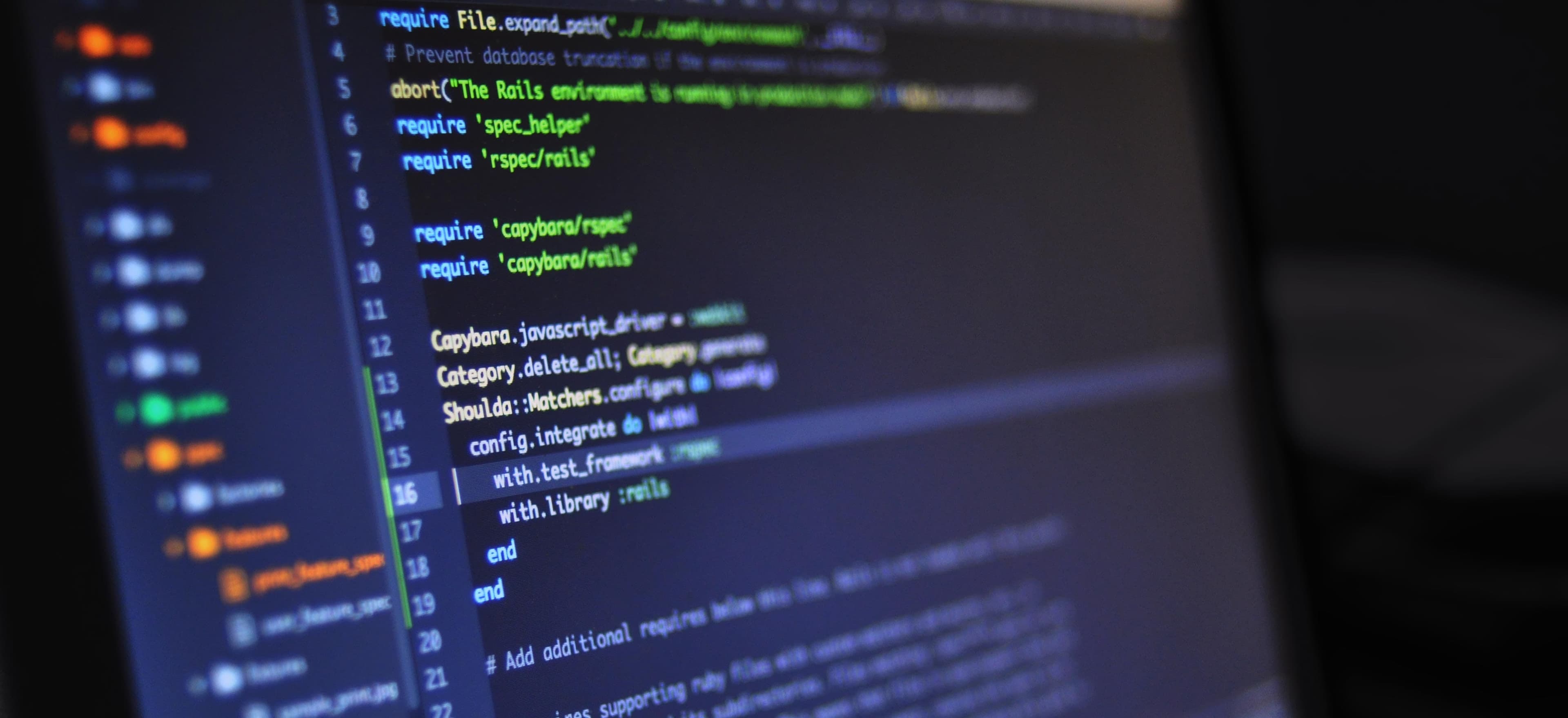
- Published on
Overcoming Roadblocks in Agile Project Design
Agile methodology has gained significant traction in the software development industry due to its iterative, flexible, and customer-centric approach. However, despite its benefits, certain roadblocks can impede the smooth implementation of Agile practices. In this article, we will explore common challenges encountered in Agile project design and discuss strategies to overcome them using Java as a primary development language.
Roadblock 1: Inadequate Test Coverage
A common roadblock in Agile development is the presence of inadequate test coverage, leading to frequent regressions and quality issues. To address this challenge, Java developers can leverage tools like JUnit and Mockito to write comprehensive unit tests.
@Test
public void testCalculateOrderTotal() {
// Arrange
ShoppingCart cart = new ShoppingCart();
cart.addItem(new Item("Product1", 25.0));
cart.addItem(new Item("Product2", 35.0));
// Act
double total = cart.calculateTotal();
// Assert
assertEquals(60.0, total, 0.001);
}
By writing unit tests early in the development cycle, teams can ensure that new code changes do not inadvertently break existing functionality. Additionally, using mocking frameworks like Mockito enables developers to simulate dependencies, ensuring thorough test coverage even in complex scenarios.
Roadblock 2: Integration Challenges
Another significant roadblock is integration challenges, especially when multiple teams work on interconnected modules within the same project. Java provides robust solutions for this, such as utilizing Spring Integration to establish seamless communication between disparate components.
@Component
public class OrderService {
@Autowired
private PaymentService paymentService;
public void processOrder(Order order) {
// Business logic
paymentService.processPayment(order.getTotalAmount());
// More business logic
}
}
Utilizing Spring Integration facilitates the creation of loosely coupled, well-defined integration points, allowing teams to collaborate effectively while ensuring the smooth assimilation of individual components into the larger system.
Roadblock 3: Scalability and Performance Concerns
Scalability and performance bottlenecks can impede the successful delivery of Agile projects, particularly in enterprise-grade applications. Java developers can address these concerns by incorporating efficient data structures and algorithms, such as using ConcurrentHashMap for concurrent access and high-throughput data processing.
ConcurrentMap<String, String> concurrentMap = new ConcurrentHashMap<>();
concurrentMap.put("key1", "value1");
concurrentMap.put("key2", "value2");
Opting for concurrency-aware data structures like ConcurrentHashMap enables applications to handle a large number of concurrent operations while maintaining data integrity and minimizing contention, thereby improving scalability and performance.
Roadblock 4: Evolving Requirements and Changing Priorities
Agile projects often face the challenge of evolving requirements and shifting priorities, making it crucial for teams to adapt swiftly without compromising existing functionality. Java developers can leverage the flexibility of reactive programming using frameworks like Reactor to build responsive and resilient systems capable of gracefully handling changes in requirements.
Flux.just("A", "B", "C")
.flatMap(value -> Mono.just("Processed: " + value))
.subscribe(System.out::println);
By embracing reactive programming, Java applications can react to new inputs and requirements in a non-blocking manner, ensuring that changes are accommodated without disrupting the core functionality.
Roadblock 5: Inadequate Collaboration and Communication
Communication gaps and lack of collaboration among team members can hinder the seamless progression of Agile projects. Java teams can mitigate this roadblock by leveraging communication-enhancing tools like Slack and integrating them with Java-based collaboration frameworks such as Spring Boot to foster real-time interactions and promote transparency.
@RestController
public class NotificationController {
@Autowired
private SlackService slackService;
@PostMapping("/notify")
public ResponseEntity<String> notifyTeam(@RequestBody String message) {
slackService.sendMessage(message);
return ResponseEntity.ok("Notification sent successfully");
}
}
Integrating Java applications with collaboration platforms empowers teams to proactively address issues, share insights, and coordinate effectively, thereby minimizing communication gaps and promoting a cohesive Agile development environment.
Bringing It All Together
While Agile project design may encounter various roadblocks, Java developers possess a wide array of tools, frameworks, and best practices to overcome these challenges effectively. By addressing test coverage, integration complexities, scalability, evolving requirements, and communication impediments, Java teams can ensure the seamless implementation of Agile principles, thereby accelerating the delivery of high-quality, customer-centric software solutions. Embracing these strategies empowers development teams to thrive in an Agile ecosystem, delivering value with efficiency and adaptability.
In conclusion, the fusion of Java's robust capabilities with Agile methodologies paves the way for resilient, adaptive, and successful software delivery.