Managing Mutable State in Java Methods
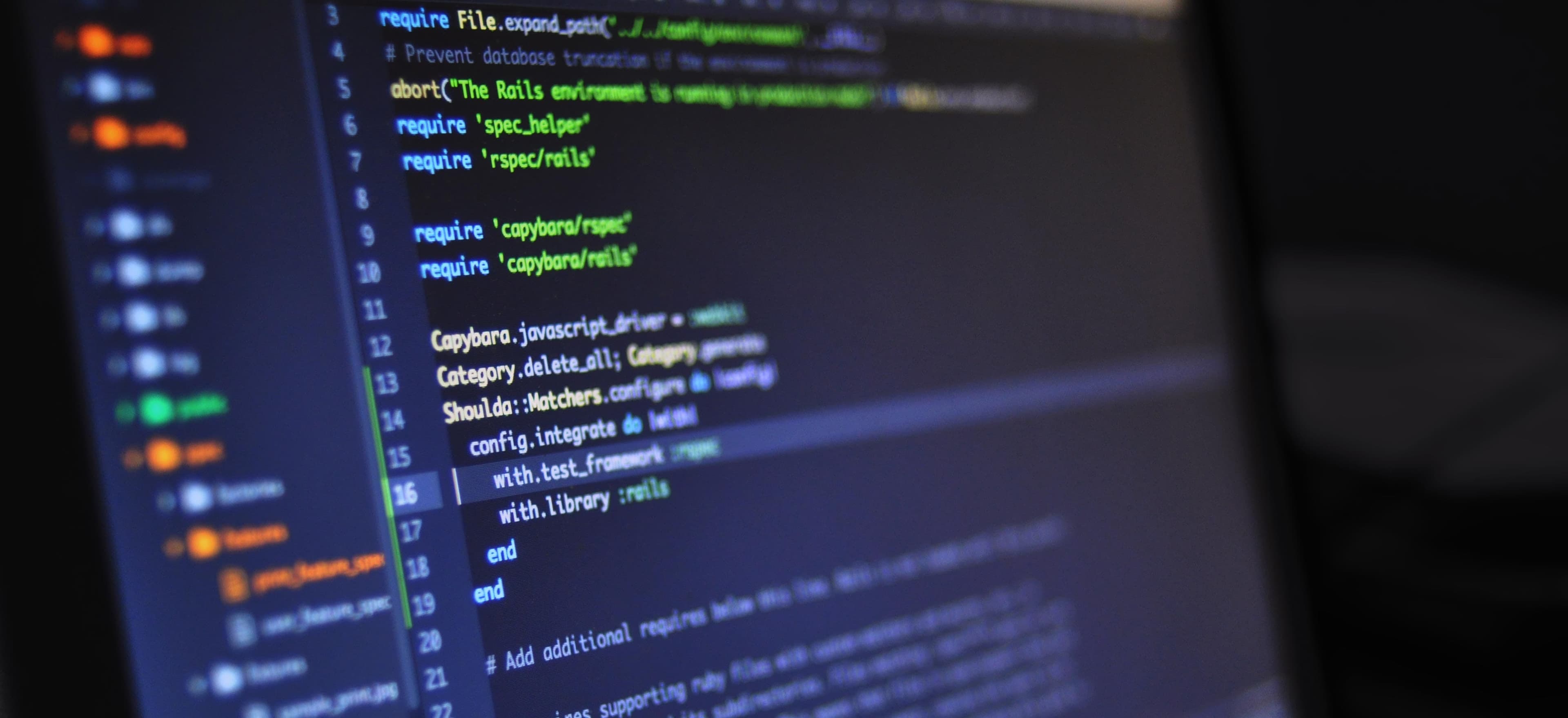
- Published on
Managing Mutable State in Java Methods
In Java programming, it’s essential to understand how to manage mutable state within methods. Mutable state refers to an object whose state can be modified after it's been created. Managing mutable state effectively can help prevent unexpected behavior and bugs in a program. In this article, we will discuss some best practices and techniques for managing mutable state within Java methods.
Use Immutable Objects Whenever Possible
One of the best ways to manage mutable state in Java methods is to use immutable objects. Immutable objects are objects whose state cannot be changed after they are created. By using immutable objects, you can avoid the complexities of managing mutable state altogether. Immutable objects are also inherently thread-safe, making them ideal for use in concurrent applications.
Here's an example of an immutable object in Java:
public final class ImmutableObject {
private final int value;
public ImmutableObject(int value) {
this.value = value;
}
public int getValue() {
return value;
}
}
In the ImmutableObject
class, the value
field is declared as final
, and the class itself is marked as final
. This ensures that the object's state cannot be modified after it's been created.
Minimize Mutability
If using immutable objects is not feasible for your use case, then another approach to managing mutable state is to minimize mutability within your methods. This means reducing the number of objects whose state can be modified and using them judiciously.
public class MutableObject {
private int value;
public void updateValue(int newValue) {
this.value = newValue;
}
public int getValue() {
return value;
}
}
In the MutableObject
class, the value
field can be modified through the updateValue
method. By minimizing mutability, you can control the state changes within your methods, making it easier to reason about the behavior of your code.
Encapsulation and Information Hiding
Encapsulation is a fundamental principle of object-oriented programming, and it plays a crucial role in managing mutable state. By encapsulating the state of an object and hiding the implementation details, you can prevent unauthorized access and modification of the object's state.
public class EncapsulatedObject {
private int value;
public void setValue(int value) {
if (value >= 0) {
this.value = value;
} else {
throw new IllegalArgumentException("Value must be non-negative");
}
}
public int getValue() {
return value;
}
}
In the EncapsulatedObject
class, the value
field is encapsulated, and access to it is restricted through the setValue
method, which enforces a validation rule. This prevents the object's state from being arbitrarily modified, ensuring data integrity.
Immutability Through Method Signatures
Another way to manage mutable state is by designing methods with immutability in mind. This involves using method parameters and return values to enforce immutability.
public class ImmutableMethod {
public ImmutableObject performOperation(ImmutableObject input, int value) {
int result = input.getValue() + value;
return new ImmutableObject(result);
}
}
In the ImmutableMethod
class, the performOperation
method takes an ImmutableObject
as input and returns a new ImmutableObject
as the result, without modifying the original input object. This approach promotes immutability and reduces the risk of unintended state changes.
Using Java Collections and Streams
When working with collections in Java, the Streams API provides powerful ways to manage mutable state. By leveraging immutable operations and the functional programming style of streams, you can perform transformations and computations on collections without directly modifying their state.
import java.util.Arrays;
import java.util.List;
public class CollectionExample {
public static void main(String[] args) {
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
long count = names.stream()
.filter(name -> name.length() > 4)
.count();
System.out.println("Number of names with more than 4 characters: " + count);
}
}
In the CollectionExample
class, the filter
operation on the stream does not modify the original list names
. Instead, it creates a new stream with the filtered elements, preserving the immutability of the original collection.
Key Takeaways
Managing mutable state in Java methods is crucial for writing robust and maintainable code. By using immutable objects, minimizing mutability, encapsulating state, designing for immutability, and leveraging Java collections and streams, you can effectively manage mutable state and reduce the potential for bugs and unexpected behavior in your code.
By following these best practices and techniques, you can write Java methods that are easier to reason about, test, and maintain, ultimately leading to more reliable and efficient software development.
In conclusion, mastering the art of managing mutable states in Java can significantly contribute to the overall elegance and robustness of your codebase.
Checkout our other articles