Overcoming Writer's Block: Making the Most of Limited Time
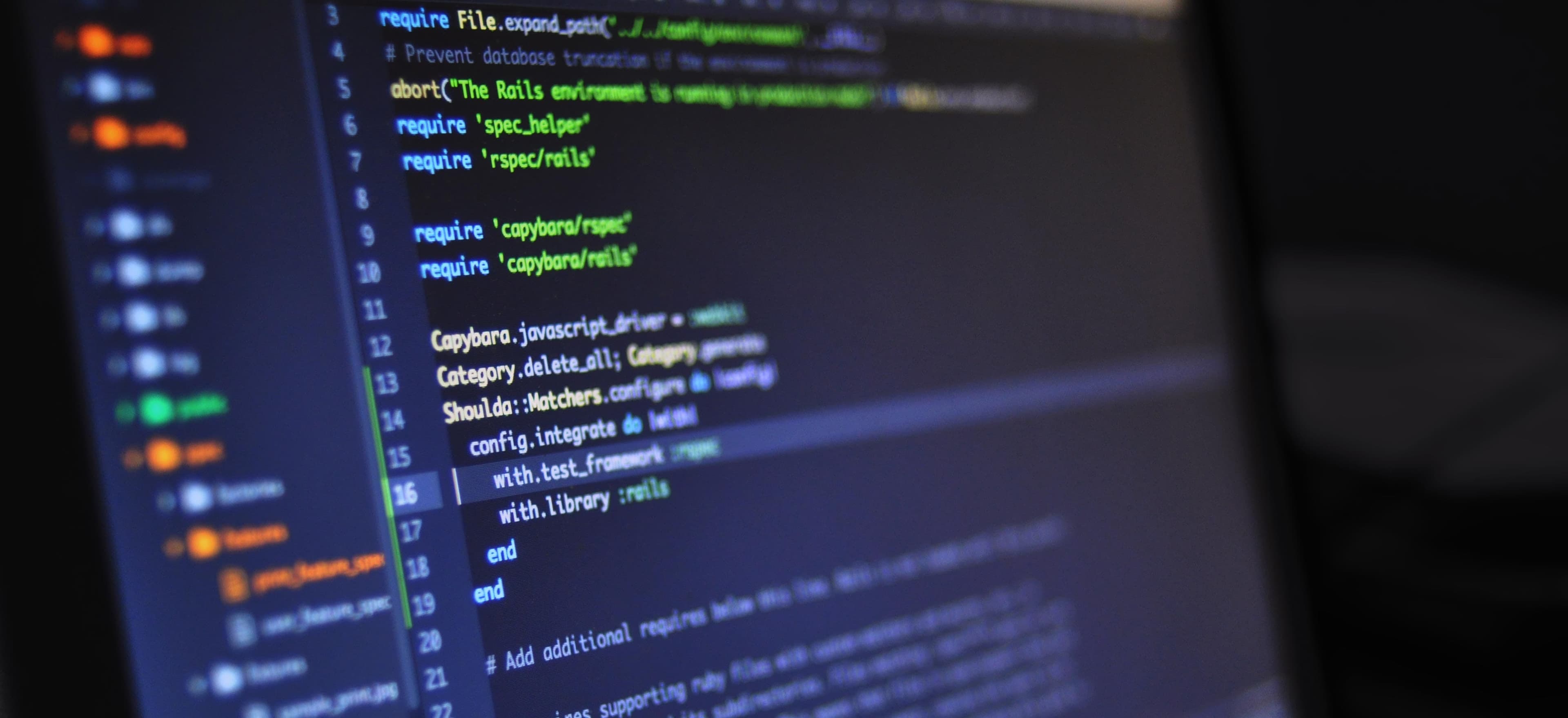
- Published on
Unlocking Your Potential: Overcoming Writer's Block with Java
Writing code is like crafting a story. The task is to create a masterpiece that not only works flawlessly but is also easy to follow and maintain. Unfortunately, just like writers, developers can experience moments of frustration and procrastination, commonly known as "writer's block". In this blog post, we will explore some strategies for overcoming writer's block and making the most of limited time when working in Java.
Understanding Writer's Block
Writer's block can strike at any time, leaving even the most experienced coders feeling creatively drained. It often manifests as a lack of inspiration, motivation, or simply an inability to move forward with a programming task. This state of mind can hinder productivity and lead to missed deadlines.
The Power of Pseudocode
When facing writer's block, one effective strategy is to start with pseudocode. Pseudocode is a high-level description of a computer program or algorithm, often used for planning and as a preliminary step in the development process. By breaking down the problem into smaller, manageable steps, you can gradually build the momentum needed to dive into actual code implementation.
// Example of Pseudocode for a Simple Java Program
START
Set up the necessary variables
Get user input
Perform calculations
Display the results
END
Embracing Agile Methodologies
In the world of software development, Agile methodologies such as Scrum and Kanban promote iterative and incremental work cycles. Leveraging Agile practices can help developers combat writer's block by breaking down larger tasks into smaller, more achievable goals. This approach not only maintains a sense of progress but also allows for regular reflections and adaptations to the original plan.
Leveraging Design Patterns
Design patterns offer proven solutions to recurring design problems in software development. When faced with writer's block, exploring various design patterns can inspire new ideas and approaches to problem-solving. For example, the Observer pattern can help in designing event-driven systems, while the Strategy pattern can assist in dynamically selecting an algorithm at runtime.
// Example of the Observer Design Pattern in Java
public interface Observer {
void update(String message);
}
public class ConcreteObserver implements Observer {
@Override
public void update(String message) {
System.out.println("Received message: " + message);
}
}
public class Subject {
private List<Observer> observers = new ArrayList<>();
public void addObserver(Observer observer) {
observers.add(observer);
}
public void notifyObservers(String message) {
for (Observer observer : observers) {
observer.update(message);
}
}
}
Leveraging Code Reviews and Pair Programming
Seeking feedback from peers through code reviews and engaging in pair programming can break the cycle of writer's block. These practices foster collaboration and provide fresh perspectives that inspire new ideas or solutions. Hearing diverse viewpoints can often spark creativity and revive motivation during challenging times.
Utilizing Java Libraries and Frameworks
Java boasts a rich ecosystem of libraries and frameworks that can aid developers in overcoming writer's block. Leveraging popular libraries like Apache Commons Lang for utility functions or Guava for collections and caching can eliminate the need to reinvent the wheel and jump-start the coding process. Similarly, frameworks like Spring and Hibernate offer robust solutions for building enterprise-level applications, unblocking developers and allowing them to focus on business logic.
My Closing Thoughts on the Matter
Writer's block is a common phenomenon that can impede software development. However, by understanding its nature and adopting strategies to combat it, developers can minimize its impact and continue to produce high-quality code. Whether through utilizing pseudocode, embracing Agile methodologies, leveraging design patterns, seeking feedback, or making use of libraries and frameworks, there are various approaches to regain momentum and overcome writer's block in Java programming.
In conclusion, facing writer's block is not a sign of weakness, but rather an opportunity to explore alternative methods and grow as a developer. By implementing the strategies discussed in this post, you can transform moments of frustration into opportunities for growth and innovation in your Java coding journey.
So, the next time you find yourself stuck, remember that writer's block is just another challenge waiting to be conquered with the right tools and mindset.
Remember that overcoming writer’s block is not only about technical solutions but also about maintaining a positive and constructive mindset. Taking care of your mental health is essential for long-term success as a developer.
Keep coding, keep growing, and keep thriving!