Mastering Explicit and Implicit Conversions
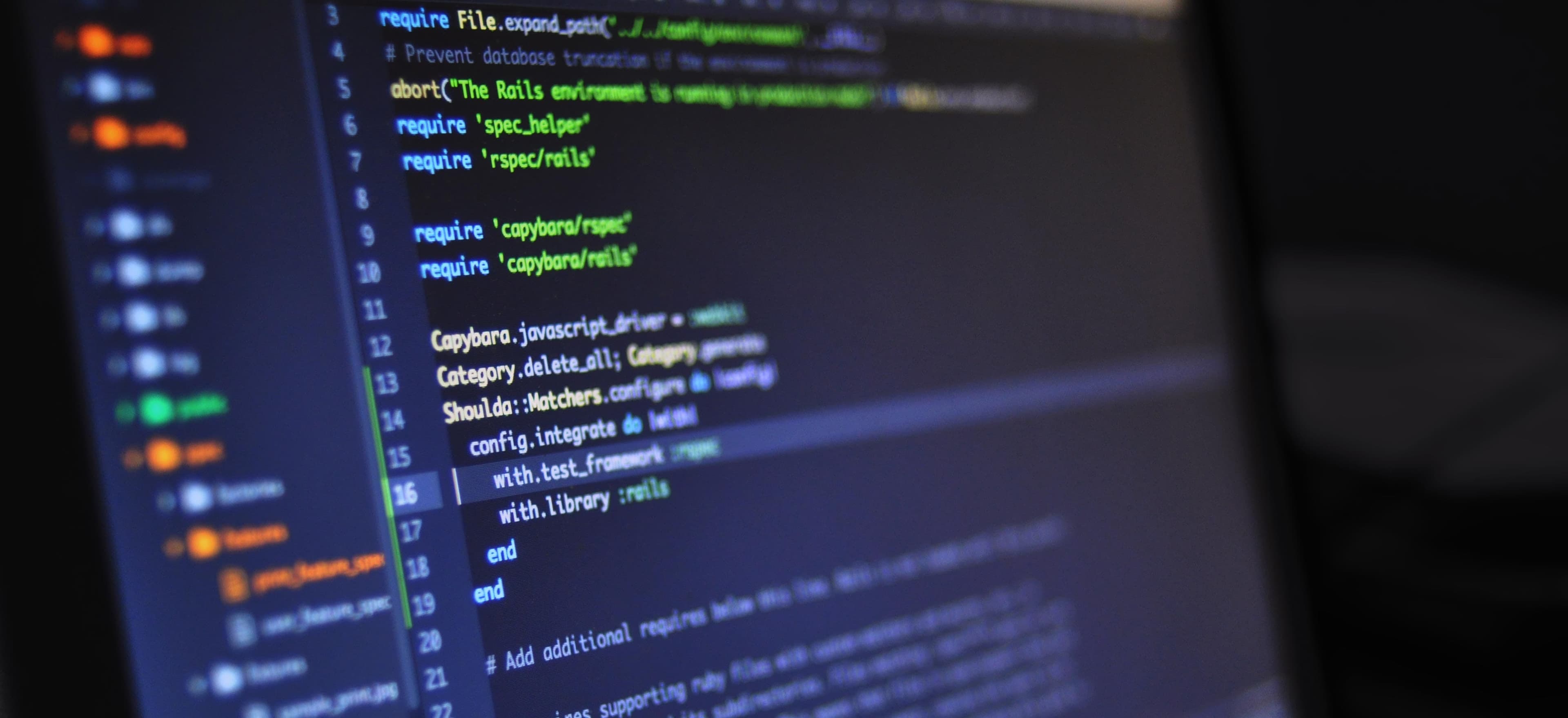
- Published on
Mastering Explicit and Implicit Conversions in Java
In Java, data type conversions play a crucial role in manipulating data and ensuring compatibility between different data types. Understanding the concepts of explicit and implicit conversions is essential for every Java developer. In this blog post, we will delve into the nuances of explicit and implicit conversions, explore their significance, and provide practical examples to demonstrate their use cases.
Implicit Conversions
Implicit conversions, also known as widening conversions, occur when a data type with a smaller range is automatically converted to a data type with a larger range. In Java, implicit conversions happen seamlessly, as the compiler handles the conversion without requiring any explicit intervention from the developer.
Example of Implicit Conversion
int intValue = 10;
long longValue = intValue; // Implicit conversion from int to long
In the above example, the int
value is implicitly converted to a long
value without the need for any explicit casting. This is possible because a long
can accommodate a wider range of values compared to an int
.
Implicit conversions are intuitive and convenient, but developers should be cautious when relying on them, especially when dealing with mixed data types in complex operations.
Explicit Conversions
Explicit conversions, also known as narrowing conversions, occur when a data type with a larger range is explicitly converted to a data type with a smaller range. In Java, explicit conversions require the use of casting to indicate the intended conversion, and it is the developer's responsibility to ensure that the conversion is safe and does not result in loss of data or precision.
Example of Explicit Conversion
double doubleValue = 10.5;
int intValue = (int) doubleValue; // Explicit conversion from double to int
In the above example, the double
value is explicitly converted to an int
value using casting. It's important to note that when performing explicit conversions, there is a risk of losing precision or encountering overflow/underflow if the source value exceeds the range of the target data type.
When to Use Explicit Conversions
Explicit conversions are commonly used in scenarios where the developer needs to explicitly control the conversion process or when dealing with mixed data types in expressions or assignments. It's crucial to apply explicit conversions prudently and ensure that the converted value remains within the valid range of the target data type.
Practical Example
Let's consider a practical scenario where both explicit and implicit conversions come into play. Suppose we have an array of int
values, and we need to calculate the average, storing the result in a double
variable.
int[] values = {10, 20, 30, 40, 50};
int sum = 0;
for (int value : values) {
sum += value;
}
double average = (double) sum / values.length;
In this example, we use explicit conversion (double) sum
to ensure that the division operation results in a double
value, thus preserving the fractional part of the average.
Best Practices and Considerations
-
Avoid Truncation: When performing explicit conversions, be mindful of the potential loss of data or precision, especially when converting from a wider range data type to a narrower range data type.
-
Be Cautious with Mixed Data Types: When dealing with expressions involving mixed data types, consider using explicit conversions to ensure consistency and avoid unintended side effects.
-
Consider Alternative Approaches: In some cases, it may be beneficial to consider alternative approaches, such as using wrapper classes or utility methods, to handle conversions more effectively and safely.
Bringing It All Together
Mastering explicit and implicit conversions is fundamental for writing robust and reliable Java code. By understanding the nuances of these conversions and utilizing them judiciously, developers can ensure data integrity and mitigate potential issues related to type compatibility and range constraints. Whether it's accommodating larger data types through implicit conversions or controlling the precision of conversions through explicit casting, proficiency in handling data type conversions is a hallmark of a proficient Java developer.
Incorporating explicit and implicit conversions effectively leads to code that is not only efficient but also resilient to data inconsistencies and type mismatches.
Now that you have a solid understanding of explicit and implicit conversions in Java, it's time to put this knowledge into practice and elevate your coding skills to new heights.
Remember, mastering explicit and implicit conversions is not just about knowing how to convert data types; it's about leveraging this knowledge to write more robust and efficient code.
I hope this blog post has shed light on the significance of explicit and implicit conversions in Java and provided valuable insights into their practical application. Happy coding!
Java Documentation on Type Conversion - For a more in-depth understanding of type conversion in Java.
Understanding Type Casting in Java - An additional resource to delve deeper into the concept of type casting in Java.
Thank you for reading!