Efficiently Wiring YAML Data with Chronicle Wire
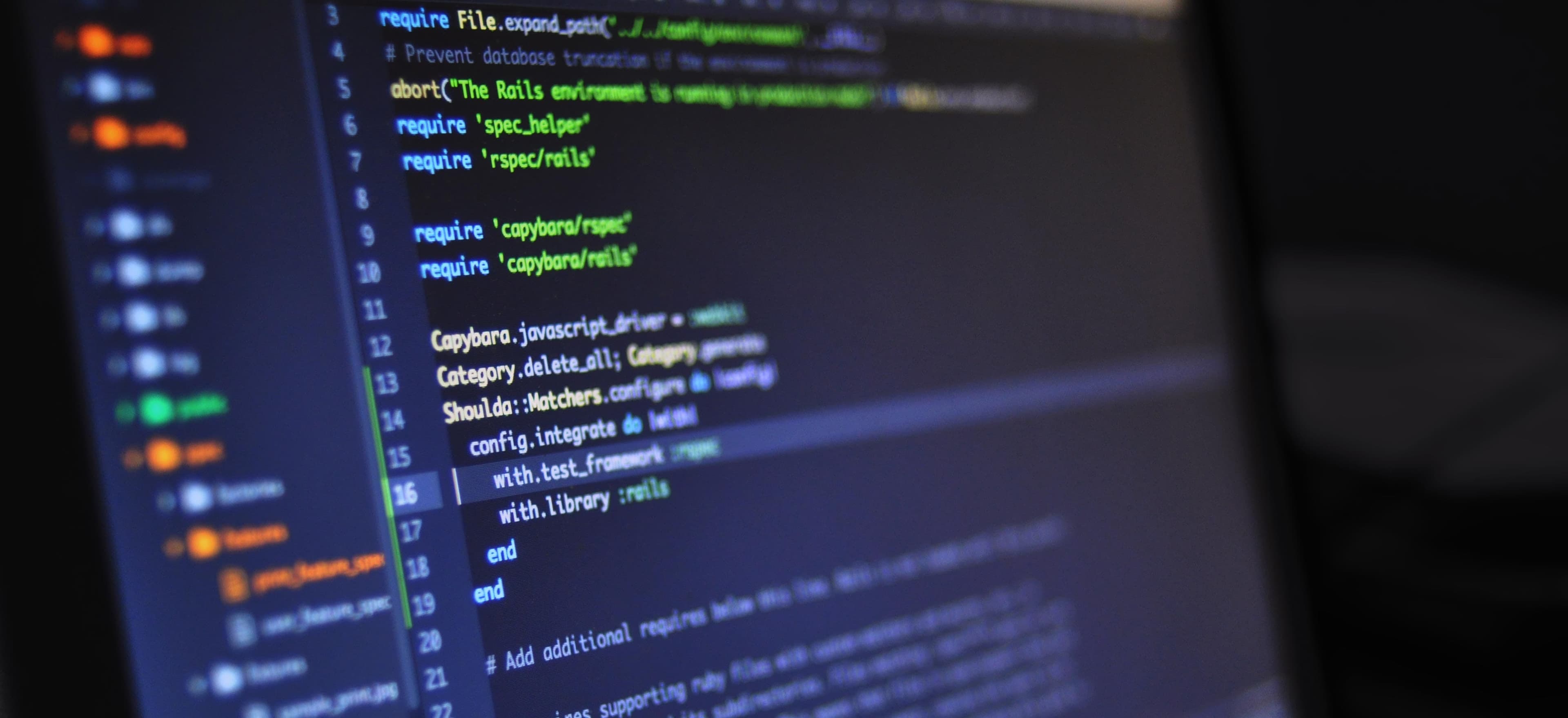
- Published on
Efficiently Wiring YAML Data with Chronicle Wire
When it comes to working with data in Java, efficiency and ease of use are crucial. In the world of financial services and low-latency trading systems, these aspects are even more important. This is where Chronicle Wire, a library designed for high-speed message marshaling and data transfer, comes into play. In this post, we'll explore how Chronicle Wire can be used to efficiently wire YAML data and discuss the benefits it brings to Java developers.
What is Chronicle Wire?
Chronicle Wire is a component of the Chronicle library that focuses on efficient data transfer and serialization. It is designed to outperform other serialization frameworks while providing a simple and flexible API for developers.
One of the key features of Chronicle Wire is its ability to serialize and deserialize data with minimal overhead. This is achieved by using a binary format that is optimized for speed and efficiency. Additionally, Chronicle Wire supports a variety of data formats, including YAML, JSON, and binary.
Wiring YAML Data
YAML is a popular human-readable data serialization format that is commonly used for configuration files and data exchange. Chronicle Wire provides a straightforward way to wire YAML data in Java, allowing developers to take advantage of YAML's readability and simplicity while benefiting from Chronicle Wire's efficiency.
Example: Wiring YAML Data
Let's consider an example where we have a YAML configuration file that contains settings for a trading system.
import net.openhft.chronicle.wire.DocumentContext;
import net.openhft.chronicle.wire.Wire;
public class YAMLWiringExample {
public static void main(String[] args) {
Wire wire = new TextWire(Bytes.elasticByteBuffer());
try (DocumentContext dc = wire.writeingDocument()) {
dc.wire().getValueOut().leafNode("tradingSystem")
.marshallable(m -> m.write("name").text("Chronicle Trading System")
.write("version").int32(1)
.write("enabled").bool(true));
}
System.out.println(wire);
}
}
In this example, we create a Wire
instance and use it to write YAML data. We start by opening a DocumentContext
to specify the boundaries of the document. Then, we use the getValueOut()
method to start wiring our YAML data. We write the trading system's name, version, and enabled status using the fluent API provided by Chronicle Wire.
Why Use Chronicle Wire for YAML Data Wiring?
- Efficiency: Chronicle Wire's binary format and low overhead make it more efficient than traditional text-based serialization formats like YAML.
- Flexibility: Chronicle Wire supports various data formats, allowing developers to choose the most suitable format for their use case. This flexibility extends to the integration of YAML data within Java applications.
- Performance: The low-latency design of Chronicle Wire makes it well suited for high-performance applications, such as trading systems, where every microsecond counts.
Benefits of Using Chronicle Wire for YAML Data
Now that we've seen how Chronicle Wire can be used to efficiently wire YAML data, let's explore the benefits it brings to Java developers.
High Performance
Chronicle Wire is built for high-speed data transfer, making it an ideal choice for applications that require low-latency and high throughput. By using Chronicle Wire to wire YAML data, developers can ensure that their applications perform optimally, especially in scenarios where speed is paramount, such as in financial trading systems.
Seamless Integration
With Chronicle Wire, integrating YAML data into Java applications is straightforward and requires minimal effort. The API provided by Chronicle Wire allows developers to work with YAML data in a natural and intuitive way, without having to deal with the complexities of low-level serialization.
Reduced Overhead
Traditional YAML parsers often come with a significant overhead in terms of memory allocation and CPU usage. Chronicle Wire's binary format significantly reduces this overhead, resulting in improved efficiency and reduced resource consumption.
Robustness and Readability
While Chronicle Wire's binary format offers exceptional performance, its support for YAML maintains the human-readable and intuitive nature of the format. This means that developers can continue working with YAML data in a familiar way while benefiting from Chronicle Wire's performance advantages.
Closing the Chapter
In the fast-paced world of Java development, the efficient wiring and transfer of data are essential. Chronicle Wire addresses these needs by offering a high-performance, flexible, and easy-to-use solution for working with data, including YAML. By utilizing Chronicle Wire for wiring YAML data, developers can achieve the optimal balance of efficiency and readability, making it a valuable addition to their toolkit.
So, whether you're building a low-latency trading system or handling high-volume data transfers, consider leveraging Chronicle Wire for efficient data wiring and serialization, and experience the benefits it brings to your Java applications.