Troubleshooting Common Problems with TestNG Annotations
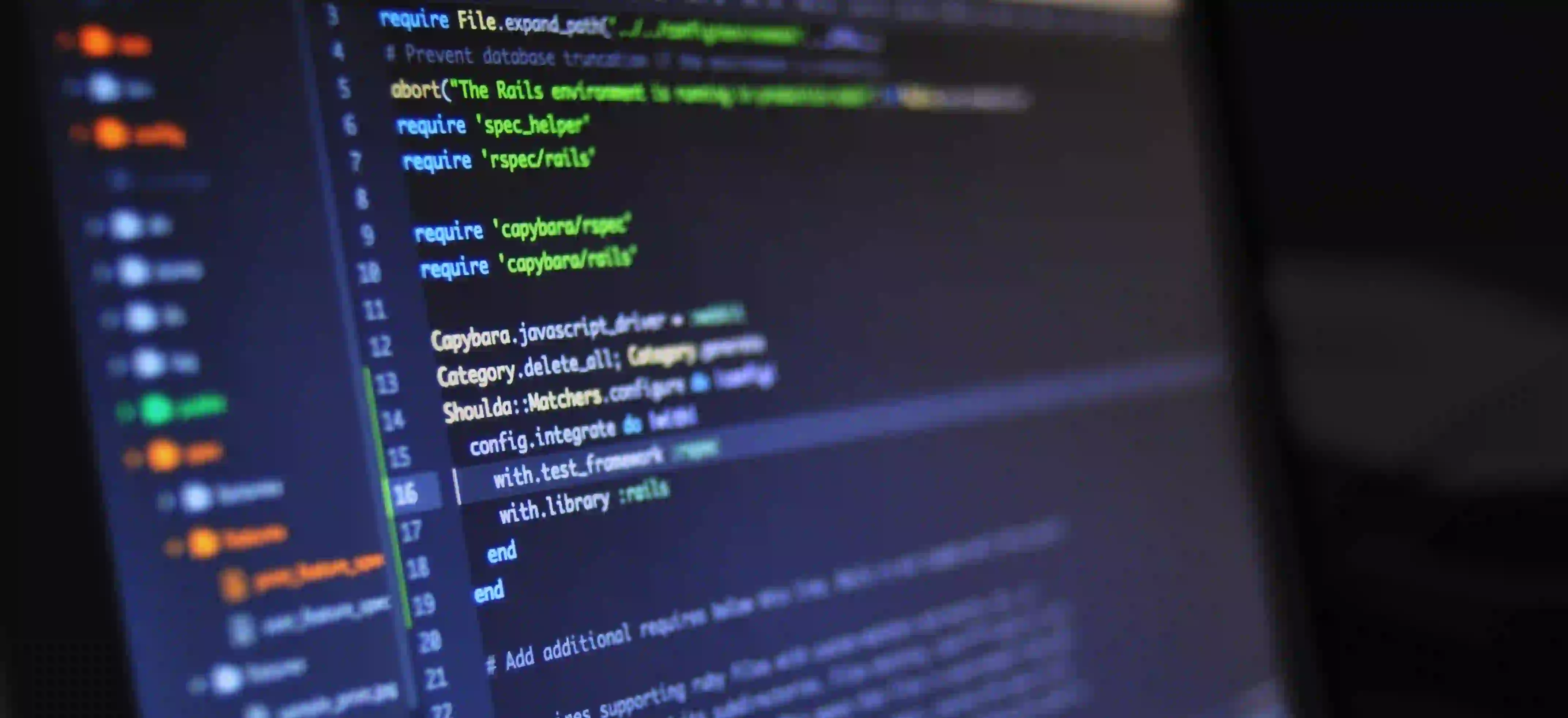
Troubleshooting Common Problems with TestNG Annotations
TestNG is a powerful testing framework for Java that simplifies the process of writing and running tests. However, like any complex tool, it can sometimes lead to confusing errors that can be challenging to debug. In this blog post, we'll explore some common problems that developers encounter when using TestNG annotations and provide solutions to troubleshoot these issues effectively.
Problem 1: TestNG annotations are not being recognized
One common issue that developers face is when TestNG annotations such as @Test
or @BeforeMethod
are not being recognized by the TestNG framework. This can lead to tests not being executed as expected.
To troubleshoot this issue, ensure that the TestNG library is included in the project's dependencies. If using Maven, the following dependency should be added to the pom.xml
file:
<dependency>
<groupId>org.testng</groupId>
<artifactId>testng</artifactId>
<version>7.3.0</version> <!-- Replace with the latest version -->
<scope>test</scope>
</dependency>
Additionally, verify that the test classes are correctly annotated with @Test
and other relevant TestNG annotations. It's essential to import the annotations from the org.testng.annotations
package to ensure that the correct annotations are used.
Problem 2: TestNG methods are not executing in the expected order
TestNG provides the ability to define the order in which test methods should be executed using the priority
attribute or by using dependsOnMethods
or dependsOnGroups
. However, developers often encounter issues where test methods are not executing in the expected order, leading to test failures.
One common reason for this problem is when the priority
attribute is not used correctly. The priority
attribute takes an integer value, and TestNG executes test methods in ascending order of their priority values. If two test methods have the same priority, the execution order is non-deterministic.
Another potential cause of this problem is when there are circular dependencies between test methods defined using dependsOnMethods
or dependsOnGroups
. TestNG does not support circular dependencies, and defining them can lead to unexpected behavior.
To troubleshoot this issue, review the priority
attribute usage and ensure that the values are assigned correctly to establish the desired execution order. Additionally, analyze the dependsOnMethods
and dependsOnGroups
dependencies to identify and remove any circular dependencies.
Problem 3: TestNG configuration methods are not running before/after tests
TestNG allows developers to define configuration methods such as @BeforeClass
, @AfterClass
, @BeforeMethod
, and @AfterMethod
to set up and tear down the test environment. However, it's not uncommon for developers to face issues where these configuration methods are not executed as expected.
One potential reason for this problem is when the configuration methods are not annotated correctly or are placed in the wrong class. Ensure that the configuration methods are annotated with the appropriate TestNG annotations and are located in the test classes where they need to be executed.
Another common mistake is when the test methods are not part of a test suite. TestNG configuration methods are executed only when the test classes are part of a test suite XML file. Ensure that the test classes are included in the test suite XML to enable the execution of configuration methods.
Problem 4: TestNG skips tests without a clear reason
In some cases, developers may find that TestNG is skipping tests without providing a clear reason for the skip. This unexpected behavior can be frustrating, especially when trying to identify the root cause of the skipped tests.
One potential cause of this problem is when the test methods are not meeting the criteria specified in the testng.xml file. TestNG allows developers to define inclusion and exclusion criteria for test methods using <include>
and <exclude>
tags in the test suite XML. If the test methods do not meet the specified criteria, TestNG skips the tests without executing them.
To troubleshoot this issue, review the testng.xml file and analyze the inclusion and exclusion criteria to ensure that the test methods are appropriately included for execution.
Another reason for skipped tests could be due to the presence of a enabled
attribute set to false
in the @Test
annotation. If the enabled
attribute is explicitly set to false
, TestNG will skip the test method without executing it.
The Closing Argument
In this blog post, we explored some common problems that developers encounter when using TestNG annotations and provided troubleshooting solutions to address these issues effectively. By understanding the potential reasons behind these problems and implementing the recommended solutions, developers can ensure a smooth and efficient testing process with TestNG.
TestNG is a robust testing framework that, when used correctly, can greatly enhance the testing capabilities for Java applications. By familiarizing themselves with the common issues and solutions discussed in this post, developers can leverage the full potential of TestNG and streamline their testing workflows.
Remember, when facing issues with TestNG annotations or any other aspect of the framework, thorough troubleshooting and careful review of the code, configuration, and test suite files can often lead to the discovery of the root cause and a successful resolution.
For more information on TestNG, you can visit the official TestNG website and explore the comprehensive documentation to further enhance your understanding of the framework.
Happy testing with TestNG!