Lagging Data Streams in Lightstreamer Flutter Plugin
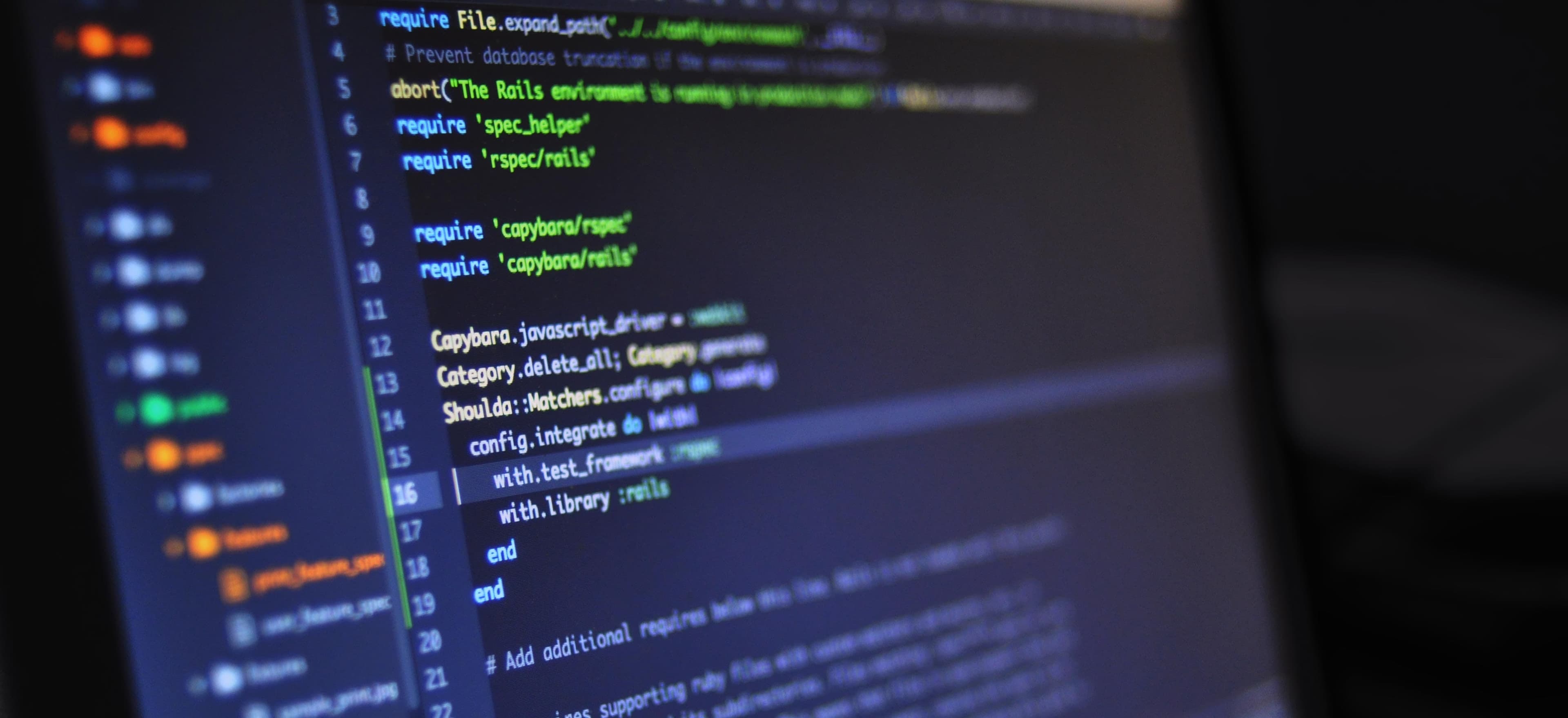
- Published on
Optimizing Data Streams in Lightstreamer Flutter Plugin
When it comes to real-time data streaming in a Flutter application, developers often encounter challenges related to performance and responsiveness. One common issue is lagging data streams, which can negatively impact the user experience. To address this problem, it's important to optimize the data streaming process by leveraging efficient techniques and tools.
In this blog post, we will explore how to optimize data streams in a Flutter application using the Lightstreamer Flutter plugin. We will delve into techniques for improving data streaming performance, eliminating lag, and enhancing the overall user experience.
Understanding the Problem
Before delving into optimization techniques, let's first understand the root causes of lagging data streams in a Flutter application. Several factors can contribute to this issue, including inefficient data processing, network latency, and suboptimal implementation of data streaming libraries.
When using the Lightstreamer Flutter plugin, it's crucial to ensure that the data streaming process is optimized to deliver real-time updates seamlessly. This involves fine-tuning the configuration, implementing best practices, and leveraging advanced features provided by the plugin.
Optimizing Data Streams with Lightstreamer
1. Efficient Configuration Settings
One of the first steps in optimizing data streams is configuring the Lightstreamer client with optimal settings. This includes defining the appropriate connection protocol, heartbeat interval, and other parameters that directly impact the data streaming performance.
lsClient.connectionOptions.setRequestedMaxBandwidth("unlimited");
lsClient.connectionOptions.setPollingInterval(100);
lsClient.connectionOptions.setSlowingEnabled(false);
In the above code snippet, we have set the maximum bandwidth to "unlimited" and disabled slowing, ensuring that the client can receive updates without any artificial limitations. Additionally, we have set the polling interval to 100 milliseconds, allowing for more frequent updates.
2. Streamlining Data Update Handling
Efficiently handling data updates is crucial for minimizing lag in real-time data streaming. By employing optimized data processing techniques, such as using dedicated isolates or compute functions for heavy data processing tasks, developers can ensure that updates are processed swiftly without impacting the UI thread.
streamSubscription = lsSubscription.getDataStream().listen((update) {
// Process the incoming update using isolates or compute functions
// Update the UI with the processed data
});
In the above code snippet, we utilize a data stream subscription to listen for incoming updates. Within the listener, developers can implement efficient data processing mechanisms to handle updates without causing UI lag.
3. Implementing Connection Resilience
To mitigate the impact of network fluctuations on data streaming, it's essential to implement connection resilience mechanisms. This involves handling network disruptions, re-establishing connections, and resuming data streaming seamlessly.
lsClient.addListener(new ConnectionListener() {
@override
public void onConnectionInterrupted() {
// Handle connection interruption
}
@override
public void onConnectionResumed() {
// Handle connection resumption
}
});
By implementing connection listeners and appropriate error-handling logic, developers can ensure that the Flutter application remains resilient to network disturbances, thereby minimizing the impact of lag on data streams.
The Bottom Line
In conclusion, optimizing data streams in a Flutter application using the Lightstreamer plugin is essential for delivering a smooth and responsive real-time data streaming experience. By configuring the client efficiently, streamlining data update handling, and implementing connection resilience, developers can effectively address lagging data streams and enhance the overall user experience.
For further insights into real-time data streaming and optimization techniques, explore the official documentation and engage with the vibrant developer community to exchange best practices and solutions.
Optimizing data streams is not just a technical endeavor but also a crucial aspect of delivering a high-quality user experience in real-time data-driven applications. By following these optimization techniques, developers can ensure that their Flutter applications powered by the Lightstreamer plugin perform optimally, even under demanding real-time data streaming scenarios.