The Pitfalls of Java Object Interning
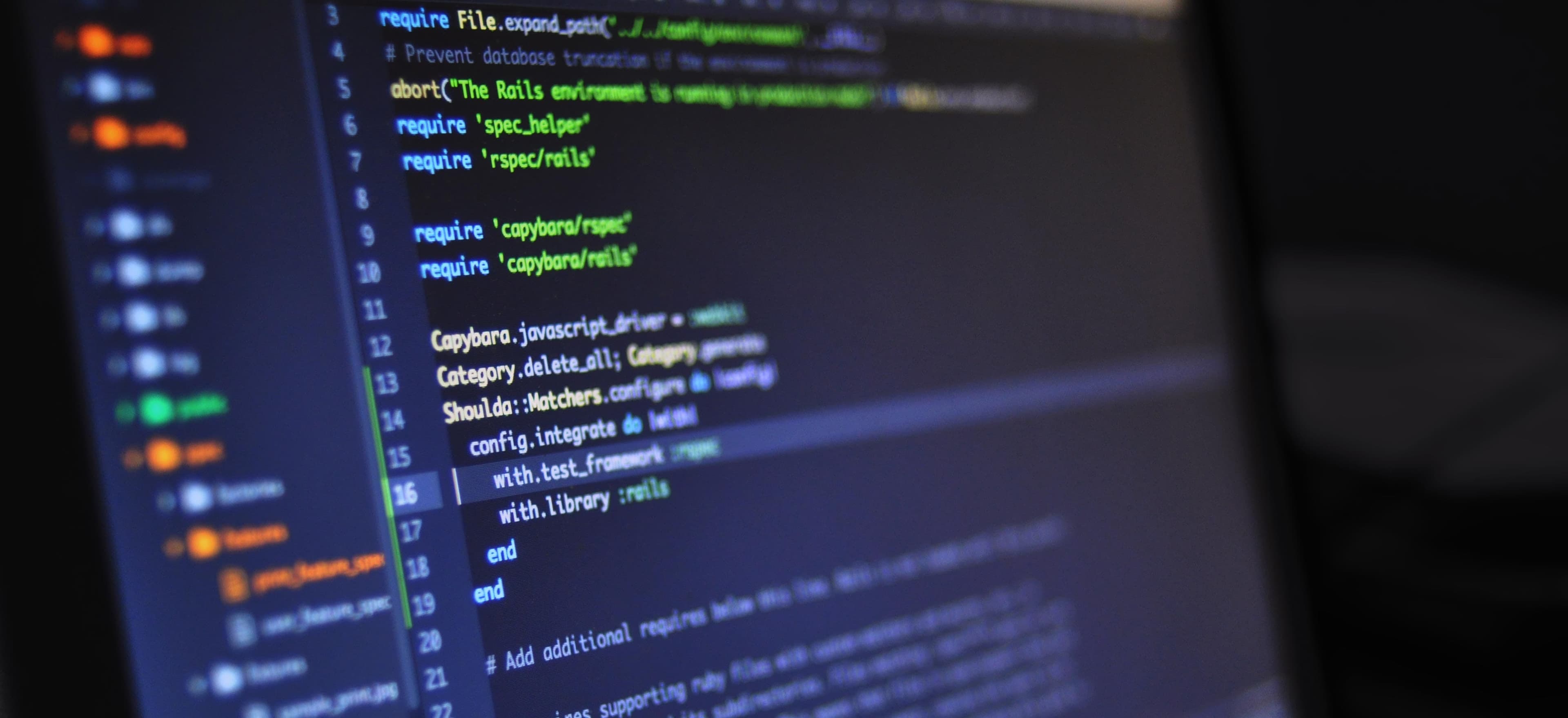
- Published on
The Pitfalls of Java Object Interning
When working with Java, developers often encounter the concept of object interning. While it can offer benefits such as memory conservation and potentially faster comparisons, it also comes with its own set of pitfalls that can lead to unexpected behavior and bugs in your code.
What is Object Interning?
Object interning in Java refers to the process of storing only one copy of each distinct value, which allows for the reusability of the existing objects instead of creating new ones. This is typically achieved through the use of the intern()
method, available on certain types like strings.
The Pitfalls
1. Memory Overhead
While object interning can save memory by reusing existing objects, it can also lead to increased memory overhead. This is because interned objects are stored in a separate area of the heap, known as the "permanent generation" (or "metaspace" in Java 8+). If not managed carefully, heavy use of interning can lead to increased memory usage and potential OutOfMemoryErrors.
2. Impact on Performance
Although interning can theoretically lead to faster comparisons when using ==
, it comes with a cost. The process of interning itself has a performance overhead, and the constant pool lookup required for interned strings can degrade performance, especially as the pool grows larger.
3. Thread Safety
The intern()
method is not thread-safe. In a multi-threaded environment, calling intern()
concurrently on the same string can lead to multiple copies of the string being interned, which can break assumptions in your code and lead to unexpected behavior.
4. Potential for Unintended Consequences
When interning objects, it's crucial to understand the impact of this process on the rest of your codebase. For instance, modifying an interned object can have unintended consequences elsewhere in the code, as the changes would be reflected across all references to the interned object.
5. PermGen/Metaspace Exhaustion
In pre-Java 8 versions, excessive interning of strings can lead to PermGen space exhaustion, especially when dealing with large, dynamically generated sets of strings. In Java 8 and later versions, where the permanent generation has been replaced by metaspace, a similar issue can occur, leading to metaspace exhaustion.
Best Practices
To mitigate the pitfalls of object interning, consider the following best practices:
-
Profile Your Application: Before considering extensive use of interning, profile your application to understand the actual memory and performance bottlenecks. This will help you make informed decisions about where interning can be beneficial.
-
Use Caution with Large Data Sets: Be cautious when interning objects from large or dynamically generated data sets, as this can quickly lead to memory exhaustion.
-
Concurrency Control: If using
intern()
in a multi-threaded environment, ensure proper synchronization to avoid concurrent interning. -
Consider Alternatives: Evaluate whether object interning is truly the best solution for your use case. In some scenarios, alternative approaches such as using a
Set
orMap
for deduplication may be more effective and less error-prone. -
Upgrade to Java 8+: If you're facing metaspace exhaustion issues, consider upgrading to Java 8 or later versions, where metaspace has replaced the permanent generation and provides more flexible memory allocation.
Example Code
public class ObjectInterningExample {
public static void main(String[] args) {
String s1 = new String("hello");
String s2 = "hello";
String s3 = s1.intern();
System.out.println(s1 == s2); // Output: false
System.out.println(s2 == s3); // Output: true
}
}
In the above example, s1
and s2
are two different string objects, even though they contain the same value. When s1.intern()
is called, it returns the canonical representation of the string, which in this case is s2
. This demonstrates the concept of object interning and its impact on object equality.
Closing the Chapter
While object interning in Java can offer benefits in terms of memory optimization and potentially faster comparisons, it's crucial to be aware of the pitfalls associated with it. By understanding the potential memory overhead, performance impact, thread safety concerns, and unintended consequences, you can make informed decisions about when and how to use object interning in your Java applications. Remember to profile your application, use interning judiciously, and consider alternative approaches when necessary to ensure a robust and efficient codebase.