The Pitfalls of Inverting Opinionated Code
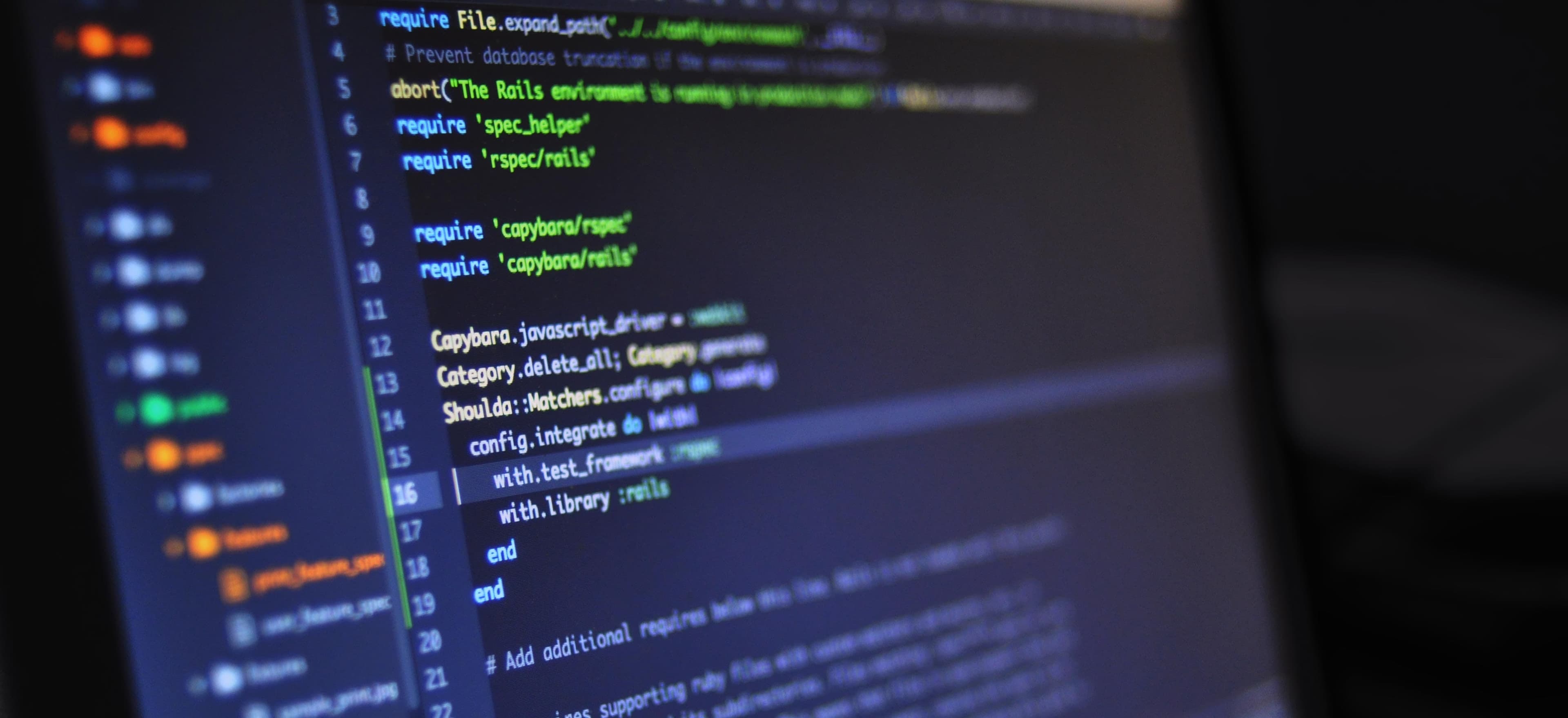
- Published on
The Pitfalls of Inverting Opinionated Code
In the world of Java development, one often encounters libraries, frameworks, and tools that come with their own opinions on how things should be done. While these opinions can be helpful in getting developers up and running quickly, they can also lead to what is known as "opinionated code." This term refers to code that closely adheres to the conventions and patterns mandated by the library or framework in use.
Understanding Opinionated Code
Opinionated code, in the context of Java, can be seen in frameworks like Spring, which strongly advocates for dependency injection using annotations like @Autowired
. While these conventions can streamline development, they also bind the codebase to the choices made by the framework. This can lead to a lack of flexibility and difficulty in migrating to newer technologies.
The Temptation to Invert
In response to the rigidity of opinionated code, an alternative approach called "inversion of control" has gained popularity. Inversion of control (IoC) promotes the idea of externalizing the control flow of the application, thereby allowing developers to have more flexibility in choosing the components and their interactions.
The Pitfalls
While IoC can provide greater flexibility and reduce the tight coupling associated with opinionated code, it is not without its own set of pitfalls. Let's explore some of the challenges that come with inverting opinionated code.
Complexity and Overhead
One of the main pitfalls of inverting opinionated code is the potential increase in complexity and overhead. In an attempt to achieve more flexibility, developers may end up introducing layers of abstraction, configuration files, and additional code that can be harder to understand and maintain.
// Example of increased complexity with IoC
public class ExampleService {
private final DependencyA dependencyA;
private final DependencyB dependencyB;
public ExampleService(DependencyA dependencyA, DependencyB dependencyB) {
this.dependencyA = dependencyA;
this.dependencyB = dependencyB;
}
// Other methods and logic here
}
In the above example, the use of inversion of control can lead to a constructor with multiple dependencies, potentially making the code more convoluted.
Dependency Management
Inverting opinionated code can also lead to challenges in managing dependencies. With the introduction of IoC containers or custom dependency injection mechanisms, the burden of managing and wiring dependencies is shifted to the developers. This can result in an increased risk of misconfigurations and runtime errors.
Learning Curve
Introducing inversion of control to a codebase can also steepen the learning curve for new developers joining the project. While opinionated code often comes with clear conventions and patterns, an inverted codebase may require a deeper understanding of the custom IoC solution in place.
Potential for Over-Engineering
In the quest for flexibility, there is a risk of over-engineering the solution. Developers may be tempted to introduce IoC containers, AOP (Aspect-Oriented Programming), or other advanced techniques without a clear understanding of the actual benefits they provide.
Debugging and Troubleshooting
When things go wrong in an inverted codebase, debugging and troubleshooting can become more challenging. The indirection introduced by IoC containers and dynamic wiring can make it harder to pinpoint the root cause of issues.
Mitigating the Pitfalls
While the pitfalls of inverting opinionated code are real, they can be mitigated with careful consideration and best practices.
Keep It Simple
When adopting inversion of control, strive to keep the implementation as simple as possible. Avoid introducing unnecessary abstractions and layers unless they provide clear benefits to the codebase.
Dependency Injection Frameworks
Instead of rolling out a custom IoC solution, consider using established dependency injection frameworks like Guice or Dagger, which provide IoC capabilities without the need for extensive custom implementations.
Documentation and Onboarding
To address the steeper learning curve, invest in comprehensive documentation and onboarding processes for new developers. Clear explanations of the IoC setup and usage can ease the transition for those joining the project.
Code Reviews and Mentoring
Regular code reviews and mentoring sessions can help prevent over-engineering and guide developers in making informed decisions when applying inversion of control principles.
Testing and Monitoring
Place a strong emphasis on testing and monitoring in an inverted codebase. Rigorous testing can help uncover misconfigurations and issues early on, while monitoring can aid in identifying performance bottlenecks introduced by IoC.
The Last Word
Inverting opinionated code through the adoption of inversion of control principles can offer desirable benefits such as flexibility and decoupling. However, it is crucial to approach this inversion with caution, considering the potential pitfalls it entails. By carefully managing complexity, dependencies, and the learning curve, and by leveraging established best practices, the pitfalls of inverting opinionated code can be effectively mitigated, leading to a codebase that strikes a balance between flexibility and maintainability.
In conclusion, understanding the potential pitfalls of inverting opinionated code and taking proactive steps to address them is essential for ensuring a successful transition to an IoC-based architecture.
For further reading on this topic, you can delve into the specifics of Guice and Dagger, two established dependency injection frameworks known for their effectiveness in managing IoC in Java applications.
Remember, striking the right balance between flexibility and maintainability is key to achieving a resilient and adaptable codebase.
Now go forth and code with confidence, keeping in mind the pitfalls and best practices when considering the inversion of opinionated code in your Java projects.