Managing Jenkins Pipeline Workflows with Docker and Ansible
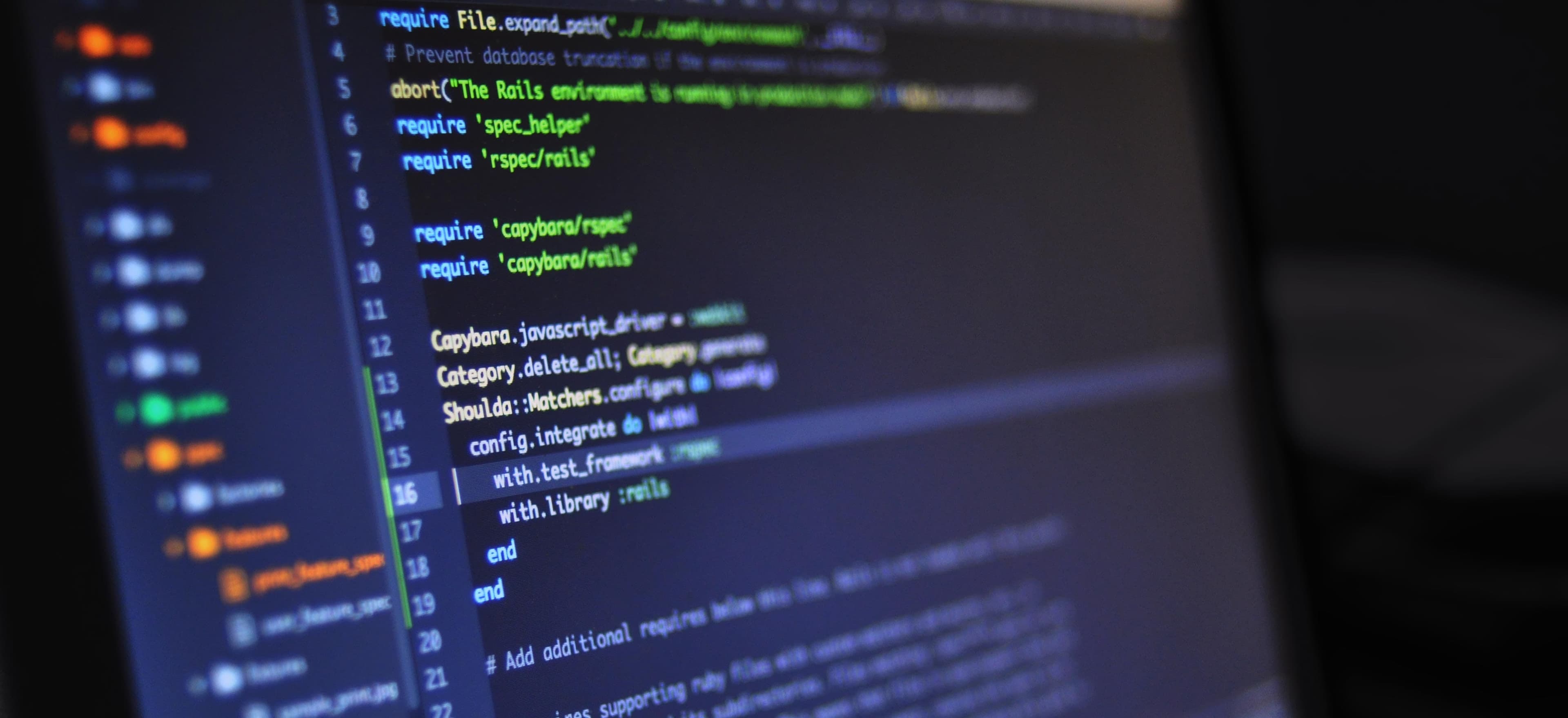
- Published on
Managing Jenkins Pipeline Workflows with Docker and Ansible
Jenkins is a popular automation server that allows you to automate various aspects of software development. Jenkins pipelines enable you to define the entire build, test, and deployment process as code, making it easier to manage, version, and reproduce your pipeline configuration.
In this blog post, we'll explore how to manage Jenkins pipeline workflows using Docker and Ansible. Docker allows us to containerize our build environment, ensuring consistency across different environments, while Ansible helps with the configuration management and orchestration of our infrastructure.
Setting Up Docker for Jenkins Pipeline
First, we need to set up Jenkins to use Docker as the build environment for our pipeline. We can do this by installing the Docker plugin in Jenkins. This plugin allows Jenkins to dynamically provision Docker containers as build slaves and run our build inside those containers.
pipeline {
agent {
docker {
image 'maven:3.5.2-jdk-8'
}
}
stages {
stage('Build') {
steps {
sh 'mvn clean package'
}
}
stage('Test') {
steps {
sh 'mvn test'
}
}
stage('Deploy') {
steps {
sh 'ansible-playbook deploy.yaml'
}
}
}
}
In this pipeline configuration, we use the Docker agent to specify the Docker image to be used as the build environment. In this case, we're using the Maven 3.5.2 with JDK 8 image. This ensures that our build environment is consistent and reproducible across different Jenkins agents.
Using Ansible for Configuration Management
Once we have our build artifacts, we might need to deploy them to various environments. This is where Ansible comes in. Ansible allows us to define our infrastructure as code and automate the deployment and configuration management processes.
- name: Deploy application
hosts: all
tasks:
- name: Copy artifact
copy:
src: /path/to/artifact
dest: /opt/myapp
- name: Restart application
systemd:
name: myapp
state: restarted
In this example Ansible playbook, we define tasks to copy the build artifact to the target servers and restart the application. We can easily integrate this Ansible playbook into our Jenkins pipeline to automate the deployment process.
Integrating Docker and Ansible into Jenkins Pipeline
Now that we have our Dockerized build environment and our Ansible playbooks for deployment, let's integrate these into our Jenkins pipeline. Below is an example Jenkinsfile that demonstrates how to use Docker for the build environment and Ansible for deployment.
pipeline {
agent {
docker {
image 'maven:3.5.2-jdk-8'
}
}
stages {
stage('Build') {
steps {
sh 'mvn clean package'
}
}
stage('Test') {
steps {
sh 'mvn test'
}
}
stage('Deploy') {
steps {
ansiblePlaybook(
playbook: '/path/to/deploy.yaml',
inventory: '/path/to/inventory'
)
}
}
}
}
In this Jenkinsfile, we use the Docker agent for the build stages and then use the ansiblePlaybook
step to run our Ansible playbook for deployment. This allows us to manage the entire CI/CD process as code, ensuring consistency and reproducibility across different environments.
Benefits of Using Docker and Ansible with Jenkins Pipeline
Consistent Build Environments
By using Docker for the build environment, we ensure that our build process is consistent across different environments. This eliminates the "but it works on my machine" problem and makes it easier to reproduce builds.
Infrastructure as Code
With Ansible, we can define our infrastructure and deployment processes as code. This makes it easier to manage and version our infrastructure and ensures that our deployments are consistent and repeatable.
Simplified Jenkins Configuration
Using Docker and Ansible with Jenkins pipelines simplifies the Jenkins configuration. We don't need to manually configure build agents or manage complex deployment scripts within Jenkins. Everything is defined as code and can be version-controlled.
Faster Feedback Loops
By automating the build, test, and deployment processes, we can shorten the feedback loops and deliver software faster. Docker and Ansible help in streamlining these processes, allowing for faster iterations and quicker delivery of features.
Final Thoughts
In this blog post, we discussed the use of Docker and Ansible in managing Jenkins pipeline workflows. We explored how Docker provides a consistent and reproducible build environment, while Ansible helps with the configuration management and orchestration of infrastructure.
Integrating Docker and Ansible into Jenkins pipelines simplifies the management of the CI/CD process, ensures consistency across different environments, and enables faster delivery of software. By adopting these tools, teams can streamline their development workflows and focus on delivering high-quality software efficiently.
We hope this blog post has provided valuable insights into managing Jenkins pipeline workflows with Docker and Ansible. If you have any thoughts or questions, feel free to leave a comment below!
For further reading, you can refer to the official documentation for Jenkins Pipeline and Docker, as well as the official guides for Ansible.
Checkout our other articles