Managing Memory: Dealing with Java's Garbage Collection
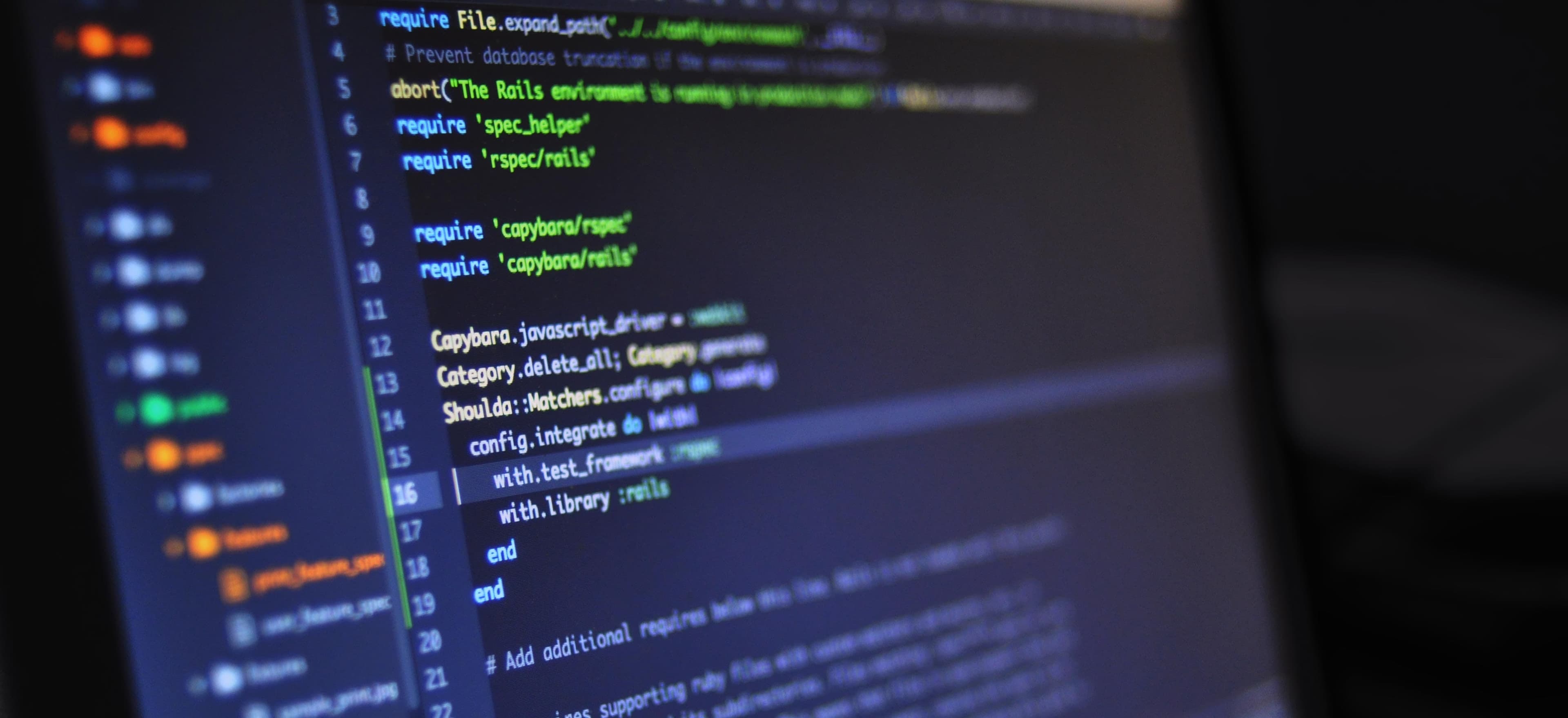
- Published on
Understanding Java Garbage Collection
Java's Garbage Collection (GC) is an automatic memory management process that relieves developers from manual memory deallocation. However, it's essential to have a good understanding of how it works, as improper memory management can lead to performance issues, such as memory leaks and high CPU usage.
How Garbage Collection Works in Java
When a Java program creates objects, the JVM allocates memory to them. Over time, some objects become unreachable because there are no references to them. The GC identifies these unreachable objects and reclaims their memory, making it available for future allocations.
Types of GC Algorithms
Java provides several GC algorithms to cater to different application scenarios. The most commonly used are:
- Serial Garbage Collector
- Parallel Garbage Collector
- CMS Garbage Collector
- G1 Garbage Collector
Each algorithm has its pros and cons, and the choice of algorithm depends on the application's memory usage and performance requirements.
Tips for Effective Memory Management in Java
1. Limit Object Creation
Excessive object creation can strain the GC as it increases the frequency of garbage collection cycles. Consider reusing objects or employing object pooling techniques to minimize unnecessary object creation.
String s1 = "Hello"; // creates a new object
String s2 = "Hello"; // reuses the existing object
Why? Minimizing object creation reduces memory churn and enhances overall performance.
2. Dispose of Resources Properly
It's crucial to release resources such as file handles, database connections, and network sockets when they are no longer needed. Failing to do so can lead to resource leaks and potentially affect the entire application's functionality.
try (FileReader fr = new FileReader("file.txt");
BufferedReader br = new BufferedReader(fr)) {
// Read from the file
} catch (IOException e) {
// Handle the exception
}
Why? Proper resource disposal ensures efficient memory usage and prevents resource exhaustion.
3. Tune Garbage Collection
Understanding the behavior of GC algorithms and tuning them based on the application's memory requirements is crucial for optimal performance. This can involve adjusting parameters such as heap size, generation sizes, and GC algorithm selection.
// Set JVM heap size
java -Xmx2G -Xms512M MyApp
Why? Tuning GC parameters can significantly impact memory utilization and overall application responsiveness.
4. Use Memory Profiling Tools
Utilize memory profiling tools like VisualVM or YourKit to identify memory hotspots, memory leaks, and inefficient memory usage. These tools provide insights into memory allocation patterns and help in optimizing memory usage.
// VisualVM memory profiler
jvisualvm
Why? Memory profiling tools offer valuable insights into memory allocation behavior and aid in resolving memory-related issues.
Best Practices for Garbage Collection
1. Minimize Object Finalization
Object finalization adds overhead to the garbage collection process. Minimize the usage of finalize()
method and prefer explicit resource cleanup methods such as close()
for better resource management.
public class ResourceManager {
public void close() {
// Clean up the resources
}
}
Why? Avoiding object finalization improves GC efficiency and reduces memory contention.
2. Utilize Weak References
For transient and non-essential references, consider using weak references to allow the GC to collect the objects when they are no longer strongly reachable.
WeakReference<Object> weakRef = new WeakReference<>(new Object());
Object obj = weakRef.get(); // Retrieve the object
Why? Weak references help in managing transient references and prevent unnecessary memory retention.
Bringing It All Together
Java's Garbage Collection automates memory management, but understanding its nuances is crucial for optimizing memory usage and overall application performance. By following best practices, utilizing memory profiling tools, and implementing effective memory management techniques, developers can ensure efficient memory utilization and smooth application functioning.
Remember, proficient memory management is not just a best practice; it's a necessity for building robust and responsive Java applications.
For further details on Java Garbage Collection, you can refer to the official Oracle documentation and Baeldung's comprehensive guide.