Mapping JPA Annotations for Effective Data Model Reflection
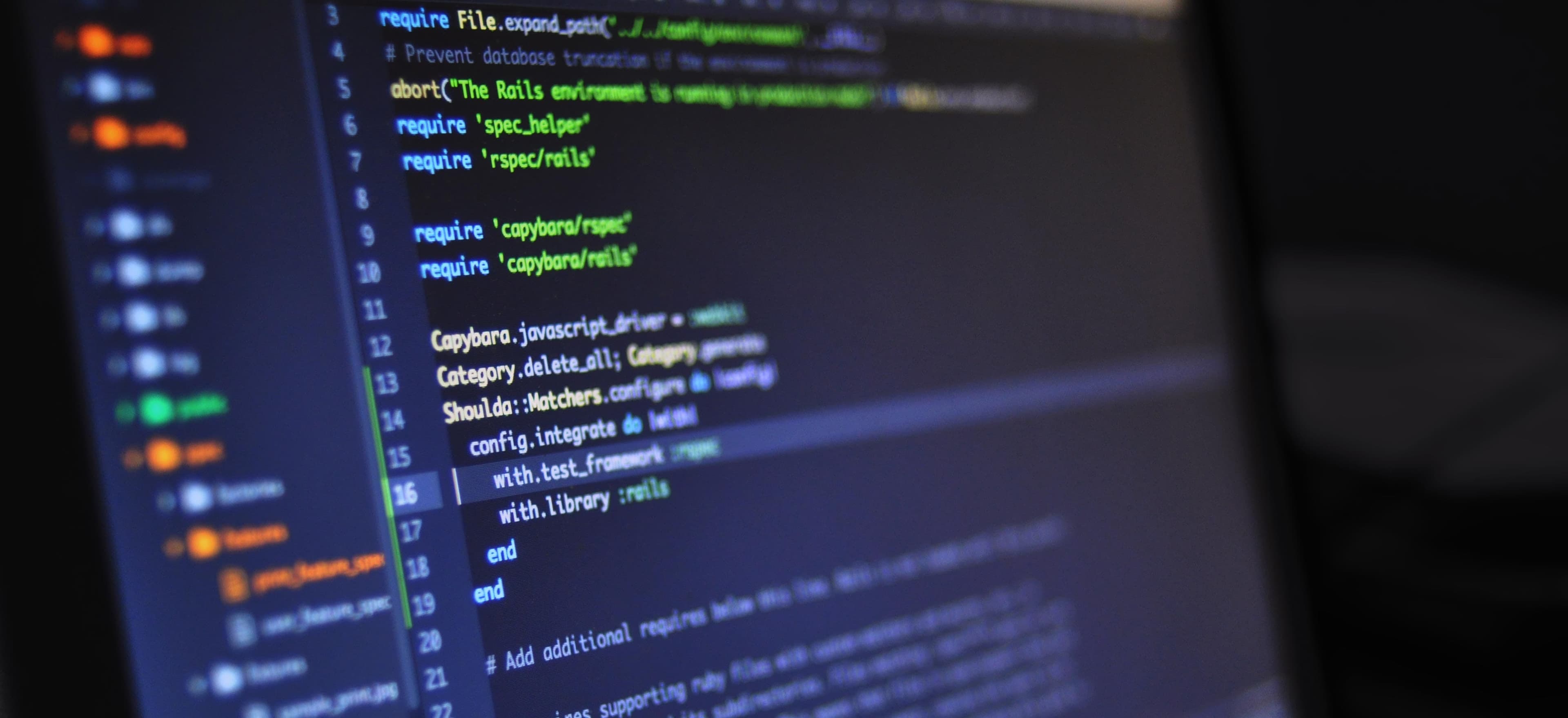
- Published on
Mapping JPA Annotations for Effective Data Model Reflection
When working with Java Persistence API (JPA), it's crucial to understand how to effectively map Java objects to a relational database. Proper mapping not only ensures data integrity but also enhances the performance of the application. In this article, we'll delve into the world of JPA annotations and explore how they can be used to create a robust and efficient data model reflective of the underlying database structure.
What is JPA?
Java Persistence API (JPA) is a specification for managing relational data in Java applications. It provides a way to map Java objects to database tables and vice versa, allowing developers to interact with a database using standard Java object-oriented paradigms. JPA implementations, such as Hibernate, EclipseLink, and OpenJPA, enable developers to work with databases in a vendor-agnostic manner.
The Power of JPA Annotations
JPA annotations play a pivotal role in defining the mapping between Java entities and database tables. By using annotations, developers can specify various attributes such as table names, column names, relationships between entities, and inheritance mappings. This declarative approach simplifies the persistence logic and reduces boilerplate code, resulting in cleaner and more maintainable data access layers.
Let's dive into some of the most commonly used JPA annotations and how they can be leveraged to create a cohesive data model.
@Entity
The @Entity
annotation is used to mark a Java class as a persistent entity. It indicates that the class should be mapped to a corresponding table in the database.
@Entity
@Table(name = "employees")
public class Employee {
// class members and methods
}
In this example, the Employee
class is designated as an entity and will be mapped to a table named "employees" in the database. The @Table
annotation is used to specify the name of the table explicitly. If the table name matches the class name, the @Table
annotation can be omitted.
@Id and @GeneratedValue
The @Id
annotation is used to denote the primary key of the entity, while the @GeneratedValue
annotation is used to specify the strategy for generating the primary key values.
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
In this snippet, the id
field is annotated with @Id
to indicate that it serves as the primary key for the Employee
entity. The @GeneratedValue
annotation with the GenerationType.IDENTITY
strategy instructs the database to automatically generate unique primary key values upon insertion.
@Column
The @Column
annotation is used to customize the mapping of entity attributes to table columns. It allows developers to specify attributes such as column name, length, nullable constraints, and more.
@Column(name = "full_name", nullable = false, length = 100)
private String fullName;
In this example, the fullName
attribute is mapped to a column named "full_name" in the database table. Additionally, the nullable
attribute is set to false
, indicating that the column cannot contain null values, and the length
attribute specifies the maximum length of the column.
Relationships: @OneToOne, @OneToMany, @ManyToOne, @ManyToMany
JPA provides annotations to define relationships between entities, such as @OneToOne
, @OneToMany
, @ManyToOne
, and @ManyToMany
. These annotations enable developers to establish associations between entities, reflecting the underlying database schema.
@Entity
public class Department {
// other attributes and methods
@OneToMany(mappedBy = "department")
private List<Employee> employees;
}
In this example, the Department
entity has a one-to-many relationship with the Employee
entity. The @OneToMany
annotation, along with the mappedBy
attribute, establishes the relationship, indicating that the employees
attribute in the Department
class maps to the department
attribute in the Employee
class.
Inheritance: @Inheritance and @DiscriminatorColumn
JPA supports inheritance mapping through annotations such as @Inheritance
and @DiscriminatorColumn
. These annotations allow developers to implement inheritance strategies, including single table, joined, and table per class hierarchies.
@Entity
@Inheritance(strategy = InheritanceType.SINGLE_TABLE)
@DiscriminatorColumn(name = "employee_type")
public class Employee {
// common attributes and methods
}
In this snippet, the Employee
class serves as the root entity for inheritance mapping. The @Inheritance
annotation with InheritanceType.SINGLE_TABLE
specifies the inheritance strategy as single table, while the @DiscriminatorColumn
annotation declares a discriminator column named "employee_type" to differentiate between different types of employees.
In Conclusion, Here is What Matters
In this article, we've explored the power of JPA annotations in mapping Java entities to a relational database. By leveraging annotations such as @Entity
, @Id
, @Column
, and relationship mappings, developers can create a well-defined and efficient data model that accurately reflects the underlying database schema. Understanding and effectively using these annotations is crucial for developing robust and performant JPA-based applications.
JPA annotations provide a declarative and intuitive means of defining the persistence logic, allowing developers to focus on the domain model and business logic rather than low-level database interactions. Mastering the art of JPA annotation mapping is essential for building scalable and maintainable Java applications with a strong foundation in data persistence.
By combining the power of JPA annotations with best practices in database design, developers can create data models that not only adhere to the principles of object-oriented programming but also optimize database performance and maintainability.
In conclusion, JPA annotations serve as a bridge between the world of Java objects and relational databases, enabling seamless interaction and persistence of data. Embracing and mastering these annotations is a pivotal step toward becoming a proficient Java developer proficient in building robust, efficient, and maintainable data access layers.
By now, you should have a solid understanding of how to effectively utilize JPA annotations for mapping Java entities, and you're ready to craft a cohesive and performant data model reflective of your database schema.
So go ahead, embrace the power of JPA annotations, and architect data models that lay a solid foundation for your Java applications!
Remember, great data models lead to great applications!
Happy coding!