Optimizing Docker Compose for Spring Boot and PostgreSQL
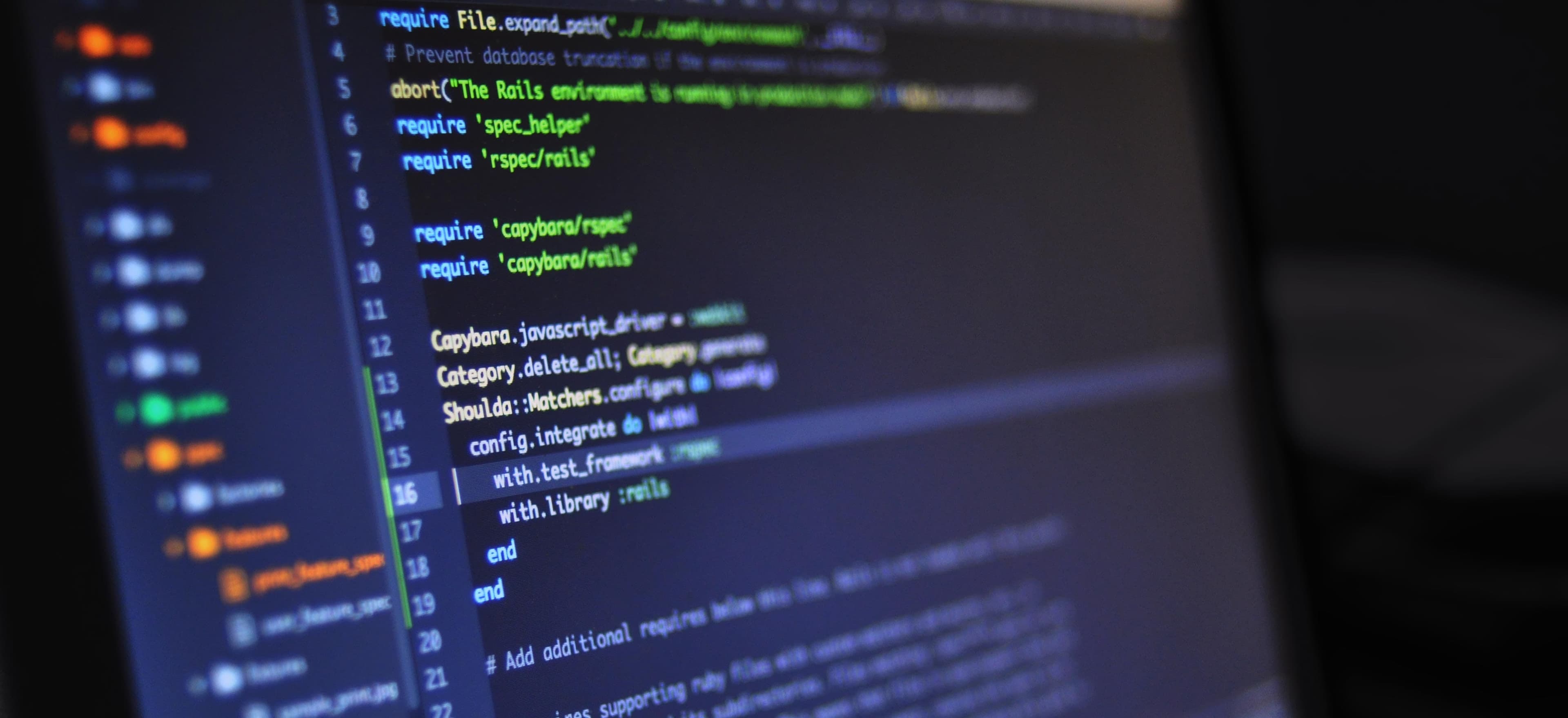
- Published on
Optimizing Docker Compose for Spring Boot and PostgreSQL
Docker Compose is a powerful tool for defining and running multi-container Docker applications. When working with a Spring Boot application backed by a PostgreSQL database, optimizing the Docker Compose configuration becomes crucial for achieving efficient development and deployment workflows. In this article, we will explore various strategies to optimize Docker Compose for a Spring Boot and PostgreSQL setup, ensuring scalability, performance, and ease of use.
Setting Up the Docker Compose Configuration
Let's start by defining the Docker Compose setup for our Spring Boot application and PostgreSQL database. We'll create a docker-compose.yml
file to configure the required services, network settings, and volumes.
version: '3.8'
services:
app:
build:
context: .
dockerfile: Dockerfile
ports:
- "8080:8080"
depends_on:
- db
environment:
SPRING_DATASOURCE_URL: jdbc:postgresql://db:5432/dbname
SPRING_DATASOURCE_USERNAME: username
SPRING_DATASOURCE_PASSWORD: password
db:
image: postgres:latest
environment:
POSTGRES_DB: dbname
POSTGRES_USER: username
POSTGRES_PASSWORD: password
volumes:
- postgres-data:/var/lib/postgresql/data
volumes:
postgres-data:
In this configuration, we have defined two services: app
for our Spring Boot application and db
for the PostgreSQL database. We have also specified the environment variables required for the Spring Boot application to connect to the PostgreSQL database.
Optimizing Network Communication
To optimize the network communication between the Spring Boot application and the PostgreSQL database, we can utilize Docker's internal networking capabilities. By default, Docker Compose creates a bridge network for our services, allowing them to communicate with each other using their service names as hostnames.
version: '3.8'
services:
app:
...
depends_on:
- db
environment:
SPRING_DATASOURCE_URL: jdbc:postgresql://db:5432/dbname
networks:
- backend
db:
...
networks:
- backend
networks:
backend:
By defining a custom network and attaching both services to it, we can isolate their communication from other containers and reduce unnecessary network overhead.
Managing Volumes for Data Persistence
Data persistence is a critical aspect of any database-driven application. Docker volumes provide a convenient way to persist data across container restarts and ensure data integrity.
version: '3.8'
services:
app:
...
volumes:
- app-data:/path/to/app/data
db:
...
volumes:
- db-data:/var/lib/postgresql/data
volumes:
app-data:
db-data:
By defining named volumes for both the Spring Boot application and the PostgreSQL database, we can ensure that their respective data remains persistent even if the containers are stopped or recreated.
Optimizing Container Build and Image Size
Efficient container builds and small image sizes are essential for fast deployment and reduced resource consumption. We can optimize our Dockerfile to achieve this goal.
# Use a lightweight base image
FROM openjdk:11-jre-slim
# Set the working directory
WORKDIR /app
# Copy the JAR file into the container
COPY target/your-application.jar app.jar
# Set the entrypoint
ENTRYPOINT ["java", "-jar", "app.jar"]
In this Dockerfile, we use a lightweight Java runtime environment as the base image and only copy the compiled JAR file into the container. This approach reduces the image size and minimizes the build time.
Leveraging Docker Compose Profiles (Optional)
Docker Compose profiles provide a way to streamline the development, testing, and production configurations of our services. We can define custom profiles in our docker-compose.yml
file to manage environment-specific settings and dependencies.
version: '3.8'
services:
app:
...
profiles:
- dev
- prod
db:
...
profiles:
- dev
- prod
By utilizing profiles, we can easily switch between different configurations and ensure consistency across environments.
In Conclusion, Here is What Matters
Optimizing Docker Compose for a Spring Boot and PostgreSQL application involves fine-tuning various aspects of the configuration, including network communication, data persistence, container build, and image size. By following the strategies outlined in this article, we can ensure a streamlined and efficient development and deployment process for our containerized application.
In summary, we have explored optimizing network communication, managing volumes for data persistence, reducing container build and image size, and leveraging Docker Compose profiles. These optimizations contribute to a robust and scalable Docker Compose setup for Spring Boot and PostgreSQL applications.
By implementing these best practices, developers can benefit from a well-organized and performance-optimized Docker Compose setup, laying the foundation for seamless deployment and management of Spring Boot applications with PostgreSQL databases.
For more in-depth information on Docker Compose and best practices for Spring Boot applications, check out the Docker documentation and Spring Boot guides.