Optimizing Loops with Local Variables
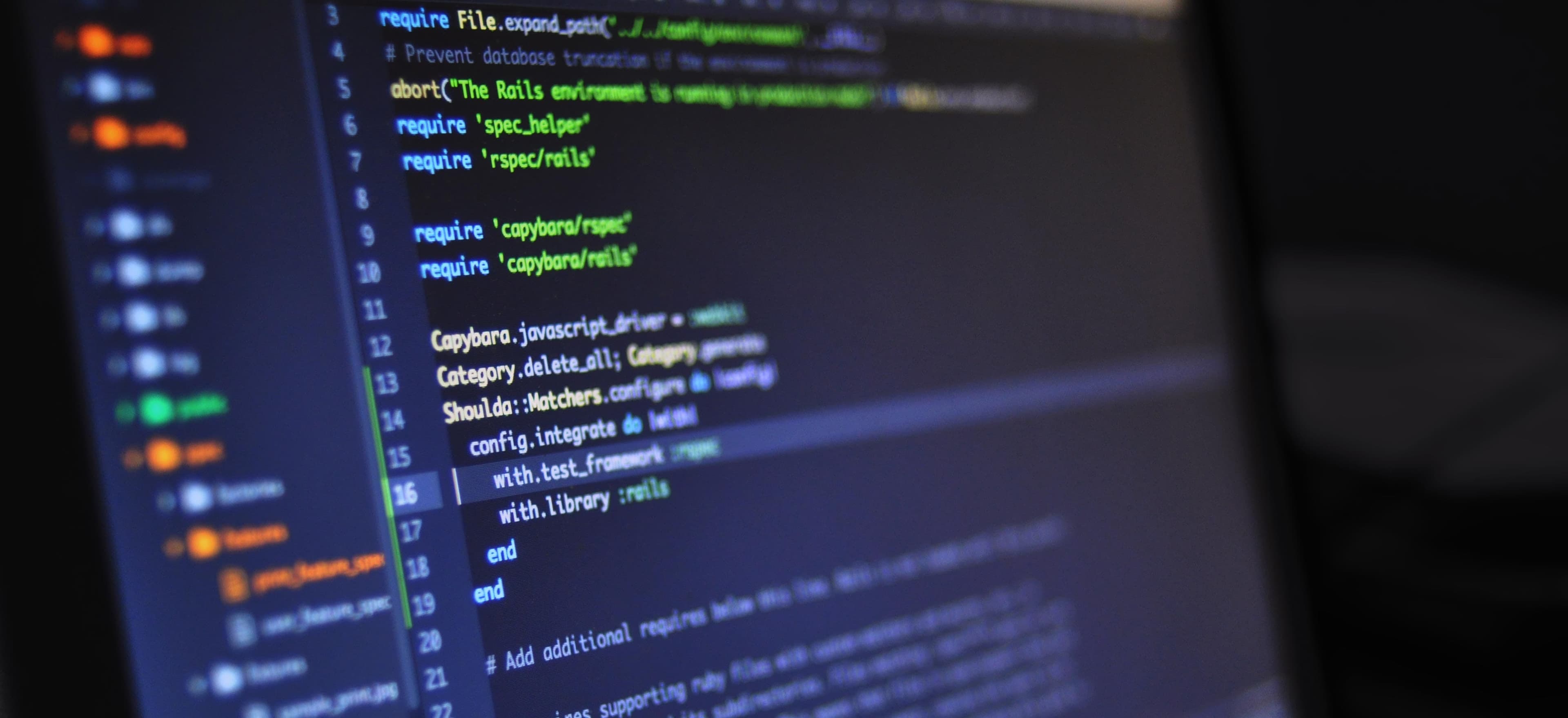
- Published on
Optimizing Loops with Local Variables in Java
When writing efficient and optimized Java code, it's crucial to pay attention to various aspects of your code, including loops. Loops are an essential part of programming, but they can also be a source of inefficiency if not implemented carefully. In this article, we'll explore how to optimize loops using local variables in Java.
The Importance of Optimizing Loops
Loops are used to perform repetitive tasks, such as iterating over arrays, lists, or performing a series of operations. Inefficiencies in loop implementations can lead to performance bottlenecks, especially when dealing with large datasets or complex algorithms. By optimizing loops, you can improve the overall performance of your Java application.
Using Local Variables to Improve Loop Performance
Local variables are variables that are declared within a method, constructor, or block. They are only accessible within the scope in which they are defined. When used effectively within loops, local variables can significantly improve performance by reducing the overhead of repeated method calls or memory accesses.
Consider the following example:
public void processList(List<String> items) {
int size = items.size(); // Store the size in a local variable
for (int i = 0; i < size; i++) {
String item = items.get(i); // Retrieve the item using the local variable
// Process the item
}
}
In this example, we store the size of the list in a local variable size
, which eliminates the need to call items.size()
within each iteration of the loop. This can lead to a performance improvement, especially for large lists, as it reduces the method call overhead.
Avoiding Redundant Method Calls
When iterating over collections or arrays within a loop, it's essential to minimize redundant method calls by storing the result of the method call in a local variable. For example:
public void processArray(int[] numbers) {
int length = numbers.length; // Store the array length in a local variable
for (int i = 0; i < length; i++) {
// Process the array element at index i
}
}
In this example, storing the length of the array in the local variable length
avoids calling numbers.length
repeatedly within the loop, which can improve performance, especially for large arrays.
Minimizing Object Instantiation Within Loops
Creating objects within loops can also impact performance, especially for complex objects or in situations where object creation is not necessary in each iteration. By creating the object outside the loop and reusing it within the loop, you can improve performance.
Consider the following example:
public void processList(List<String> items) {
StringBuilder sb = new StringBuilder(); // Create StringBuilder outside the loop
for (String item : items) {
sb.append(item); // Append to the StringBuilder within the loop
}
String result = sb.toString(); // Get the final result after the loop
// Process the result
}
In this example, we create a StringBuilder
outside the loop and reuse it to construct the final result within the loop. This approach minimizes unnecessary object creation within the loop, leading to improved performance.
Final Thoughts
Optimizing loops using local variables in Java can have a significant impact on the performance of your code. By minimizing redundant method calls, storing intermediate results in local variables, and minimizing object instantiation within loops, you can improve the efficiency of your Java applications. Remember to profile your code and measure the performance improvements achieved through loop optimizations.
In conclusion, optimizing loops using local variables is a simple yet effective technique for enhancing the performance of your Java code.
To delve deeper into the performance optimization of Java code, check out Java Performance: The Definitive Guide and Effective Java. These resources provide valuable insights into writing high-performance Java code.
Start applying these loop optimization techniques to your Java code and witness the performance improvements first-hand!