Implementing a Flexible Domain-Specific Configuration API
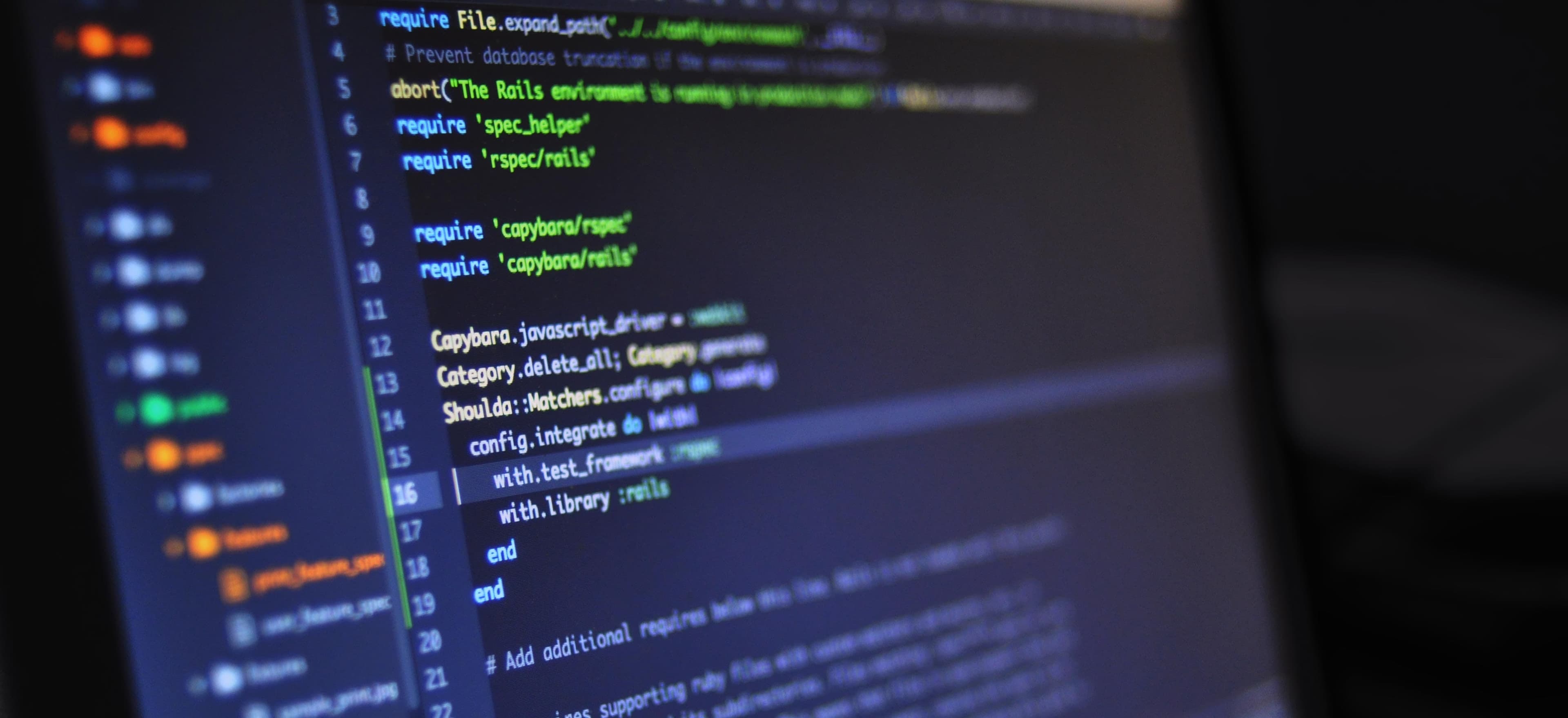
- Published on
Implementing a Flexible Domain-Specific Configuration API in Java
Configuring applications using properties files or environment variables is common, but often not flexible enough to meet the needs of complex systems. In this article, we will explore how to implement a flexible domain-specific configuration API in Java. This approach allows us to define configuration settings specific to our application's domain, providing a simplified way to manage and access configurations.
Defining the Requirements
Before we dive into the implementation, let's define the requirements for our domain-specific configuration API:
- Flexibility: The API should allow for flexible configuration settings that can be easily updated.
- Type Safety: Configuration settings should be strongly typed to provide compile-time type safety.
- Ease of Use: The API should be easy to use and integrate with existing codebases.
- Scalability: It should be scalable for use in small-scale as well as large-scale applications.
- Extensibility: The API should be designed to support future extensions and additional functionality.
Leveraging Java Enums for Type Safety
One way to achieve type safety is by leveraging Java enums to define configuration settings. Enums provide a way to represent a fixed set of constants, which in our case, will correspond to configuration settings. Let's create an example to illustrate this:
public enum AppConfig {
DATABASE_URL("db.url"),
DATABASE_USERNAME("db.username"),
// Add more configuration settings here
private final String key;
AppConfig(String key) {
this.key = key;
}
public String getKey() {
return key;
}
}
In the above code snippet, we've defined an enum AppConfig
with configuration settings such as DATABASE_URL
and DATABASE_USERNAME
, each associated with a corresponding key. This approach provides compile-time type safety when accessing configuration settings.
Implementing the Configuration API
Next, let's implement the configuration API that will provide methods to access and update configuration settings. We'll use a ConfigManager
class to achieve this:
import java.util.HashMap;
import java.util.Map;
public class ConfigManager {
private Map<String, String> configMap;
public ConfigManager() {
this.configMap = new HashMap<>();
// Initialize configMap from properties files, environment variables, or any other source
}
public String getConfig(AppConfig config) {
return configMap.get(config.getKey());
}
public void setConfig(AppConfig config, String value) {
configMap.put(config.getKey(), value);
// Update the configuration source (e.g., properties file) with the new value
}
}
In the ConfigManager
class, we store the configuration settings in a configMap
, and provide methods to get and set configuration values based on the AppConfig
enum. This design allows for a centralized way to manage and access configuration settings.
Integration with Existing Code
To integrate the domain-specific configuration API with existing code, we can use it in the following manner:
public class DatabaseService {
private ConfigManager configManager;
public DatabaseService(ConfigManager configManager) {
this.configManager = configManager;
}
public void connect() {
String url = configManager.getConfig(AppConfig.DATABASE_URL);
String username = configManager.getConfig(AppConfig.DATABASE_USERNAME);
// Use the configuration values to establish a database connection
}
}
In the DatabaseService
class, we inject the ConfigManager
instance via the constructor, and then use it to retrieve the database connection information. This approach decouples the configuration logic from the business logic, making the code more maintainable and testable.
Additional Functionality
To further enhance the flexibility and extensibility of the domain-specific configuration API, we can consider additional functionality such as:
- Validation: Adding validation logic to ensure the correctness of configuration values.
- Dynamic Updates: Supporting dynamic updates to configuration settings without restarting the application.
- Integration with Configuration Providers: Integrating with configuration providers like Apache ZooKeeper or Consul for distributed configurations.
A Final Look
In this article, we've explored how to implement a flexible domain-specific configuration API in Java, catering to the specific needs of an application. By leveraging Java enums for type safety and encapsulating the configuration logic within a dedicated API, we've achieved a more maintainable, scalable, and robust solution. This approach not only simplifies the management of configuration settings but also improves the overall code quality and reliability.
Implementing a domain-specific configuration API in Java can greatly enhance the flexibility and maintainability of applications, and it's a valuable addition to any developer's toolkit.
By following the principles outlined in this article, you can create a powerful and versatile domain-specific configuration API that meets the unique needs of your application. Happy coding!