Resolving Emacs Clojure Mode Package Dependency Issues
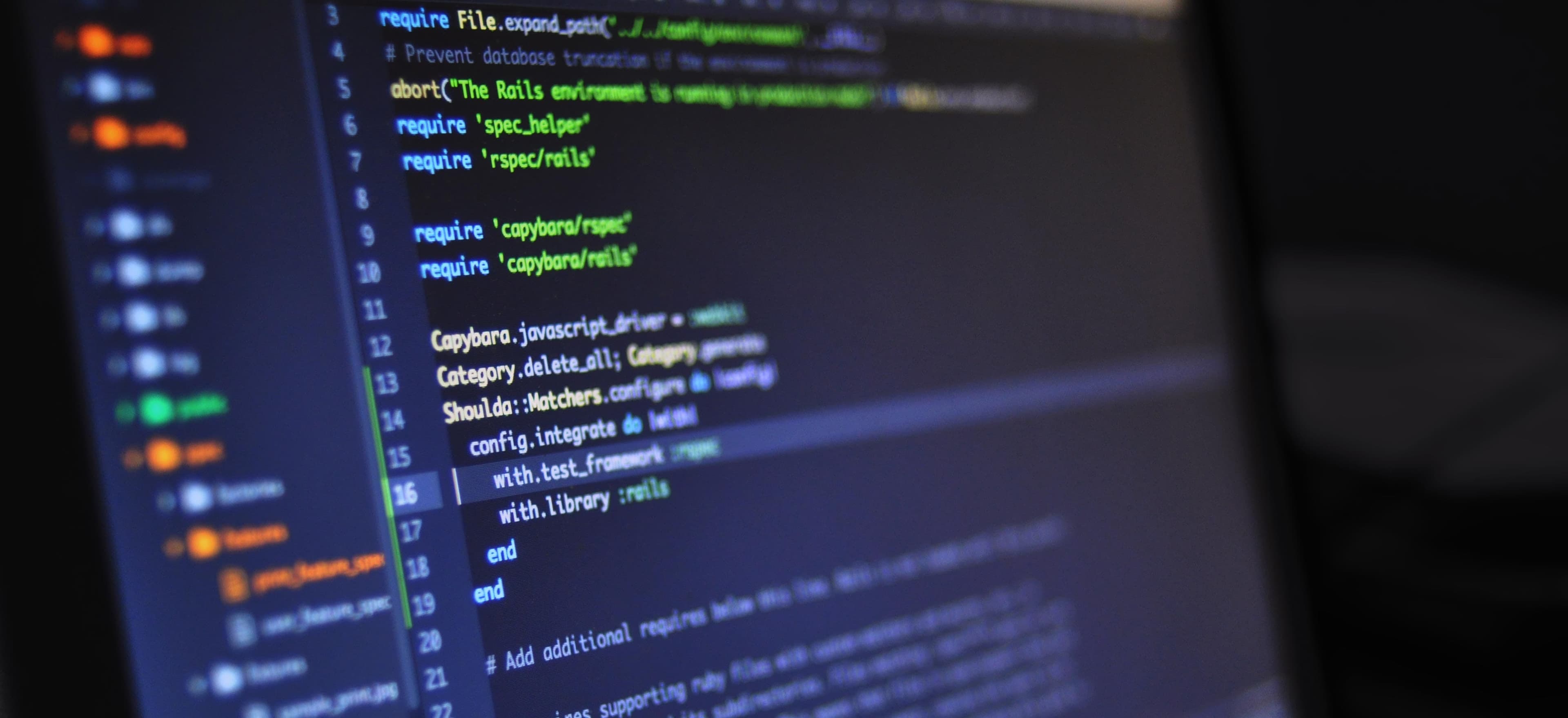
- Published on
Resolving Emacs Clojure Mode Package Dependency Issues
When working with Clojure programming language in Emacs, you may encounter package dependency issues that can disrupt your workflow. These issues often arise due to conflicts between different packages or versions. Fortunately, there are several steps you can take to resolve these problems and ensure that your Emacs environment is properly configured for Clojure development.
Understanding Package Dependency Issues
Before diving into the solutions, it's essential to understand the nature of package dependency issues in Emacs. When you work with Clojure mode in Emacs, you might be using packages like cider
for Clojure development, clojure-mode
for syntax highlighting and indentation, and clj-refactor
for refactoring. These packages often depend on each other, and conflicts can occur when different versions are installed, or when packages are not properly configured to work together.
Updating Package Repositories
One of the most common causes of package dependency issues is outdated package repositories. To ensure that you have access to the latest versions of packages, it's crucial to update the package repositories in your Emacs configuration. You can do this by adding the following code to your Emacs configuration file (usually init.el
):
(require 'package)
(add-to-list 'package-archives
'("melpa" . "https://melpa.org/packages/") t)
(package-initialize)
This code snippet adds the Melpa repository, a popular source for Emacs packages, to your package archives. By updating the repositories, you can access the most recent versions of the packages, potentially resolving any version conflicts that may be causing dependency issues.
Managing Package Versions with use-package
The use-package
macro is a powerful tool for managing package configurations in Emacs. It allows you to specify package dependencies, load packages only when needed, and configure package settings concisely. By using use-package
, you can ensure that the required versions of packages are installed and activated for Clojure development.
Below is an example of how you can use use-package
to manage Clojure-related packages:
(use-package cider
:ensure t
:config
(add-hook 'cider-mode-hook #'eldoc-mode))
(use-package clojure-mode
:ensure t
:config
(require 'flycheck-clj-kondo)
(add-hook 'clojure-mode-hook 'flycheck-mode))
(use-package clj-refactor
:ensure t
:config
(add-hook 'clojure-mode-hook
(lambda ()
(clj-refactor-mode 1)
(cljr-add-keybindings-with-prefix "C-c C-m"))))
In this example, use-package
is used to ensure that the cider
, clojure-mode
, and clj-refactor
packages are installed. Additionally, specific configurations and hooks are applied to each package, ensuring that they work seamlessly together for Clojure development.
Resolving Version Conflicts
If you are experiencing version conflicts between different packages, you might need to explicitly specify the versions of the packages to resolve the conflicts. This can be achieved using the :pin
keyword within the use-package
declaration. For example:
(use-package cider
:ensure t
:pin "melpa-stable")
In this snippet, :pin "melpa-stable"
ensures that the cider
package is installed from the Melpa Stable repository, potentially resolving conflicts with other packages that depend on different versions of cider
.
Understanding Package Initialization Order
Another common source of package dependency issues in Emacs is the initialization order of packages. If the order in which packages are loaded conflicts with their dependencies, it can lead to errors and unexpected behavior. To address this, you can use the :after
keyword in use-package
to specify the order in which packages should be loaded.
For example:
(use-package clojure-mode
:ensure t
:after cider)
In this instance, clojure-mode
is configured to load after cider
, ensuring that any dependencies of clojure-mode
on cider
are satisfied.
Troubleshooting Using package-list-packages
If you have followed the steps above and are still encountering package dependency issues, it can be helpful to use the package-list-packages
command in Emacs to inspect the currently installed packages and their versions. This can assist in identifying conflicting versions or missing dependencies, allowing you to rectify the issues manually.
To use package-list-packages
, simply type M-x package-list-packages
in Emacs, and you will be presented with a list of installed packages along with their versions. You can then update, remove, or install specific versions of packages as needed to resolve dependency conflicts.
Final Considerations
In conclusion, package dependency issues in Emacs can be a common hurdle when working with Clojure mode for development. By understanding the nature of these issues and utilizing tools like use-package
to manage package configurations, you can effectively resolve dependency problems and ensure a smooth and efficient Clojure development environment in Emacs.
Addressing package dependency issues not only streamlines your workflow but also enhances the stability and consistency of your development environment. With a well-configured Emacs setup, you can fully leverage the power of Clojure for your programming projects.
By applying the techniques and best practices discussed in this post, you can overcome package dependency issues and enjoy a seamless Clojure development experience in Emacs.