Implementing Custom Deserializer in Jackson: Overcoming Validation Issues
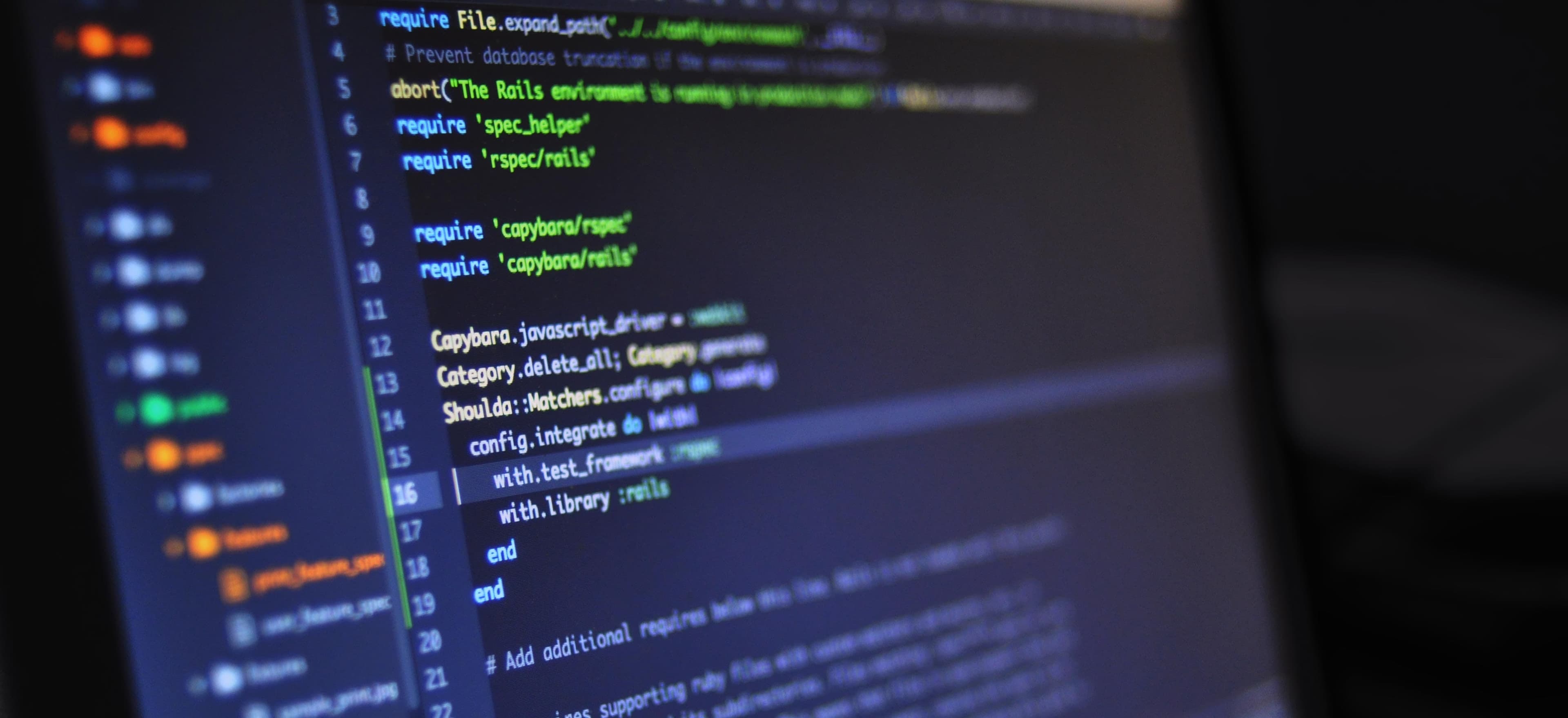
- Published on
Implementing Custom Deserializer in Jackson: Overcoming Validation Issues
In this blog post, we will delve into the world of custom deserialization using Jackson, a popular Java library for handling JSON. We'll explore how to create a custom deserializer to overcome validation issues that may arise during the process of deserializing JSON data.
Understanding Custom Deserialization
When working with JSON in Java, deserialization is the process of converting JSON data into Java objects. While Jackson provides default deserialization mechanisms, there are scenarios where custom deserialization logic is required, such as when the JSON structure does not align with the default behavior, or when additional validation needs to be performed during deserialization.
Discovering the Need for Custom Deserialization
Suppose you have a JSON representation of a Person
object containing a name
and age
field. However, the JSON may sometimes contain an invalid or missing age
field. In such cases, the default deserialization process may result in unexpected behavior or exceptions. To address this, we can create a custom deserializer to handle the validation logic during deserialization.
Let's explore how to implement a custom deserializer to validate and handle the Person
object's age
field.
Creating the Person Class
public class Person {
private String name;
private int age;
// Getters and setters omitted for brevity
}
Implementing the Custom Deserializer
First, we need to create a custom deserializer for the Person
class. This deserializer will be responsible for validating the age
field during deserialization.
public class PersonDeserializer extends JsonDeserializer<Person> {
@Override
public Person deserialize(JsonParser jsonParser, DeserializationContext deserializationContext)
throws IOException, JsonProcessingException {
ObjectCodec codec = jsonParser.getCodec();
JsonNode node = codec.readTree(jsonParser);
String name = node.get("name").asText();
int age = validateAndRetrieveAge(node);
return new Person(name, age);
}
private int validateAndRetrieveAge(JsonNode node) {
JsonNode ageNode = node.get("age");
if (ageNode == null || ageNode.isNull()) {
// Handle missing or null age field
throw new InvalidFormatException("Age field is missing or null", ageNode, int.class);
}
int ageValue = ageNode.asInt();
if (ageValue < 0) {
// Handle negative age
throw new InvalidFormatException("Age cannot be negative", ageNode, int.class);
}
return ageValue;
}
}
In the deserialize
method, we extract the name
and validate the age
field using the validateAndRetrieveAge
method. If the age
field is missing or null, or if it is a negative value, we throw a custom InvalidFormatException
. By creating a custom deserializer, we can enforce specific validation logic during deserialization.
Registering the Custom Deserializer
Now that we have the custom deserializer, we need to register it with the ObjectMapper
in order to use it during deserialization. This ensures that the custom deserializer is invoked when converting JSON to the Person
object.
ObjectMapper mapper = new ObjectMapper();
SimpleModule module = new SimpleModule();
module.addDeserializer(Person.class, new PersonDeserializer());
mapper.registerModule(module);
By registering the custom deserializer with the ObjectMapper
, we enable the validation logic to be applied during the deserialization process for the Person
class.
The Bottom Line
In this blog post, we discussed the need for custom deserialization and demonstrated how to implement a custom deserializer in Jackson to overcome validation issues during the deserialization process. By creating a custom deserializer for the Person
class, we were able to enforce specific validation logic for the age
field, ensuring that the deserialization process meets our requirements.
Custom deserialization in Jackson provides a powerful mechanism to tailor the deserialization process according to specific business needs. It empowers developers to handle complex JSON structures and apply custom validation while converting JSON data into Java objects.
By mastering the art of custom deserialization, developers can enhance the resilience and flexibility of their Java applications when dealing with diverse and sometimes unpredictable JSON data.
Now it's your turn to explore further and unleash the full potential of custom deserialization in Jackson! Happy coding!
For more information on custom deserialization and Jackson, refer to the official Jackson documentation.