Resolving Cross-Browser Compatibility Woes
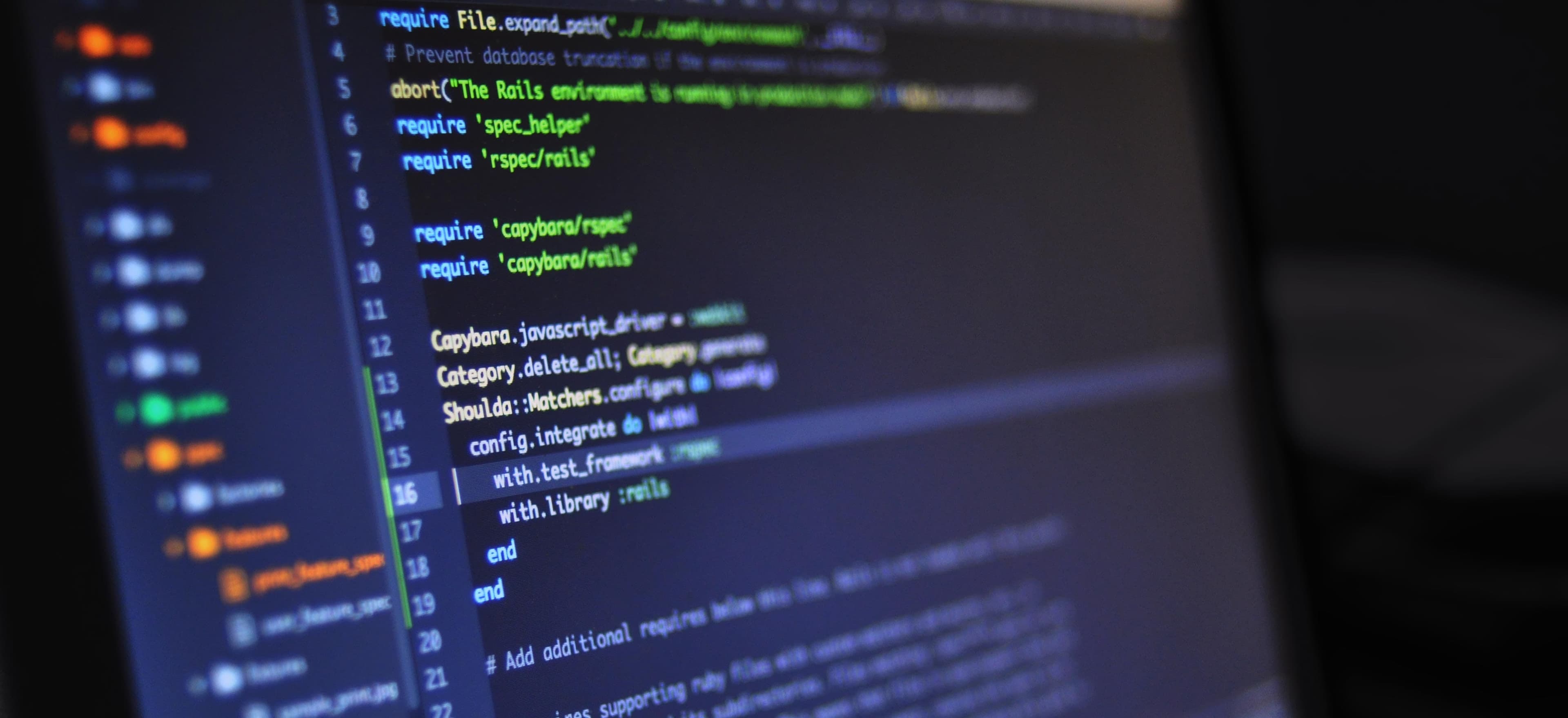
- Published on
Resolving Cross-Browser Compatibility Woes
When developing web applications, ensuring cross-browser compatibility is paramount. Users access websites and web applications using a wide array of browsers such as Chrome, Firefox, Safari, and Edge, each with its own quirks and features. One of the most significant challenges faced by developers is ensuring that their code works seamlessly across all these platforms. In this post, we will explore how Java can be leveraged to tackle cross-browser compatibility issues and provide tips to streamline the process.
Understanding the Challenge
Different browsers interpret code in slightly different ways, which can lead to inconsistencies in the appearance and functionality of web applications. This can manifest in various forms, such as layout discrepancies, styling issues, or even functional impairments. The disparity in rendering engines across browsers further complicates matters, making it essential to write code that is robust and compatible with a variety of environments.
Leveraging Java to Enhance Compatibility
Java, renowned for its versatility and cross-platform capabilities, can be a valuable asset in addressing cross-browser compatibility issues. By employing Java for server-side scripting and leveraging its robust libraries, developers can create web applications that are more resilient to the nuances of different browsers. Let's delve into some strategies for utilizing Java to enhance cross-browser compatibility.
1. Data Handling and Formatting
Java excels in data handling and formatting, offering a comprehensive range of methods and libraries for parsing, manipulating, and presenting data. By performing intricate data formatting operations on the server side with Java, developers can minimize the reliance on client-side scripting, thereby mitigating potential discrepancies arising from browser-specific interpretations.
// Example of data formatting in Java
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd");
Date date = new Date();
String formattedDate = sdf.format(date);
In the above code snippet, Java's SimpleDateFormat class is utilized to format a date, showcasing how server-side data formatting can alleviate cross-browser compatibility concerns.
2. Standardized HTML Generation
Java's server-side capabilities enable the generation of standardized HTML, ensuring consistency across browsers. By leveraging Java's robust libraries and frameworks such as Thymeleaf or Apache Wicket, developers can construct HTML templates that adhere to best practices and are less susceptible to rendering discrepancies on different browsers.
// Example using Thymeleaf to generate HTML
model.addAttribute("pageTitle", "Welcome");
return "index";
Here, the Thymeleaf framework is employed to dynamically generate HTML content, illustrating how Java can facilitate the creation of browser-agnostic markup.
3. Comprehensive Testing with Selenium
Selenium, a widely-used automated testing framework, can be seamlessly integrated with Java to conduct rigorous cross-browser testing. By crafting tests in Java with Selenium, developers can systematically verify the behavior and functionality of their web applications across multiple browsers, pinpointing and rectifying compatibility issues effectively.
// Example of using Selenium in Java for cross-browser testing
WebDriver driver = new ChromeDriver();
driver.get("https://www.example.com");
WebElement element = driver.findElement(By.name("username"));
element.sendKeys("example");
The above code snippet showcases the use of Selenium in Java to automate interactions with a web page, underscoring its utility in ensuring cross-browser compatibility through comprehensive testing.
Tips for Streamlining Cross-Browser Compatibility
In addition to leveraging Java's capabilities, adopting best practices and streamlined approaches can further fortify cross-browser compatibility. Here are some tips to streamline the process:
1. Embrace Progressive Enhancement
Adopting a progressive enhancement approach entails focusing on core functionality and then layering advanced features for browsers that support them. By initially prioritizing functionality that is universally supported, developers can mitigate the impact of browser-specific disparities and bolster the overall compatibility of their web applications.
2. Regularly Update Browser Support Policies
Staying abreast of browser updates and revisiting support policies are imperative. By monitoring browser trends and revising support policies accordingly, developers can adapt their code to align with the evolving landscape of web browsers, preempting compatibility issues before they arise.
3. Leverage CSS Resets and Vendor Prefixes
Employing CSS resets and vendor prefixes judiciously can rectify styling inconsistencies across browsers. By utilizing established CSS resets and accommodating vendor prefixes for CSS properties, developers can promote uniformity in styling and circumvent rendering disparities.
The Last Word
Navigating the intricate terrain of cross-browser compatibility demands a combination of astute coding practices, leveraging robust technologies, and embracing adaptive strategies. Java, with its versatile capabilities and steadfast performance, can be harnessed to fortify web applications against the vagaries of different browsers. By amalgamating Java's prowess with meticulous testing, streamlined approaches, and a penchant for adaptability, developers can surmount cross-browser compatibility woes, ensuring that their web applications resonate harmoniously across diverse browsing environments.
Considering the ever-evolving nature of web technologies and browser landscape, the pursuit of seamless cross-browser compatibility remains a perpetual endeavor, underpinning the importance of continuous refinement and vigilance in the realm of web development.
In conclusion, by incorporating Java's strengths and adhering to best practices, developers can chart a robust course towards harmonious cross-browser compatibility, underlining the invaluable role of Java in fortifying web applications against the perils of disparate browser interpretations.
Remember, the quest for cross-browser compatibility is an ongoing odyssey, and with Java as a stalwart ally, the journey becomes not only navigable but also gratifying.
Checkout our other articles