Overcoming Challenges in End-to-End Testing
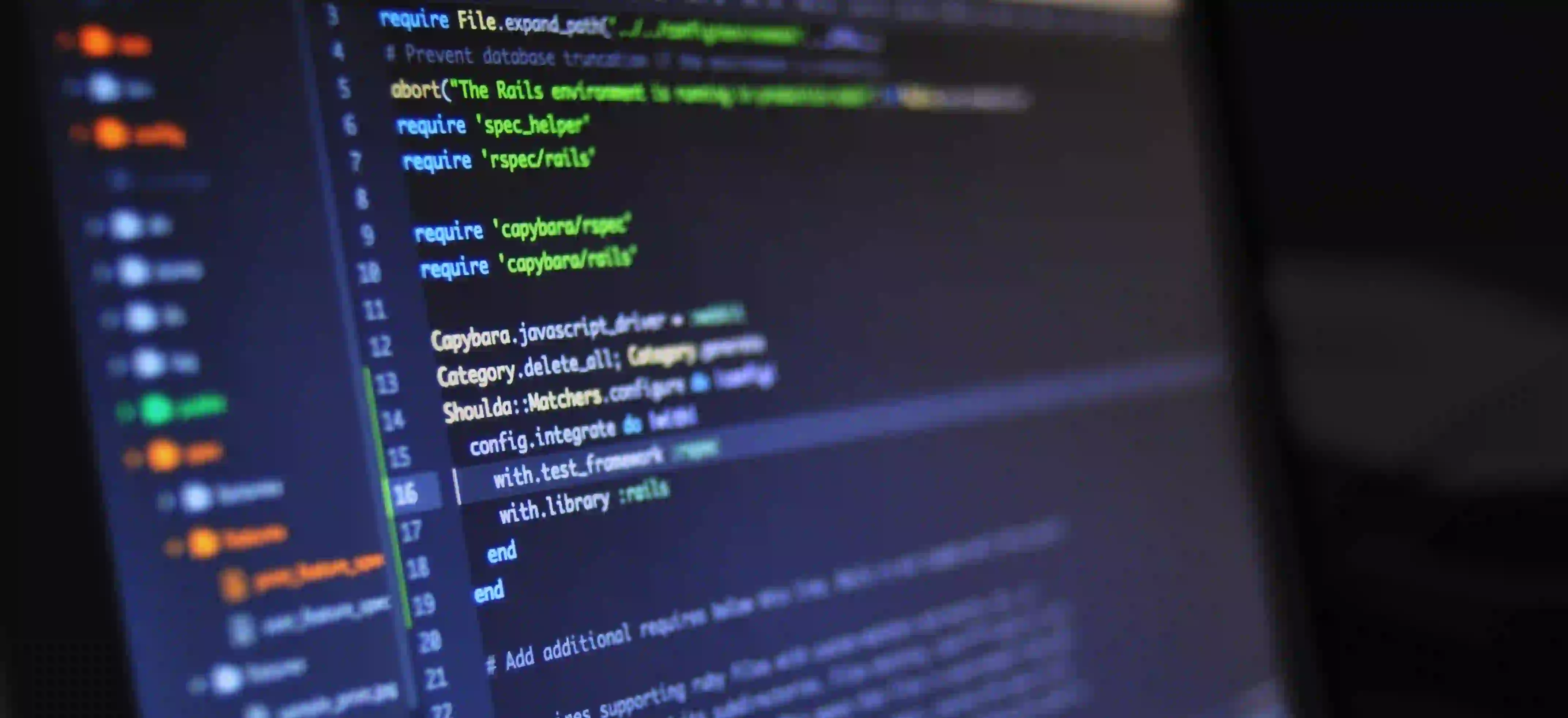
Overcoming Challenges in End-to-End Testing
End-to-end testing is a critical process in software development that ensures all the integrated components of an application work as expected. However, this type of testing comes with its own set of challenges. In this post, we will explore some of these challenges and discuss strategies to overcome them using Java.
Challenge 1: Setting Up Test Environments
Setting up test environments that mimic production can be quite challenging. This is because end-to-end testing requires the integration of multiple components such as databases, APIs, and UI.
Overcoming the Challenge
Using Docker for Environment Setup
With Docker, you can containerize each component of your application, making it easier to set up and tear down test environments consistently.
@Test
public void testEnvironmentSetup() {
// Use Docker Java API to set up containers for databases, APIs, etc.
// Your test code here
}
Using Docker Java API simplifies the process of orchestrating containers for your test environment.
Utilizing Mocking Frameworks
Mocking frameworks like Mockito can be used to simulate the behavior of external dependencies, such as APIs or databases, thus reducing the complexity of the test environment setup.
@Test
public void testSimulatedAPIBehavior() {
// Use Mockito to mock the behavior of an external API
// Your test code here
}
Mockito allows you to create mock objects to emulate the behavior of real objects.
Challenge 2: Handling Asynchronous Operations
In modern applications, asynchronous operations are commonplace. Testing these operations in an end-to-end scenario can be challenging.
Overcoming the Challenge
Leveraging CompletableFuture in Java
Using CompletableFuture
in Java can help in testing asynchronous operations, as it provides a way to perform operations asynchronously and then combine them when they are complete.
@Test
public void testAsynchronousOperation() {
CompletableFuture<String> futureResult = CompletableFuture.supplyAsync(() -> {
// Asynchronous operation here
return "result";
});
futureResult.thenAccept(result -> {
// Assertions on the result
}).join();
}
In this example, CompletableFuture
is used to test an asynchronous operation and perform assertions on the result when it's available.
Challenge 3: Data Setup and Cleanup
Managing test data for end-to-end testing can be complex, especially when dealing with databases and external services.
Overcoming the Challenge
Using Test Data Builders
Test data builders can be employed to create and manage test data effectively. Libraries such as builder
can help in building test data objects with fluent APIs.
@Test
public void testDataSetupAndCleanup() {
TestData testData = TestDataBuilder
.create()
.withName("John")
.withAge(25)
.build();
// Use the testData in your test scenario
}
The builder
library allows for the creation of test data objects with a clear and fluent syntax.
Employing Database Migration Tools
Tools like Flyway or Liquibase can be used to manage database schema and data changes during the testing process, ensuring that the test data is set up and cleaned up effectively.
@Test
public void testDatabaseMigration() {
// Use Flyway or Liquibase to migrate test database to a known state
// Your test code here
}
These tools assist in managing database changes and ensuring consistency in test data setup.
Challenge 4: Dealing with External Dependencies
End-to-end testing often involves interacting with external dependencies such as third-party APIs or services, which can be unreliable or slow.
Overcoming the Challenge
Using Service Virtualization
Tools like WireMock or Mountebank can be employed to create virtualized versions of third-party services, allowing for predictable and fast responses during testing.
@Test
public void testExternalServiceInteraction() {
// Use WireMock to stub the behavior of a third-party service
// Your test code here
}
Using WireMock to stub the behavior of a third-party service enables predictable and fast responses during testing.
With these strategies and tools, you can overcome the challenges associated with end-to-end testing in Java and ensure the robustness of your applications. Embracing best practices in testing not only enhances the quality of your code but also strengthens the overall reliability of your software.
In conclusion, addressing the challenges of setting up test environments, handling asynchronous operations, managing test data, and dealing with external dependencies empowers developers to write comprehensive end-to-end tests in Java. With the right strategies and tools in place, testing can become a seamless and integral part of the software development lifecycle.