Maximizing Efficiency: Blue-Green Deployment Strategies
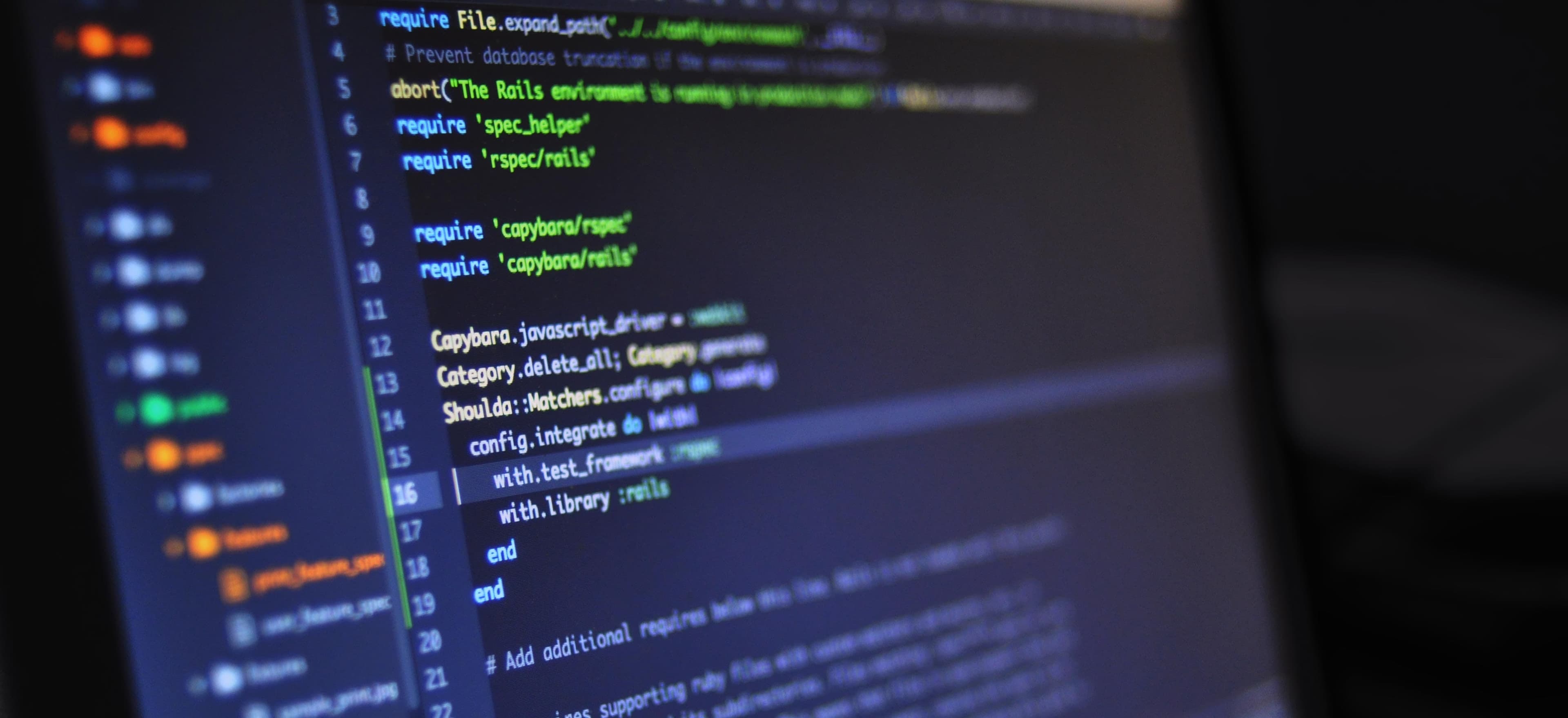
- Published on
Maximizing Efficiency: Blue-Green Deployment Strategies
In the fast-evolving world of software development, deployment strategies play a critical role in ensuring seamless rollouts and minimizing downtime. One such strategy that has gained significant traction is the Blue-Green deployment. This approach not only aims to reduce deployment risks but also maximizes resource utilization and enhances overall efficiency. In this article, we will delve into the concept of Blue-Green deployments, explore its benefits, and examine how Java can be leveraged to implement this strategy effectively.
Understanding Blue-Green Deployments
Blue-Green deployment is a technique used to reduce downtime and risk when deploying application updates. In this approach, two identical production environments, aptly referred to as Blue and Green, are utilized. At any given time, only one of these environments actively serves live user traffic while the other remains idle. The active environment handles all ongoing operations and transactions. When a new release is ready to be deployed, it is installed in the inactive environment, essentially creating a parallel environment for the updated version.
Once the update is successfully deployed and validated in the inactive environment, the traffic is seamlessly switched from the active environment (let's say, Blue) to the updated environment (Green) without interrupting user experience. The inactive environment becomes the new active environment, ready to serve user traffic, while the previous active environment (Blue) is then idle and available for future updates or rollbacks.
Benefits of Blue-Green Deployments
Minimized Downtime
Blue-Green deployments significantly reduce downtime during updates as the cutover from one environment to the other is instantaneous. This ensures that end-users experience minimal disruption, if any, during the deployment process.
Risk Mitigation
By maintaining two identical production environments, Blue-Green deployments provide a safety net in case of unexpected issues post-deployment. If the updated environment experiences any problems, reverting to the previous environment requires a simple traffic switch, swiftly mitigating the risks associated with faulty deployments.
Seamless Rollbacks
In scenarios where the new release introduces unforeseen bugs or issues, rolling back to the previous version is straightforward. Instead of performing complex and time-consuming rollback procedures, you simply redirect the traffic back to the proven, stable environment.
Scalability and Resource Efficiency
Blue-Green deployments facilitate efficient resource utilization as both environments can be leveraged for tasks such as testing, quality assurance, and performance tuning, without impacting the live environment. This scalability ensures that the system can handle increased load efficiently, while maintaining high performance and availability.
Implementing Blue-Green Deployments in Java
Java, with its robust ecosystem and multitude of tools, provides a solid foundation for implementing Blue-Green deployments effectively. Let's explore some key considerations and best practices when employing this deployment strategy in a Java-based environment.
Configuration Management
Central to the success of Blue-Green deployments is the seamless management of configuration settings. Utilizing tools such as Spring Cloud Config allows for externalized configuration management, enabling the application to dynamically pick up configuration changes without requiring a restart. This dynamic configuration management is crucial in ensuring that both the Blue and Green environments stay in sync with the latest configuration settings, thereby reducing the chances of configuration-related discrepancies.
// Example of dynamically picking up configuration changes using Spring Cloud Config
@RefreshScope
@RestController
public class ConfigurationController {
@Value("${feature.flag}")
private boolean featureFlag;
@GetMapping("/feature-flag")
public boolean isFeatureFlagEnabled() {
return featureFlag;
}
}
In this code snippet, the @Value
annotation allows for the dynamic retrieval of configuration settings, and the @RefreshScope
annotation enables the ability to refresh the configuration properties at runtime without requiring a full application restart.
Health Checks and Load Balancing
In a Blue-Green deployment setup, efficient health checks and load balancing are crucial for seamless traffic cutover and ensuring high availability. Leveraging tools like Netflix Eureka and Ribbon for service discovery and client-side load balancing, in combination with Spring Boot Actuator for health checks, can significantly enhance the robustness and reliability of the deployment setup.
// Health check endpoint using Spring Boot Actuator
@GetMapping("/actuator/health")
public ResponseEntity<String> healthCheck() {
// Perform health checks and return appropriate response
}
Implementing custom health checks specific to the application's requirements ensures that the new environment is fully operational and ready to handle the incoming traffic before the cutover.
Automated Testing and Validation
Automation plays a pivotal role in validating the integrity of the newly deployed environment. Incorporating automated integration tests, end-to-end tests, and canary analysis allows for thorough validation of the Green environment before it becomes the active production environment. Tools such as JUnit, Mockito, and Selenium are widely used in the Java ecosystem for automated testing and validation.
// Example of a JUnit test for validating a REST API endpoint
@Test
public void testApiEndpoint() {
// Perform API request and validate the response
// Assert the expected outcome
}
Automated tests provide the assurance that the new release functions as expected, and any discrepancies are identified before the traffic switch occurs.
Database Migrations and Compatibility
Managing database schema changes and data migrations is a critical aspect of Blue-Green deployments. Employing frameworks like Flyway or Liquibase for versioning and applying database changes ensures that both environments stay in sync, and data consistency is maintained across deployments. Additionally, continuous integration and continuous deployment (CI/CD) pipelines, integrated with tools like Jenkins or GitLab CI, streamline the database migration process and enforce compatibility across the Blue and Green environments.
// Example Flyway migration script for adding a new table
CREATE TABLE new_table (
id INT PRIMARY KEY,
name VARCHAR(255)
);
By maintaining versioned database scripts and automation for migration execution, database compatibility between the environments is upheld, allowing for a seamless transition between the Blue and Green environments.
Final Considerations
Blue-Green deployments offer a potent strategy for achieving high availability, minimizing downtime, and mitigating risks during software deployments. With Java's versatility and a wide array of tools and frameworks tailored for robust deployment strategies, implementing Blue-Green deployments becomes a feasible and efficient endeavor. By leveraging the principles and practices highlighted in this article, organizations can harness the power of Blue-Green deployments to ensure seamless rollouts, optimal resource utilization, and enhanced operational efficiency.
Incorporating Blue-Green deployment strategies into the software development lifecycle can yield significant dividends in terms of reliability, stability, and overall user experience. Embracing these best practices and leveraging the capabilities of Java empowers organizations to stay at the forefront of deployment excellence in today's dynamic digital landscape.
Implementing Blue-Green deployments is crucial for seamless rollouts and optimal resource utilization. Contact us for expert insights on deploying Java applications at [your company name] and learn how to stay ahead in the ever-evolving software deployment landscape.