Overcoming Challenges in Microservices Service-to-Service Communication
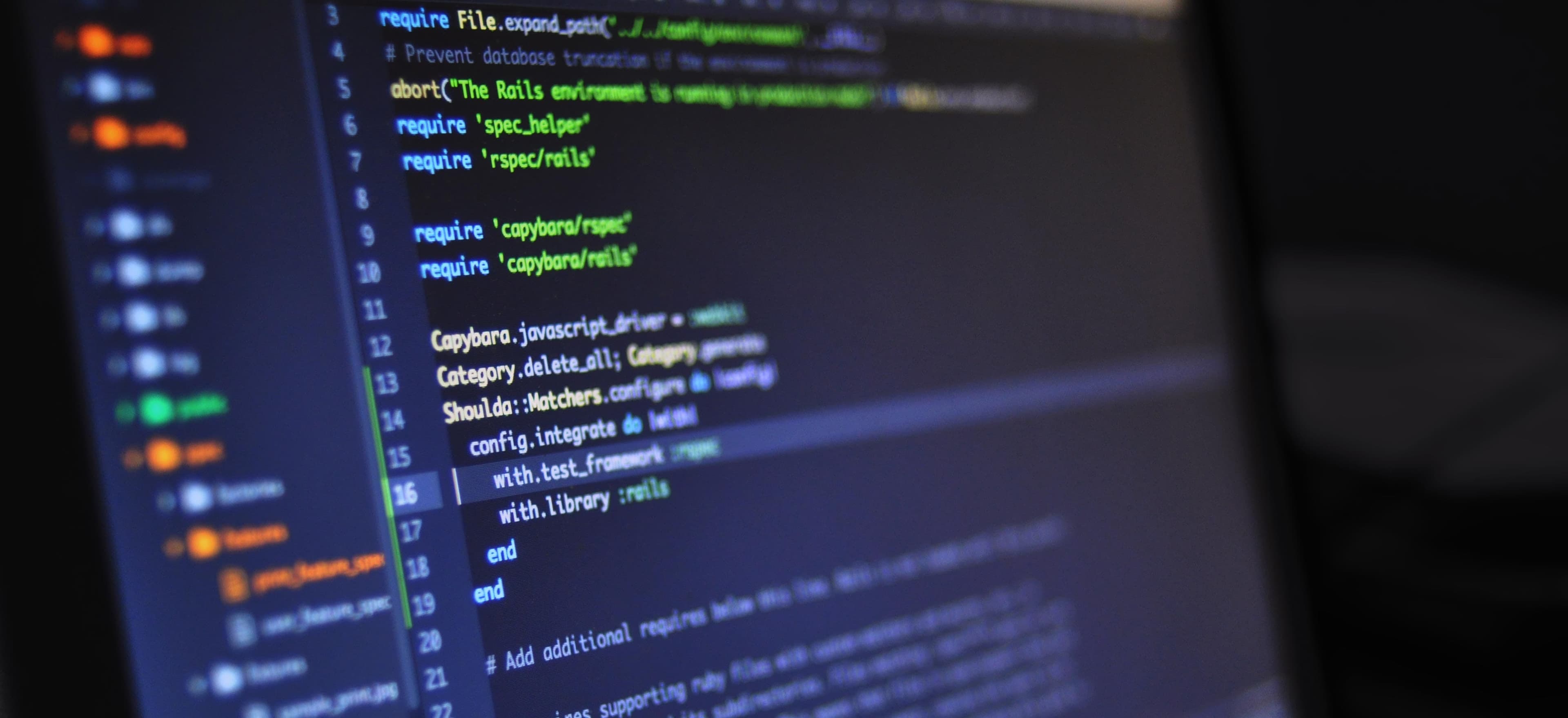
- Published on
Overcoming Challenges in Microservices Service-to-Service Communication
Microservices architecture has gained popularity due to its ability to break down complex applications into smaller, manageable services. However, along with its benefits come challenges, one of which is service-to-service communication. In this post, we will explore the challenges faced in microservices service-to-service communication and how to overcome them using Java.
1. Asynchronous Communication
In a microservices environment, services often need to communicate with each other asynchronously. This can lead to challenges in managing communication between services, especially when dealing with high latency or unreliable networks.
Using Message Brokers
One way to overcome this challenge is to use message brokers such as Apache Kafka or RabbitMQ. These message brokers enable asynchronous communication by allowing services to publish messages to topics or queues.
Java Code Example (Using Apache Kafka)
// Producer
Properties props = new Properties();
props.put("bootstrap.servers", "localhost:9092");
props.put("key.serializer", "org.apache.kafka.common.serialization.StringSerializer");
props.put("value.serializer", "org.apache.kafka.common.serialization.StringSerializer");
Producer<String, String> producer = new KafkaProducer<>(props);
ProducerRecord<String, String> record = new ProducerRecord<>("topicName", "key", "value");
producer.send(record);
// Consumer
Properties props = new Properties();
props.put("bootstrap.servers", "localhost:9092");
props.put("group.id", "test-group");
props.put("key.deserializer", "org.apache.kafka.common.serialization.StringDeserializer");
props.put("value.deserializer", "org.apache.kafka.common.serialization.StringDeserializer");
Consumer<String, String> consumer = new KafkaConsumer<>(props);
consumer.subscribe(Collections.singletonList("topicName"));
ConsumerRecords<String, String> records = consumer.poll(Duration.ofMillis(100));
By using a message broker like Apache Kafka, services can communicate asynchronously without depending on the availability of other services.
2. Resilience and Fault Tolerance
In a microservices architecture, services need to be resilient to failures and have built-in fault tolerance. When one service is down or experiencing high latency, it should not adversely affect the overall system.
Circuit Breaker Pattern
To address this challenge, the Circuit Breaker pattern can be implemented. Libraries like Netflix Hystrix provide support for implementing the Circuit Breaker pattern in Java.
Java Code Example (Using Netflix Hystrix)
@HystrixCommand(fallbackMethod = "fallbackMethod")
public String remoteServiceCall() {
// Make remote service call
}
public String fallbackMethod() {
// Fallback logic
}
With the Circuit Breaker pattern, services can isolate failures and prevent cascading failures across the system.
3. Service Discovery and Load Balancing
In a microservices environment, services are dynamically added or removed, making it challenging to keep track of their locations and manage their load.
Using Service Registry and Client-Side Load Balancing
Service registry solutions like Netflix Eureka and load balancers like Ribbon can help in addressing this challenge.
Java Code Example (Using Netflix Eureka and Ribbon)
// Service Registration (Using Eureka)
@EnableEurekaClient
@SpringBootApplication
public class ServiceApplication {
public static void main(String[] args) {
SpringApplication.run(ServiceApplication.class, args);
}
}
// Service Discovery and Load Balancing (Using Ribbon)
@FeignClient(name = "service-application")
public interface ServiceClient {
@RequestMapping(method = RequestMethod.GET, value = "/endpoint")
String callService();
}
By integrating Netflix Eureka and Ribbon, services can discover and communicate with each other using client-side load balancing.
A Final Look
Microservices service-to-service communication poses several challenges, including asynchronous communication, resilience, fault tolerance, and service discovery. By leveraging Java and relevant libraries and tools, such as Apache Kafka, Netflix Hystrix, Netflix Eureka, and Ribbon, these challenges can be effectively addressed, enabling robust and efficient communication between microservices.
In conclusion, mastering service-to-service communication is crucial for the success of microservices architecture, and Java has robust tools and patterns to help overcome these challenges.
For more in-depth insights and practical examples, refer to books like "Microservices Patterns" by Chris Richardson and "Release It!" by Michael T. Nygard.
With the right approach and tools, overcoming the challenges in microservices service-to-service communication is not only achievable but can also lead to a highly resilient and responsive system. Happy coding!
This article has been brought to you by Java Online Learning, where you can master the art of Java programming and microservices architecture.