Optimizing Java Garbage Collection for HotSpot
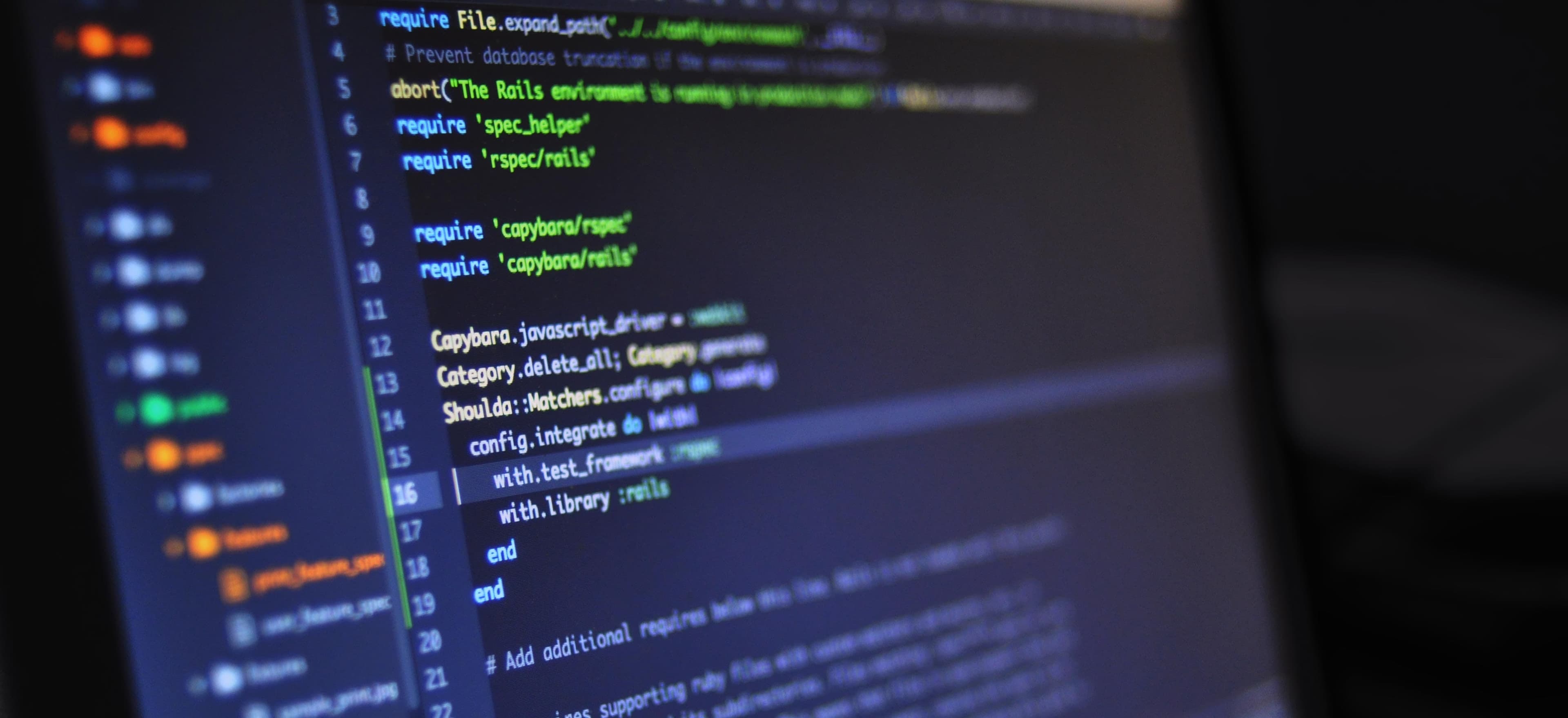
- Published on
Understanding Java Garbage Collection
In the world of Java programming, memory management is crucial. When coding in Java, there is no need to explicitly allocate and deallocate memory as Java has a built-in automatic garbage collection mechanism. However, as with any automated process, there are ways to optimize and fine-tune the garbage collection to increase the performance of your Java applications.
HotSpot Garbage Collector
The HotSpot JVM is one of the most widely used Java Virtual Machines, and it comes with several garbage collection algorithms. Understanding these algorithms and how to optimize them can significantly impact the performance of your Java applications.
Types of HotSpot Garbage Collectors
HotSpot comes with several garbage collectors, such as:
- Serial Garbage Collector: Suitable for small applications and simple client applications.
- Parallel Garbage Collector: Utilizes multiple threads for young generation collection and a single thread for old generation collection. It is designed for throughput optimization.
- Concurrent Mark-Sweep (CMS) Garbage Collector: Also known as the concurrent collector, it minimizes the pauses due to garbage collection by doing most of the work concurrently with the application threads.
- Garbage-First (G1) Garbage Collector: Aimed at providing high throughput and low-latency garbage collection for applications running on multi-processor machines with large memories.
Optimizing HotSpot Garbage Collection
1. Choose the Right Garbage Collector
Selecting the appropriate garbage collector depends on the nature of your application. For instance, if your application requires low latency and handles a large heap, the G1 collector might be the best choice. On the other hand, if throughput is a higher priority, the Parallel Garbage Collector may be the better option.
// Example: Setting the G1 garbage collector
java -XX:+UseG1GC YourMainClass
2. Analyze Garbage Collection Logs
Analyzing garbage collection logs can provide valuable insights into the behavior of the garbage collector and help identify potential optimization opportunities. Tools like jstat
, jcmd
, and jvisualvm
can be used to generate and analyze garbage collection logs.
3. Adjust Heap Sizing
Optimizing the heap size is critical for efficient garbage collection. Setting the heap size too small can lead to frequent garbage collection cycles, while setting it too large can result in longer pause times.
// Example: Setting initial and maximum heap size
java -Xms512m -Xmx2048m YourMainClass
4. Minimize Object Creation
Frequent object creation can burden the garbage collector. Reusing objects or using object pooling can help reduce the pressure on the garbage collector.
5. Utilize Garbage Collection Profilers
Tools like Java Mission Control and VisualVM provide detailed insights into garbage collection behavior, allowing you to identify potential bottlenecks and areas for improvement.
My Closing Thoughts on the Matter
In conclusion, optimizing Java garbage collection for HotSpot can significantly enhance the performance of your Java applications. By selecting the right garbage collector, analyzing logs, adjusting heap sizing, minimizing object creation, and utilizing profilers, you can fine-tune the garbage collection process to meet the specific requirements of your application.
For further reading on HotSpot garbage collection optimization, I recommend the following resources:
- Oracle's Garbage Collection Tuning Guide
- Understanding Java Garbage Collection
By implementing these best practices, you can ensure that your Java applications run efficiently and perform optimally in a variety of runtime environments.
Checkout our other articles