Custom Content Handling in JAX-RS 2.0
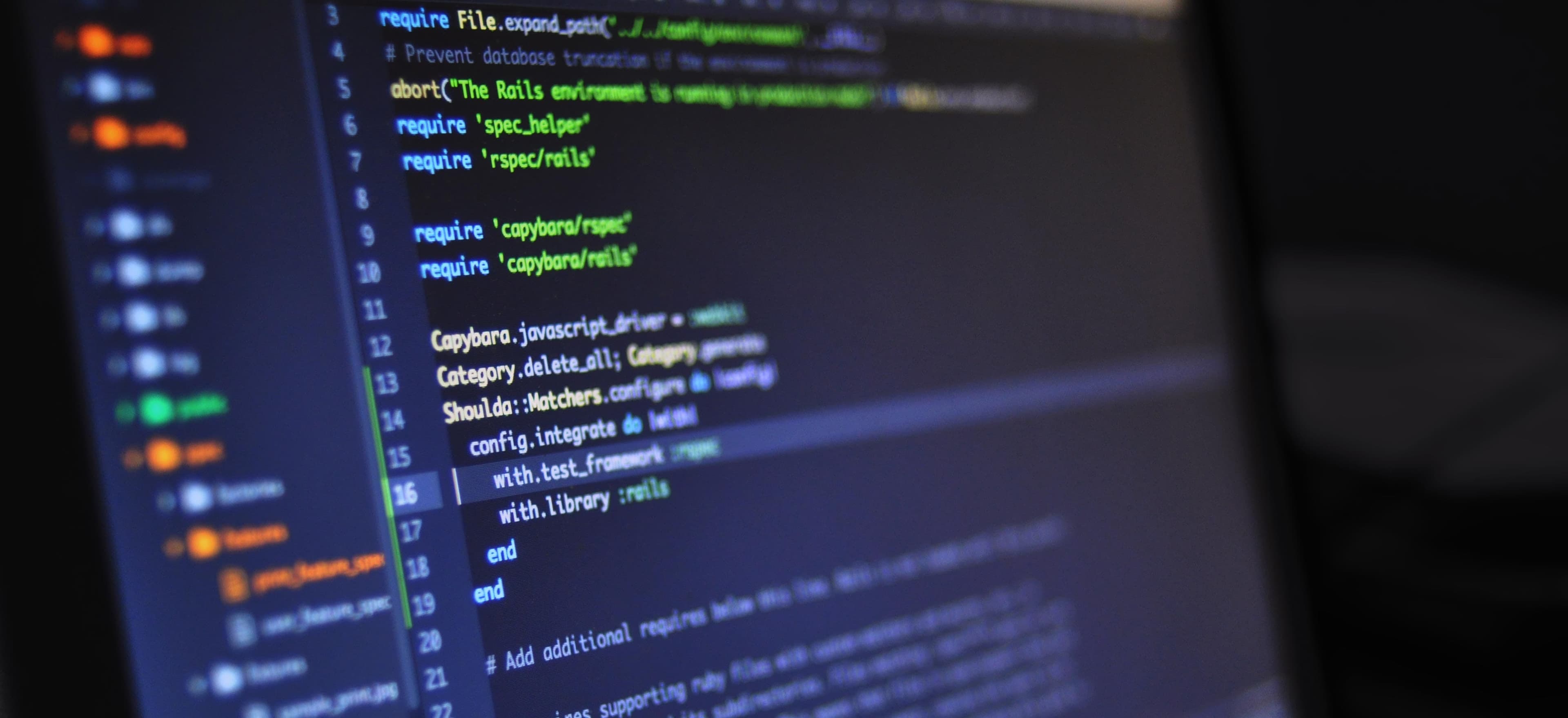
- Published on
Custom Content Handling in JAX-RS 2.0
When working with Java web applications, it's common to encounter the need for customized content handling. With the release of JAX-RS 2.0, developers gained more flexibility in managing content types. In this article, we'll explore how to implement custom content handling in JAX-RS 2.0, allowing for seamless integration of diverse data formats within your web services.
Understanding Content Handling
Content handling in JAX-RS is crucial for managing the exchange of data between clients and servers. This encompasses not only standard data formats like JSON and XML but also custom or proprietary formats that may be specific to your application's requirements.
Standard Content Handling
JAX-RS provides built-in support for standard media types like JSON and XML through its @Produces
and @Consumes
annotations. These annotations allow you to specify the content types that your resource methods can produce or consume.
@GET
@Produces(MediaType.APPLICATION_JSON)
public Response getJsonData() {
// Method implementation
}
Custom Content Handling
When it comes to handling custom content types, JAX-RS 2.0 enables developers to extend the framework's capabilities to support proprietary data formats. This can be achieved through message body readers and writers, which are responsible for serializing and deserializing data.
Implementing Custom Message Body Readers and Writers
Message body readers and writers are the cornerstone of custom content handling in JAX-RS. These components are used to process incoming and outgoing entity bodies based on specific content types.
Custom Message Body Reader
Let's start by creating a custom message body reader for a hypothetical CustomFormat
data type. In this example, we'll assume that CustomFormat
is a proprietary format that needs to be supported by our JAX-RS application.
@Provider
@Consumes("application/custom-format")
public class CustomFormatReader implements MessageBodyReader<CustomFormat> {
@Override
public boolean isReadable(Class<?> type, Type genericType, Annotation[] annotations, MediaType mediaType) {
return type == CustomFormat.class;
}
@Override
public CustomFormat readFrom(Class<CustomFormat> type, Type genericType, Annotation[] annotations, MediaType mediaType, MultivaluedMap<String, String> httpHeaders, InputStream entityStream) throws IOException, WebApplicationException {
// Read and deserialize the entityStream into a CustomFormat object
}
}
In the CustomFormatReader
class, we implement the MessageBodyReader
interface and annotate the class with @Provider
and @Consumes
to indicate that it is a provider component for consuming the application/custom-format
media type.
Custom Message Body Writer
Similarly, we can create a custom message body writer to handle the serialization of CustomFormat
objects.
@Provider
@Produces("application/custom-format")
public class CustomFormatWriter implements MessageBodyWriter<CustomFormat> {
@Override
public boolean isWriteable(Class<?> type, Type genericType, Annotation[] annotations, MediaType mediaType) {
return type == CustomFormat.class;
}
@Override
public long getSize(CustomFormat customFormat, Class<?> type, Type genericType, Annotation[] annotations, MediaType mediaType) {
// Return the size of the serialized entity
}
@Override
public void writeTo(CustomFormat customFormat, Class<?> type, Type genericType, Annotation[] annotations, MediaType mediaType, MultivaluedMap<String, Object> httpHeaders, OutputStream entityStream) throws IOException, WebApplicationException {
// Serialize and write the CustomFormat object to the entityStream
}
}
In the CustomFormatWriter
class, we implement the MessageBodyWriter
interface, annotate the class with @Provider
, and use @Produces
to specify that it is a provider component for producing the application/custom-format
media type.
Registering Custom Providers
Once the custom message body readers and writers are implemented, they need to be registered with the JAX-RS application. This can be done using the Application
subclass or by directly registering the providers in the resource configuration.
Application Subclass
@ApplicationPath("/api")
public class CustomApplication extends Application {
@Override
public Set<Object> getSingletons() {
Set<Object> singletons = new HashSet<>();
singletons.add(new CustomFormatReader());
singletons.add(new CustomFormatWriter());
return singletons;
}
}
In this Application
subclass, we override the getSingletons
method to include instances of the custom message body readers and writers. By doing so, these providers become part of the JAX-RS application configuration.
Resource Configuration
If you prefer to register the providers directly in the resource configuration, you can use the ResourceConfig
class as follows:
public class CustomResourceConfig extends ResourceConfig {
public CustomResourceConfig() {
register(CustomFormatReader.class);
register(CustomFormatWriter.class);
}
}
In this example, we extend the ResourceConfig
class and register the custom message body readers and writers in its constructor.
Consuming and Producing Custom Content
With the custom message body readers and writers in place and registered with the JAX-RS application, you can now consume and produce custom content types in your resource methods.
Consuming Custom Content
To consume a custom content type in a resource method, you can use the @Consumes
annotation with the corresponding media type.
@POST
@Consumes("application/custom-format")
public Response processCustomData(CustomFormat customFormat) {
// Process the CustomFormat object
}
Producing Custom Content
Similarly, to produce a custom content type in a resource method, you can use the @Produces
annotation with the desired media type.
@GET
@Produces("application/custom-format")
public Response getCustomData() {
CustomFormat customFormat = // Retrieve or create the CustomFormat object
return Response.ok(customFormat).build();
}
By specifying the appropriate @Consumes
and @Produces
annotations, the JAX-RS runtime uses the custom message body readers and writers to handle the serialization and deserialization of custom content.
In Conclusion, Here is What Matters
In this article, we explored the process of implementing custom content handling in JAX-RS 2.0 using message body readers and writers. By creating custom providers for specific content types, developers can seamlessly integrate proprietary data formats into their JAX-RS applications.
Understanding and implementing custom content handling in JAX-RS opens up new possibilities for building robust and flexible web services that cater to diverse data requirements.
By following the principles outlined in this article, you can enhance your JAX-RS applications with support for custom content types, paving the way for enhanced interoperability and compatibility.
For further reading on JAX-RS and custom content handling, you may find the official Java EE JAX-RS documentation and the Java API for JSON Processing (JSON-P) valuable resources.
As you continue to explore the world of web services in Java, incorporating custom content handling will undoubtedly expand your arsenal of capabilities, empowering you to tackle a wide range of data formats with confidence.
Checkout our other articles