Optimizing Game Performance with LibGDX Framework
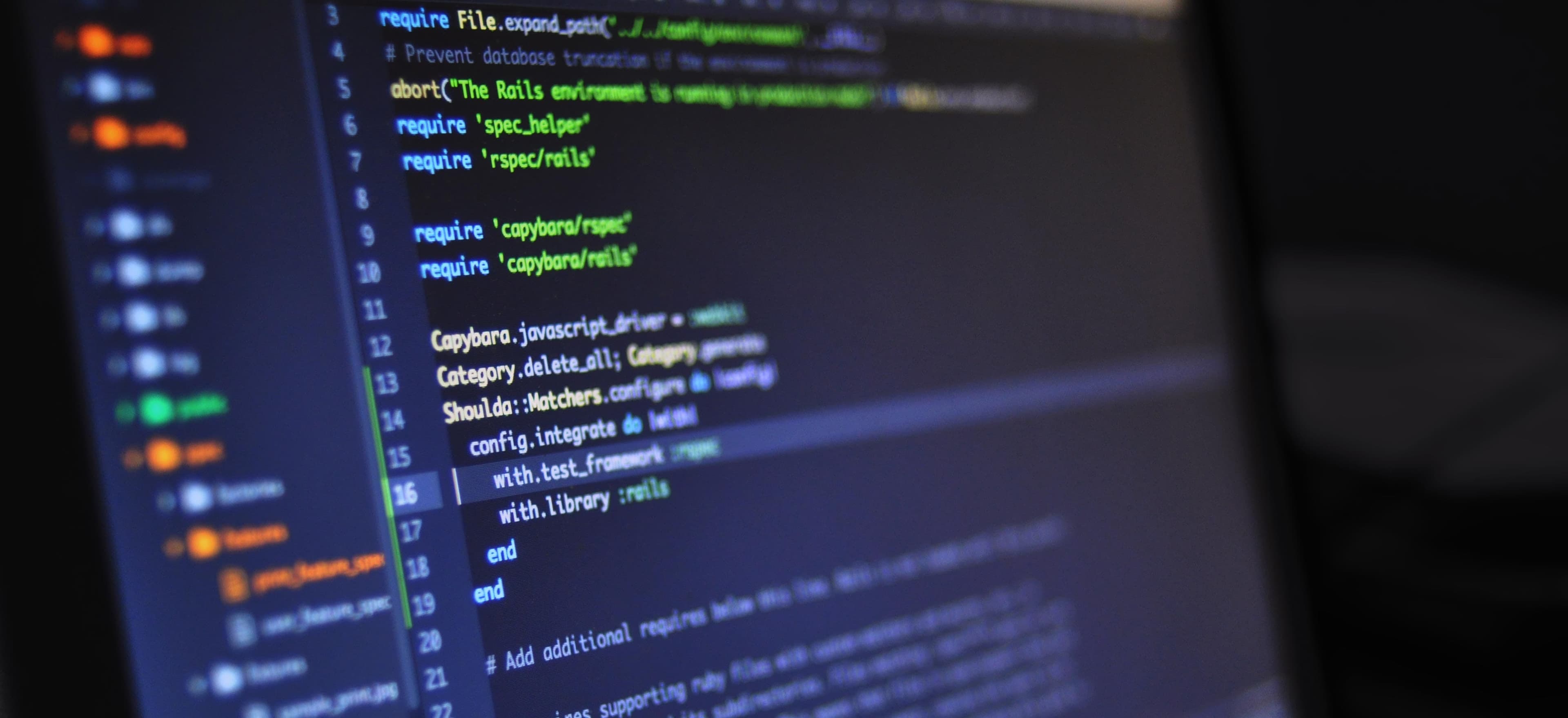
- Published on
Optimizing Game Performance with LibGDX Framework
Developing a game with smooth and seamless performance is crucial for providing an enjoyable user experience. As a Java game developer, understanding how to optimize game performance using the LibGDX framework can greatly enhance the quality of your games. In this article, we will explore various techniques and best practices for optimizing game performance with the LibGDX framework.
Understanding the Importance of Game Performance Optimization
Before diving into the optimization techniques, let's first understand why game performance optimization is so vital. Game performance directly impacts the overall user experience, including aspects such as smooth gameplay, responsive controls, and consistent frame rates. Poor performance can lead to player frustration and negative reviews, ultimately affecting the success of your game.
Profiling Your Game
Before optimizing game performance, it's essential to profile your game to identify performance bottlenecks. Profiling tools such as Java Flight Recorder and VisualVM can provide insights into CPU usage, memory allocation, and thread activity. By analyzing the profiling data, you can pinpoint areas of your game that require optimization.
Efficient Memory Management
Memory management plays a significant role in game performance. Inefficient memory usage can lead to garbage collection pauses, causing frame rate drops and gameplay hiccups. To mitigate these issues, consider the following memory management strategies:
Object Pooling
Object pooling involves reusing objects instead of creating and destroying them frequently. This can reduce the frequency of garbage collection and mitigate memory churn. Here's a simple example of object pooling for bullets in a game:
public class BulletPool {
private Array<Bullet> bullets = new Array<>();
public Bullet obtain() {
if (bullets.size > 0) {
return bullets.removeIndex(bullets.size - 1);
} else {
return new Bullet();
}
}
public void free(Bullet bullet) {
bullets.add(bullet);
}
}
By reusing bullets from the pool, you can avoid continuous object instantiation and destruction.
Texture Atlases
Incorporating texture atlases for sprites and textures can reduce memory overhead and improve rendering performance. Texture atlases pack multiple images into a single texture, reducing the number of texture binds and draw calls. LibGDX provides the TexturePacker
tool to create texture atlases from individual images, optimizing memory usage and rendering efficiency.
Rendering Optimization
Efficient rendering is crucial for achieving smooth frame rates and responsive visuals. Consider the following techniques to optimize rendering performance in your game:
Sprite Batching
Sprite batching involves combining multiple draw calls into a single batch, reducing the overhead of draw calls and state changes. In LibGDX, the SpriteBatch
class facilitates sprite batching. For example:
SpriteBatch spriteBatch = new SpriteBatch();
// Begin batch
spriteBatch.begin();
// Render multiple sprites
spriteBatch.draw(texture1, x1, y1);
spriteBatch.draw(texture2, x2, y2);
// ...
// End batch
spriteBatch.end();
By rendering sprites using a single batch, you can minimize the performance impact of draw calls.
Use of OpenGL ES Features
Utilize OpenGL ES features supported by LibGDX, such as vertex buffer objects (VBOs) and shaders, to offload rendering work to the GPU and enhance rendering performance. Understanding and leveraging these low-level graphics features can significantly improve the efficiency of rendering complex scenes and effects.
Optimizing Input Handling
Responsive input handling is crucial for a satisfying gaming experience. Inefficient input processing can lead to delayed or stuttering controls. Optimize input handling by:
Input Polling
Avoid excessive polling of input devices such as keyboards, mice, and touchscreens. Instead, use event-driven input handling to process input events only when they occur, reducing CPU overhead and improving responsiveness.
Closing the Chapter
Optimizing game performance with the LibGDX framework involves a combination of efficient memory management, rendering optimization, and streamlined input handling. By profiling your game, identifying performance bottlenecks, and implementing the discussed optimization techniques, you can deliver a smooth and immersive gaming experience to your players.
Incorporating these optimization techniques in your game development workflow will not only enhance the performance of your games but also contribute to a more enjoyable and immersive user experience.
Remember, consistent testing and iteration are essential for fine-tuning the performance of your game. By continuously optimizing and refining your code, you can ensure that your game delivers a consistently high level of performance across various platforms and devices.