Optimizing Serverless Architecture for Efficient Workloads
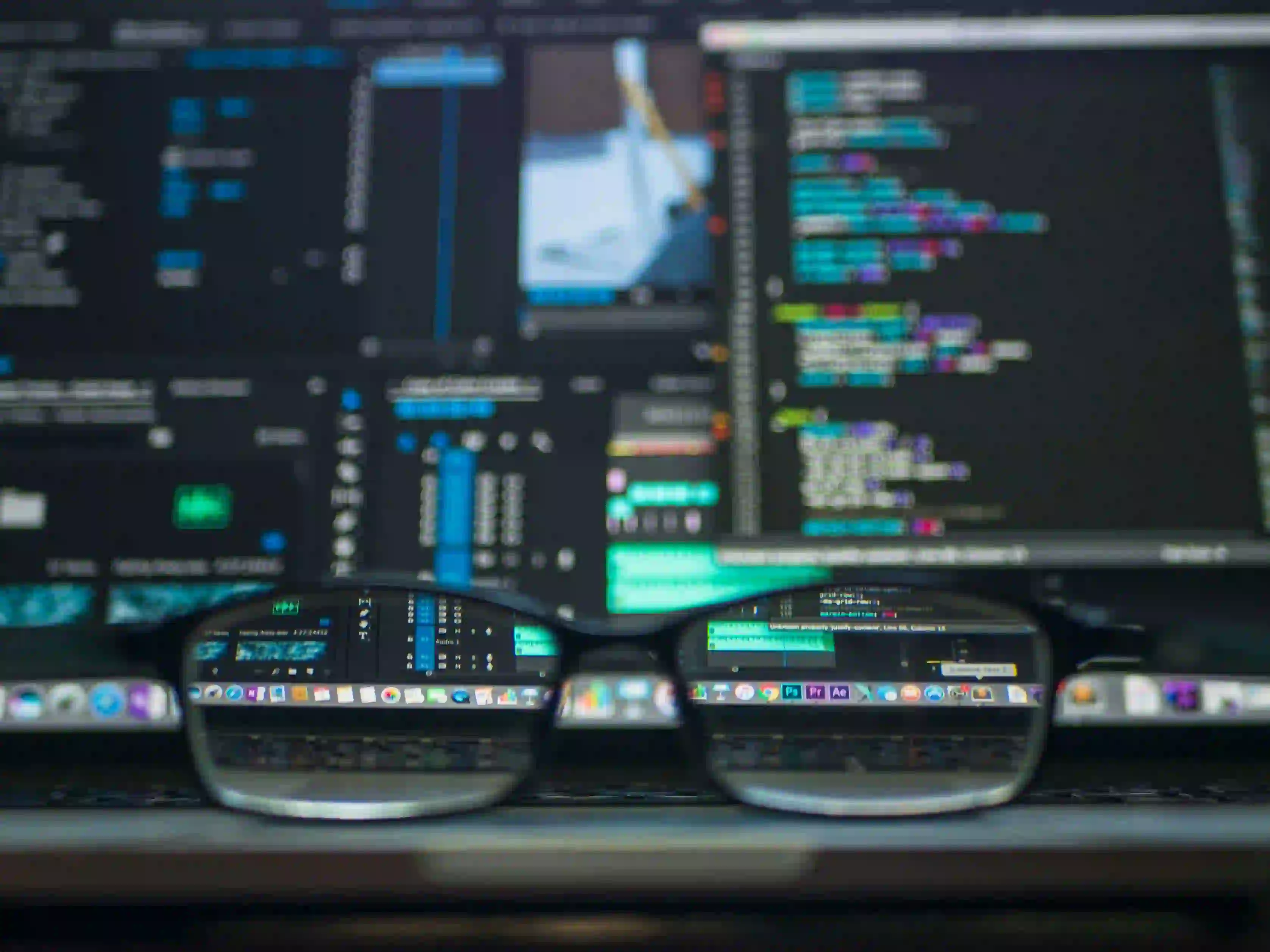
Improving Workload Efficiency in Serverless Architecture with Java
Serverless architecture has gained popularity for its cost-efficiency and scalability. However, to make the most of serverless, it's crucial to optimize the workloads. In this article, we will explore how to optimize serverless architecture for efficient workloads using Java.
Understanding Serverless Workloads
Serverless architecture allows developers to focus on writing code without worrying about server management. Workloads in a serverless environment are typically broken down into small, focused functions that execute in response to events.
Leveraging Java for Serverless
Java is a popular choice for serverless development due to its strong ecosystem and performance. With Java, developers can build robust, scalable functions that seamlessly integrate with serverless platforms like AWS Lambda, Azure Functions, and Google Cloud Functions.
Key Considerations for Workload Efficiency
Minimizing Function Cold Starts
Cold starts occur when a function is invoked for the first time or after a prolonged period of inactivity. These can impact workload efficiency by introducing latency. To mitigate cold starts, it's essential to optimize Java functions for faster initialization.
Efficient Memory Management
Memory allocation can significantly impact the performance of serverless functions. By optimizing memory usage, developers can reduce costs and improve the overall efficiency of workloads. Java provides several memory management tools and techniques that can be utilized for this purpose.
Streamlining Dependencies
Java projects often rely on third-party dependencies. While these libraries can enhance functionality, they can also increase function size and initialization time. It's imperative to carefully evaluate and streamline dependencies to ensure optimal workload efficiency.
Optimizing Java Functions for Serverless Efficiency
Minimizing Cold Starts with GraalVM
GraalVM is a powerful tool for optimizing Java applications for serverless environments. By compiling Java functions ahead of time into native executables, GraalVM mitigates cold starts and improves overall performance.
public class HelloFunction implements RequestHandler<String, String> {
@Override
public String handleRequest(String input, Context context) {
return "Hello, " + input;
}
}
By using GraalVM, we can transform our Java functions into native images, reducing startup time and enhancing workload efficiency.
Memory Management with Java G1 Garbage Collector
The Garbage-First (G1) Garbage Collector in Java enables efficient memory management for serverless functions. By tuning G1 GC settings, developers can optimize memory allocation and reduce the impact of garbage collection on function performance.
-XX:+UseG1GC
-XX:G1HeapRegionSize=4M
-XX:MaxGCPauseMillis=50
Configuring the G1 GC parameters allows for fine-tuning memory management in serverless Java functions.
Dependency Optimization with Maven
Maven is a popular build automation tool for Java projects. By analyzing and optimizing dependencies using tools like the Maven Dependency Plugin, developers can efficiently manage library usage and minimize the footprint of serverless functions.
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-dependency-plugin</artifactId>
<executions>
<execution>
<id>analyze</id>
<phase>prepare-package</phase>
<goals>
<goal>analyze</goal>
</goals>
</execution>
</executions>
</plugin>
Integrating the Maven Dependency Plugin into the build process helps identify and eliminate unnecessary dependencies, improving the efficiency of serverless workloads.
In Conclusion, Here is What Matters
Optimizing serverless workloads for efficiency is essential for maximizing cost savings and performance. By leveraging Java's capabilities and integrating optimization techniques such as GraalVM, G1 GC, and dependency management, developers can ensure that their serverless functions operate at peak efficiency within a serverless architecture.
In summary, Java's versatility and robust tooling make it well-suited for achieving efficient workloads in a serverless environment. By implementing the discussed strategies, developers can unlock the full potential of serverless architecture while delivering high-performance, cost-effective solutions.