Formatting Numbers in Java: A Complete Guide
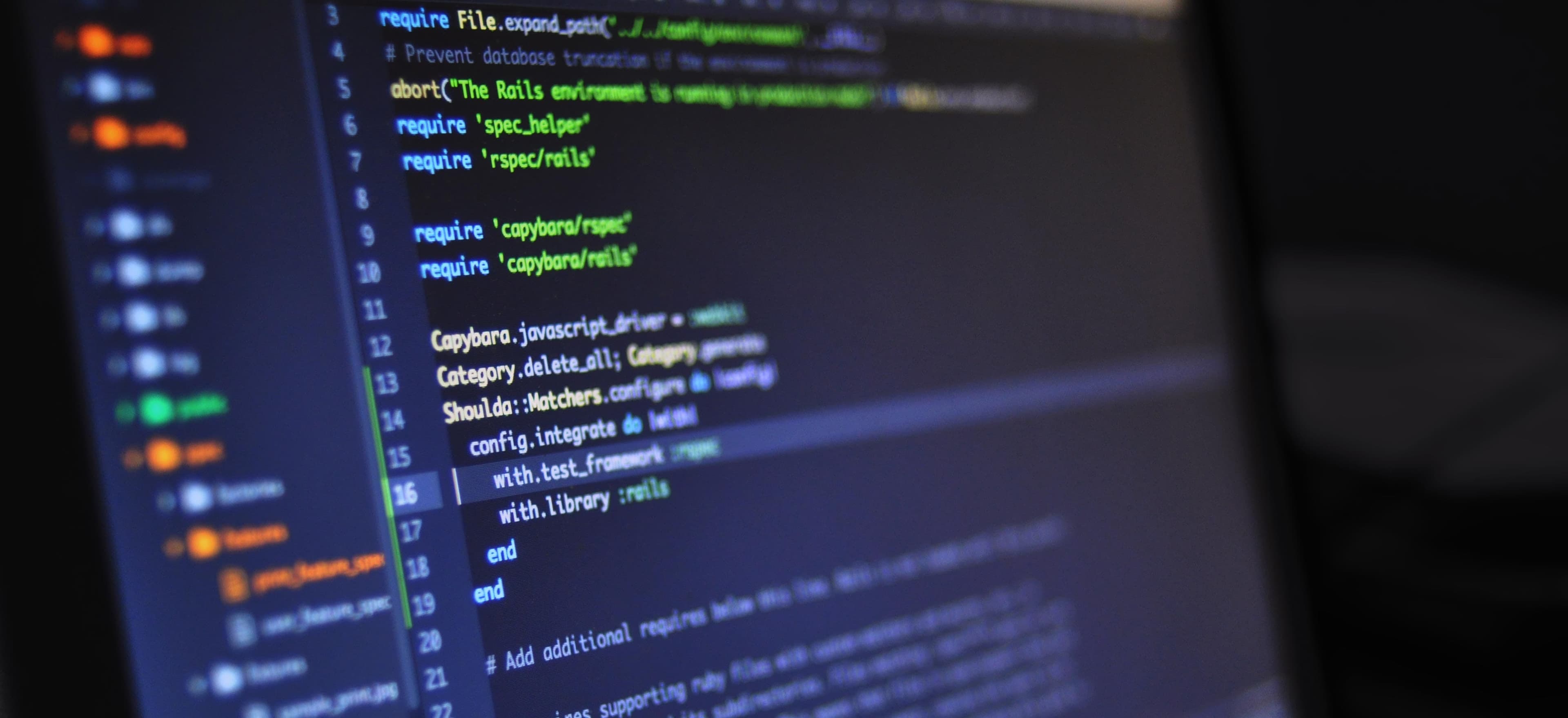
- Published on
Formatting Numbers in Java: A Complete Guide
When dealing with numbers in Java, it's essential to understand how to format them properly for display and manipulation. Properly formatted numbers not only enhance the user experience but also improve the readability of the code. In this comprehensive guide, we'll cover various techniques and libraries for formatting numbers in Java, including formatting integers, decimals, currencies, and percentages.
Formatting Integers
Java provides the DecimalFormat
class in the java.text
package, which allows us to format integers and decimals. Let's see an example of formatting an integer using DecimalFormat
:
import java.text.DecimalFormat;
public class IntegerFormattingExample {
public static void main(String[] args) {
int number = 1000000;
DecimalFormat decimalFormat = new DecimalFormat("#,###");
String formattedNumber = decimalFormat.format(number);
System.out.println("Formatted Number: " + formattedNumber);
}
}
In this example, the #
and ,
symbols in the pattern #
in the DecimalFormat
specify the thousands separator. The output of the code would be: Formatted Number: 1,000,000
.
Formatting Decimals
Formatting decimals is similar to formatting integers, but it requires handling the decimal part as well. Let's see an example of formatting a decimal number using DecimalFormat
:
import java.text.DecimalFormat;
public class DecimalFormattingExample {
public static void main(String[] args) {
double number = 12345.6789;
DecimalFormat decimalFormat = new DecimalFormat("#,###.##");
String formattedNumber = decimalFormat.format(number);
System.out.println("Formatted Number: " + formattedNumber);
}
}
In this example, the pattern #,###.##
in the DecimalFormat
specifies the thousands separator and the number of decimal places. The output of the code would be: Formatted Number: 12,345.68
.
Formatting Currencies
When dealing with currencies, it's important to format the numbers according to the currency standards. Java provides the NumberFormat
class for formatting currencies. Let's see an example of formatting a currency in Java:
import java.text.NumberFormat;
import java.util.Locale;
public class CurrencyFormattingExample {
public static void main(String[] args) {
double amount = 12345.67;
NumberFormat currencyFormat = NumberFormat.getCurrencyInstance(Locale.US);
String formattedCurrency = currencyFormat.format(amount);
System.out.println("Formatted Currency: " + formattedCurrency);
}
}
In this example, we use the NumberFormat.getCurrencyInstance(Locale.US)
to get the currency format for the US locale. The output of the code would be: Formatted Currency: $12,345.67
.
Formatting Percentages
Formatting percentages is another common requirement when working with numbers. Java provides the NumberFormat
class for formatting percentages. Let's see an example of formatting a percentage in Java:
import java.text.NumberFormat;
public class PercentageFormattingExample {
public static void main(String[] args) {
double percentage = 0.75;
NumberFormat percentageFormat = NumberFormat.getPercentInstance();
String formattedPercentage = percentageFormat.format(percentage);
System.out.println("Formatted Percentage: " + formattedPercentage);
}
}
In this example, we use the NumberFormat.getPercentInstance()
to get the percentage format. The output of the code would be: Formatted Percentage: 75%
.
More Advanced Formatting
For more advanced formatting requirements, such as controlling the rounding mode, handling different locales, or customizing the format symbols, libraries like Apache Commons Lang and Guava can be extremely helpful. These libraries offer additional formatting options and utilities to handle various number formatting scenarios.
Lessons Learned
Properly formatting numbers is an essential aspect of Java programming, whether it's for displaying data to users or processing numerical values. Understanding how to format integers, decimals, currencies, and percentages using Java's built-in classes and libraries empowers developers to present data in a clear, readable manner while adhering to specific formatting standards. By leveraging the techniques and libraries discussed in this guide, developers can ensure that the numbers in their Java applications are presented accurately and effectively.