Building Cross-Platform Mobile Apps with Java - A Complete Guide
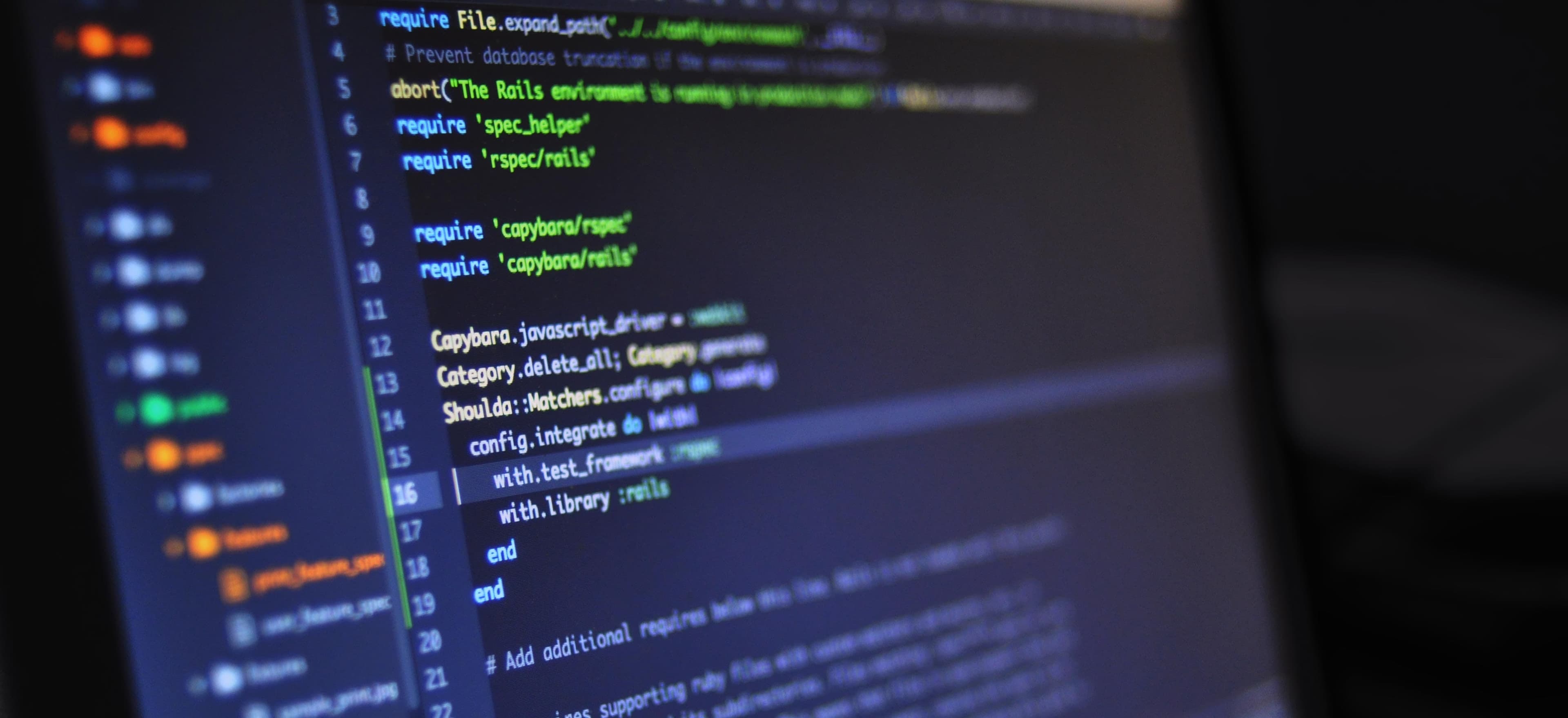
- Published on
Building Cross-Platform Mobile Apps with Java - A Complete Guide
Mobile app development is a crucial aspect of today's tech landscape, and choosing the right platform can be a challenging decision for developers. Java, a versatile and widely-used programming language, offers a compelling option for building cross-platform mobile apps. In this guide, we'll delve into the world of Java for mobile development, exploring its benefits, tools, and best practices to empower you in creating top-notch apps.
Why Java for Cross-Platform Mobile App Development?
Versatility and Familiarity
Java's versatility makes it an ideal choice for cross-platform mobile app development. With Java, developers can write code once and run it on multiple platforms, saving time and resources. Additionally, the widespread use of Java in enterprise-level applications means that many developers are already familiar with its syntax and ecosystem.
Robust Ecosystem
Java boasts a robust ecosystem with a myriad of libraries, frameworks, and tools specifically designed for mobile app development. Platforms like Android, powered by Java, provide extensive support and documentation, making it easier for developers to create high-quality mobile apps.
Tools for Cross-Platform Mobile Development with Java
1. Codename One
Codename One is a powerful open-source platform that allows developers to build native mobile applications using Java. It provides a seamless way to create cross-platform apps with a single codebase, leveraging the native capabilities of each platform.
2. Gluon
Gluon offers a comprehensive set of tools and libraries for Java developers to build cross-platform mobile applications. With Gluon, developers can create stunning, high-performance apps that run seamlessly on iOS and Android devices.
Getting Started with Java for Cross-Platform Mobile Development
To kickstart your journey in building cross-platform mobile apps with Java, let's dive into some exemplary code snippets and gain insights on best practices.
Example 1: Creating a Basic User Interface Using JavaFX
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class Main extends Application {
@Override
public void start(Stage primaryStage) {
Button btn = new Button("Click me!");
StackPane root = new StackPane();
root.getChildren().add(btn);
Scene scene = new Scene(root, 300, 250);
primaryStage.setTitle("Cross-Platform App");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
In this code snippet, we utilize JavaFX, a versatile GUI toolkit, to create a basic user interface for our cross-platform mobile app. JavaFX provides a platform-independent way to construct graphical interfaces, allowing for easy adaptation across different devices.
Example 2: Implementing Platform-Specific Features
import com.codename1.ui.Display;
import com.codename1.ui.Form;
import com.codename1.ui.Label;
public class Main {
public void createUI() {
Form form = new Form("My App");
if (Display.getInstance().isAndroid()) {
form.add(new Label("Welcome to Android App"));
} else if (Display.getInstance().isIOS()) {
form.add(new Label("Welcome to iOS App"));
}
form.show();
}
}
In this code snippet, we leverage Codename One to implement platform-specific features within our mobile app. By detecting the platform at runtime, we can tailor the user experience to meet the unique requirements of each platform, enhancing overall user satisfaction.
Best Practices for Java Cross-Platform Mobile Development
1. Embrace Responsive Design
Cross-platform apps should prioritize responsive design to ensure optimal user experience across various devices and screen sizes. Utilize layout managers and adaptive UI components to accommodate diverse form factors seamlessly.
2. Leverage Native Capabilities
While maintaining a single codebase, capitalize on platform-specific APIs and capabilities to deliver a native-like experience. This approach enhances performance and takes full advantage of each platform's unique features.
3. Continuous Testing and Optimization
Regular testing across different platforms and devices is critical to identify and rectify any compatibility or performance issues. Strive for continuous optimization to deliver a consistently high-quality user experience.
The Bottom Line
Java presents a compelling option for developers seeking to build cross-platform mobile apps with efficiency and scalability. Its robust ecosystem, coupled with versatile tools like Codename One and Gluon, empowers developers to create high-performance apps that cater to a broad user base. By following best practices and leveraging platform-specific capabilities, developers can ensure seamless functionality and user satisfaction across multiple platforms. Embrace the power of Java for cross-platform mobile app development and embark on the journey to create impactful, versatile apps that resonate with diverse audiences.
Start your cross-platform mobile development journey with Java today and unlock the potential to reach a broader audience with your exceptional apps!
Learn more about Java for mobile app development
Explore the capabilities of Codename One for cross-platform app development