Reactive Queue Durability: Ensuring Reliable Akka Persistence
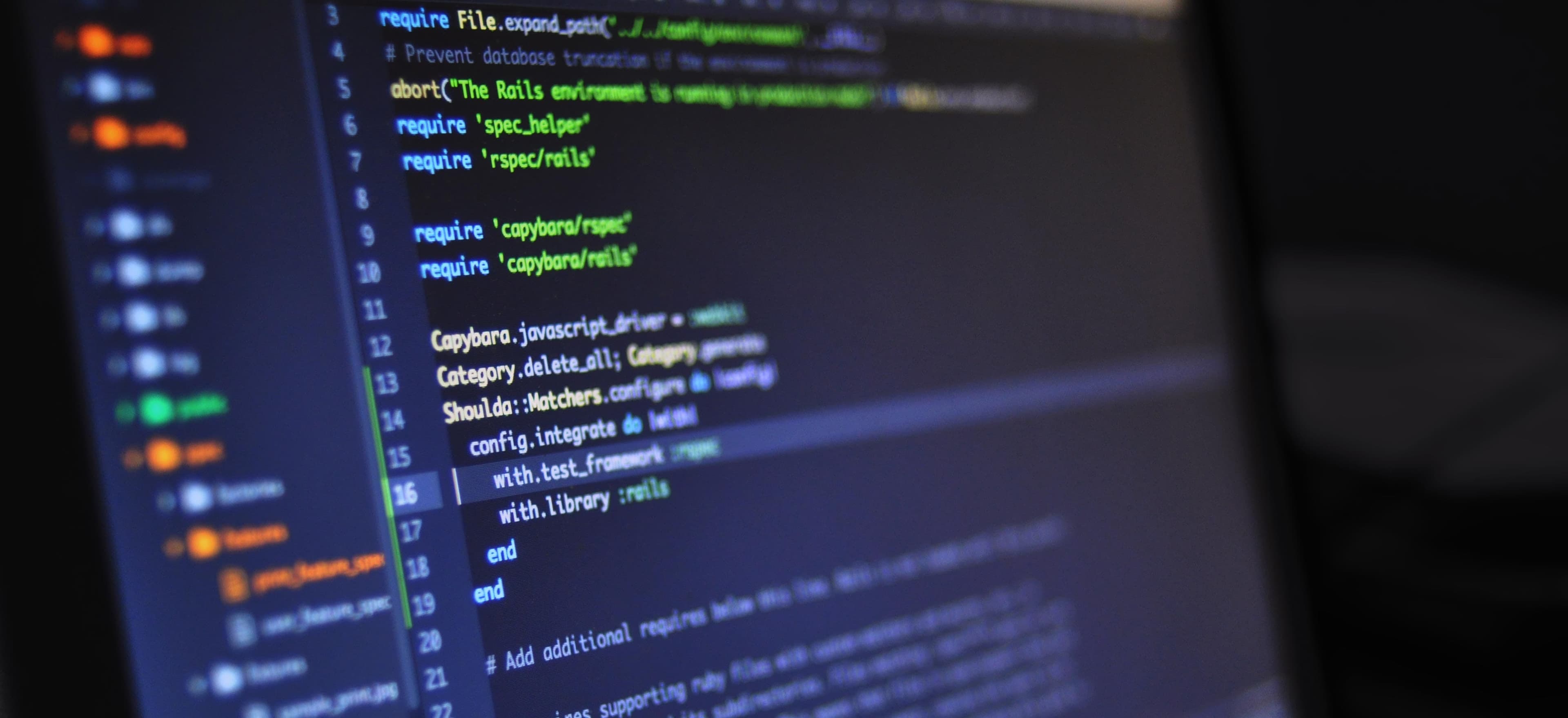
- Published on
Reactive Queue Durability: Ensuring Reliable Akka Persistence
In the realm of reactive programming, Akka has emerged as a powerful toolkit for building scalable and resilient applications. At the heart of Akka lies its persistence module, which allows actors to persist their state, enabling the construction of resilient systems.
One crucial aspect of persistence is ensuring the durability of the message queue. In this blog post, we will explore the significance of queue durability in Akka persistence and how it contributes to building reliable and fault-tolerant systems.
Why Queue Durability Matters
In a distributed system built with Akka, the message queue serves as a critical component for ensuring reliable communication between actors. If the message queue lacks durability, there is a risk of losing messages in the event of system failures or crashes.
By focusing on queue durability, we can guarantee that messages are not lost and that the system can recover from failures without compromising the integrity of the data flow. This is particularly important in scenarios where the processing of messages has side effects, and losing messages could lead to inconsistencies in the system state.
Using Akka Persistence for Queue Durability
Akka Persistence provides a robust mechanism for achieving queue durability by persisting messages to a journal. The journal acts as the source of truth for the state of persistent actors, storing all the messages that have been processed. This allows the system to reconstruct the state of actors in case of failures or restarts.
Let's take a look at an example of how Akka Persistence ensures queue durability. Consider the following code snippet:
public class ExamplePersistentActor extends AbstractPersistentActor {
private List<Object> events = new ArrayList<>();
@Override
public String persistenceId() {
return "example-actor-id";
}
@Override
public Receive createReceive() {
return receiveBuilder()
.match(String.class, s -> {
persist(s, event -> {
events.add(event);
// Handle the persisted event
});
})
.build();
}
}
In this example, persist()
is used to save the incoming message to the journal, ensuring that it is durably stored. The events
list maintains the state of the actor by keeping track of all the persisted events. In case of a crash, Akka Persistence will replay the events from the journal, allowing the actor to reconstruct its state reliably.
Guarantees Provided by Akka Persistence
Akka Persistence offers several guarantees that contribute to queue durability:
At-Least-Once Message Delivery
Akka Persistence ensures that messages are delivered to the journal at least once. This prevents message loss even in the face of network partitions or transient failures.
Idempotent Recovery
The recovery process in Akka Persistence is designed to be idempotent, meaning that replaying the same set of events multiple times produces the same result. This property ensures that the state of persistent actors remains consistent, even when recovering from failures.
Crash Resilience
In the event of actor crashes or system failures, Akka Persistence facilitates the recovery of actor state by replaying the journaled events. This resilience is fundamental to maintaining queue durability in the face of unexpected disruptions.
Strategies for Optimizing Queue Durability
While Akka Persistence provides robust mechanisms for ensuring queue durability, there are additional strategies that can be employed to optimize this aspect further.
Configuring Journal Plugins
Akka Persistence allows for the use of various journal plugins, such as Cassandra or JDBC, each offering different capabilities for storing and retrieving journaled messages. By selecting the appropriate journal plugin based on the specific requirements of the application, it is possible to enhance the durability of the message queue.
Tuning Write Batch Sizes
Adjusting the write batch sizes can have a significant impact on the durability of the message queue. By configuring the size of write batches, it is possible to optimize the throughput of messages being persisted, thereby improving the overall durability of the system.
Leveraging Event Sourcing
Event sourcing, a fundamental concept in Akka Persistence, involves storing the state of an actor as a sequence of events. By leveraging event sourcing, it is possible to ensure a comprehensive log of all state transitions, enhancing the durability of the actor's state and the overall message queue.
Wrapping Up
In the world of reactive programming, ensuring the durability of the message queue is pivotal to building reliable and fault-tolerant systems. With Akka Persistence, developers can leverage robust mechanisms for persisting messages to a journal, thereby guaranteeing the durability of the message queue and enabling the construction of resilient applications.
By understanding the significance of queue durability and employing strategies to optimize it, developers can harness the full potential of Akka Persistence in creating scalable, reliable, and fault-tolerant systems within the realm of reactive programming.
In conclusion, queue durability in Akka Persistence is not just a technical detail; it is a cornerstone for building systems that can withstand the challenges of distributed environments and deliver consistent and reliable behavior.
By focusing on the importance of queue durability in Akka Persistence, this blog post has shed light on the critical role it plays in building resilient systems. We've explored how Akka Persistence ensures queue durability and discussed strategies for optimizing it, highlighting the significance of this aspect in the realm of reactive programming. If you're interested in diving deeper into Akka Persistence, be sure to check out the official documentation for comprehensive insights.