Document Structure Evolution in Morphia and MongoDB
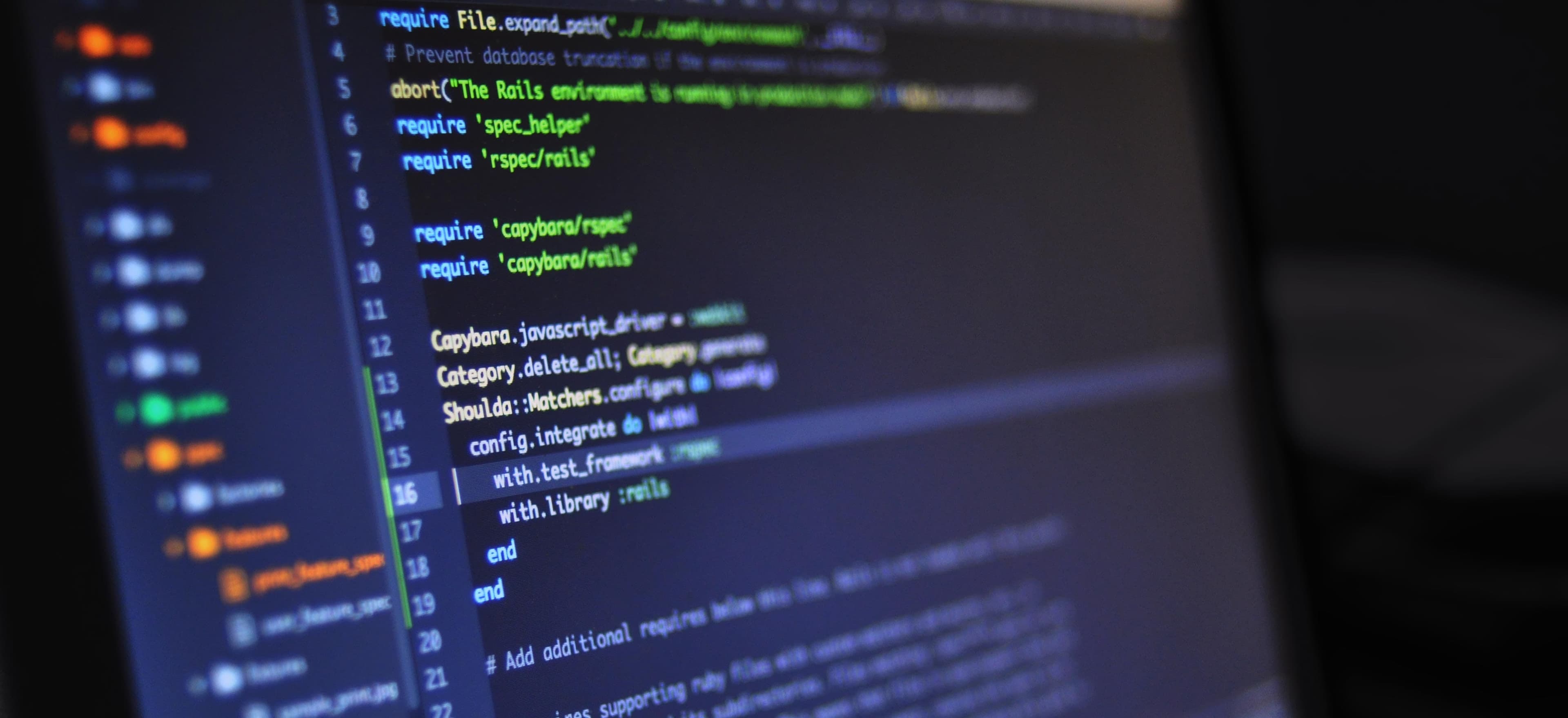
- Published on
Evolution of Morphia and MongoDB Document Structure
As Java developers, we are often faced with the challenge of designing and evolving document structures while working with NoSQL databases such as MongoDB. One of the key Java libraries for interacting with MongoDB is Morphia, which provides a powerful way to map Java objects to and from MongoDB documents. In this article, we will explore the evolution of document structure in Morphia and MongoDB, discussing best practices and techniques for handling changes in document structures over time.
Understanding Document Structures in MongoDB
MongoDB is a document-oriented NoSQL database, which means data is stored in flexible, JSON-like documents. In MongoDB, a collection is a group of documents, and each document is a set of key-value pairs. This flexible schema allows for easy evolution of document structures over time, making it ideal for agile development and rapid iteration.
Mapping Java Objects to MongoDB Documents with Morphia
Morphia is an object-document mapping library for MongoDB and Java that allows us to work with MongoDB in a type-safe and intuitive manner. Using Morphia, we can define Java classes that map to MongoDB documents, and Morphia takes care of the object-document mapping for us.
Defining Document Structures in Morphia
@Entity("employees")
public class Employee {
@Id
private ObjectId id;
private String name;
private int age;
private List<String> skills;
// getters and setters
}
In the above example, we have defined a simple Employee
class that maps to a MongoDB collection called "employees". The @Entity
annotation marks the class as a document entity, and the @Id
annotation indicates the primary key field. The other fields in the class correspond to the key-value pairs in the MongoDB document.
Evolving Document Structures Over Time
In real-world applications, document structures often need to evolve to accommodate new requirements, features, or bug fixes. This evolution may involve adding new fields, removing existing fields, or restructuring the document altogether. Let's explore some best practices and techniques for handling these changes in Morphia and MongoDB.
Adding a New Field to an Existing Document
Adding a new field to an existing document is a common scenario when evolving document structures. In Morphia, we can simply add a new field to the Java class and annotate it with @Property
.
public class Employee {
// existing fields...
@Property
private String department;
// getters and setters
}
By annotating the new field with @Property,
Morphia will map this field to the corresponding key in the MongoDB document. Existing documents will be automatically updated to include the new field when they are loaded into the Java application.
Removing a Field from an Existing Document
Removing a field from an existing document requires careful consideration, especially if the field contains data that needs to be migrated or handled differently. In Morphia, we can simply remove the field from the Java class, and Morphia will handle the removal of the field when documents are updated.
public class Employee {
// existing fields excluding 'age'
private String name;
// other fields...
}
Morphia will automatically exclude the 'age' field from the MongoDB document when documents are saved or updated using the updated Employee
class.
Restructuring Document Structures
In some cases, we may need to restructure the entire document to better represent the domain model or improve query performance. Morphia allows us to refactor the document structure by modifying the Java class and using query projections to handle changes in document structure without affecting existing data.
@Entity("employees")
public class Employee {
@Id
private ObjectId id;
private PersonalInfo personalInfo;
// other fields...
}
public class PersonalInfo {
private String name;
private int age;
// other fields...
}
In the above example, we have restructured the Employee
document to have a nested PersonalInfo
object. Morphia seamlessly handles this restructuring, and existing documents will be automatically updated to match the new structure when loaded into the application.
Data Migration and Versioning
When evolving document structures, it's essential to consider data migration and versioning to ensure backward compatibility and smooth transitions. Morphia does not provide built-in support for data migration or versioning, so it's important to implement these concerns as part of the application's data access layer.
The Last Word
In this article, we have explored the evolution of document structures in Morphia and MongoDB. We have discussed best practices for adding, removing, and restructuring document fields, as well as considerations for data migration and versioning. By understanding how to evolve document structures effectively, Java developers can ensure that their applications remain flexible, maintainable, and efficient when working with Morphia and MongoDB.
For further reading and in-depth exploration of Morphia and MongoDB, you can refer to the official Morphia documentation and the MongoDB manual. These resources provide comprehensive insights into using Morphia and MongoDB effectively in Java applications.
Remember, document structure evolution is a crucial aspect of maintaining a healthy and scalable database design. With Morphia and MongoDB, Java developers can embrace changes in document structures with confidence and agility. Happy coding!